---------------------加油加油武汉加油中国加油!------------------------------------------------------------------------------------2020-02-04----------------------------------------
一.属性篇
1.placeholder
<input type = "text" placeholder = "手机/邮箱/用户名">
2.input新增的type
<!--Calender类-->
<input type = "date"><!--chrome支持,Safari,IE不支持 -->
<input type = "time">
<input type = "datetime-local">
<input type = "week">
<input type = "number"><!--只允许填数字,以上以下兼容性都不好-->
<input type = "email">
<input type = "color">
<input type = "range" min="1" max = "100" name = "range"><!--进度条-->
<input type = "search"><!--会自动提示以前填过的信息-->
<input type = "url">
3.contenteditable
//使字母wangjunkai可在屏幕中直接修改,可以继承给子元素,不要嵌套,没有兼容性问题
<div contenteditable = "true">wangjunkai</div>
4.Drag被拖拽元素
<div class = "k" draggable = "true"></div><!--chrome,safari可用-->
<!--<a>标签,<img src = " ">标签,默认携带拖拽功能。-->
<!--拖拽的生命周期
1.拖拽开始,拖拽进行中,拖拽结束。
2.被拖拽的物体,目标区域
-->
<!--按下物体的瞬间是不会触发事件的-->
<script>
//拖拽开始
var oDragDiv = document.getElementByClassName("a")[0];
oDragDiv.ondragstart = function(e){
console.log(e);
}
//拖拽中
oDragDiv.ongrag = function(e){
console.log(e);
}
//拖拽结束
oDragDiv.ondragend = function(e){
console.log(e);
}
</script>
//一个拖拽事件,拖拽移动正方形
<style>
.a{
width: 200px;
height: 200px;
position: absolute;
background-color: aqua;
}
</style>
<body>
<div class="a" draggable="true"></div>
</body>
<script>
var oDragDiv = document.getElementsByClassName("a")[0];
var beginX = 0;
var beginY = 0;
oDragDiv.ondragstart = function(e){
beginX = e.clientX;
beginY = e.clientY;
}
oDragDiv.ondragend = function(e){
var x = e.clientX-beginX;
var y = e.clientY-beginY;
oDragDiv.style.left = oDragDiv.offsetLeft+x+"px";
oDragDiv.style.right = oDragDiv.offsetRight+y+"px";
}
</script>
//拖拽到目标,目标元素的事件
<style>
.a{
width: 200px;
height: 200px;
position: absolute;
background-color: aqua;
}
.target{
position: absolute;
width: 300px;
height: 300px;
border: 1px solid;
left: 600px;
}
</style>
<body>
<div class="a" draggable="true"></div>
<div class="target"></div>
</body>
// 所有的标签元素当周期事件结束时,默认事件回到原处。
// 事件是由行为触发的,但是一个行为不止触发一个事件。
<script>
var oDragDiv = document.getElementsByClassName("a")[0];
oDragDiv.ondragstart = function(e){
}
oDragDiv.ondragend = function(e){
}
var oDragTarget = document.getElementsByClassName("target")[0];
oDragTarget.ondragenter = function(e){//不是元素图形进入就触发,是拖拽的鼠标进入才触发。
}
oDragTarget.ondragover = function(e){//在目标区域移动触发
e.preventDefault();
}
oDragTarget.ondragleave = function(e){//离开目标区域触发
}
oDragTarget.ondrop = function(e){//在ondragover中触发default,才能触发drop事件。
}
</script>
//拖拽事件
<style>
*{
margin: 0;
padding: 0;
}
.a{
width: 150px;
height: auto;
position: absolute;
border: 1px solid;
padding-bottom: 10px;
}
.target{
position: absolute;
width: 150px;
height: autopx;
border: 1px solid;
left: 300px;
padding-bottom: 10px;
}
li{
position: relative;
width: 100px;
height: 30px;
background-color: #abcdef;
margin: 10px auto 0px auto;
list-style: none;
}
</style>
<body>
<div class="a" draggable="true">
<ul>
<li></li>
<li></li>
<li></li>
</ul>
</div>
<div class="target"></div>
</body>
<script>
var dragDom;
var liList = document.getElementsByTagName("li");
for(var i = 0;i<liList.length;i++){
liList[i].setAttribute("draggable",true);
liList[i].ondragstart = function(e){
dragDom = e.target;
}
}
var box2 = document.getElementsByClassName("target")[0];
box2.ondragover = function(e){
e.preventDefault();
}
box2.ondrop = function(e){
box2.appendChild(dragDom);
dragDom = null;
}
</script>
5.DataTransfer
oDragDiv.ondragstart = function(e){
//拖动后改变鼠标样式,不常用,兼容性极差。
e.dataTransfer.effectAllowed = "link|copy|move";
}
//还有一个dropeffect 在ondrop函数中;
二,标签篇
1.语义化标签
<header></header>
<footer></footer>
<nav></nav>
<article></article>
<section></section>//段落
<name></name>
<aside></aside>//侧边栏
2.canvas画线
<style>
canvas{
width: 500px;
height: 300px;
border: 1px solid;
}
</style>
</head>
<body>
<canvas id="can" width="700px" height="500px"></canvas><!--设置画布大小-->
</body>
<script>
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
// 起点
ctx.moveTo(100,100);
// 线的粗细
ctx.lineWidth = 10;
//画
ctx.lineTo(200,100);
ctx.lineTo(200,200);
ctx.lineTo(128,298);
// 闭合(针对一个笔画来说)
ctx.closePath();
// 填充
ctx.fill();
ctx.stroke();
// 重新开启路径
ctx.beginPath();
ctx.lineWidth=5;
ctx.moveTo(300,100);
ctx.lineTo(300,200);
ctx.stroke();
</script>
//用canvas画矩形
<script>
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
//(起始位x,起始位y,长x,宽y);
// 分步画
ctx.rect(100,100,200,100);
ctx.stroke();
//直接画一个矩形
ctx.strokeRect(100,100,200,100);
//直接画出来一个填充的矩形
ctx.fillRect(100,100,200,100);
</script>
//画一个会移动的矩形
<body>
<canvas id="can" width="300px" height="300px"></canvas><!--设置画布大小-->
</body>
<script>
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
var height = 100;
//直接画一个矩形
var timer = setInterval(function(){
//相当于清屏
ctx.clearRect(0,0,500,300);
ctx.strokeRect(100,height,50,50);
height += 5;
},1000/60);
</script>
//画圆
<body>
<canvas id="can" width="300px" height="300px"></canvas><!--设置画布大小-->
</body>
<!-- 圆心(x,y),半径(r),弧度(起始弧度,结束弧度),方向(顺时针0|逆时针1) -->
<script>
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
ctx.arc(100,100,50,0,Math.PI / 2,1);
ctx.stroke();
</script>
//画圆角矩形
<body>
<canvas id="can" width="300px" height="300px"></canvas><!--设置画布大小-->
</body>
<!-- B(x,y),c(x,y),圆角大小 -->
<script>
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
ctx.moveTo(100,110);
ctx.arcTo(100,200,200,200,10);
ctx.arcTo(200,200,200,100,10);
ctx.arcTo(200,100,100,100,10);
ctx.arcTo(100,100,100,200,10);
ctx.fill();
ctx.stroke();
</script>
//贝塞尔曲线
// 二次贝塞尔曲线
ctx.quadraticCurveTo(200,200,300,100);
// 三次贝塞尔曲线
ctx.bezierCurveTo(200,200,300,100,400,200);
//canvas坐标平移旋转与缩放
扫描二维码关注公众号,回复:
9031649 查看本文章
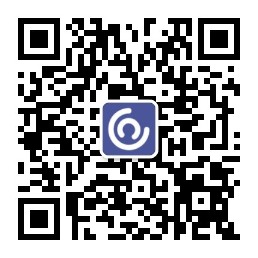
<!--旋转-->
var canvas = document.getElementById("can");
var ctx = canvas.getContext("2d");
ctx.beginPath();
// 旋转的时候是根据坐标系原点旋转的,以下是改变坐标系原点的位置
ctx.translate(100,100)
// 旋转
ctx.rotate(Math.PI / 6);
ctx.moveTo(0,0);
ctx.lineTo(100,0);
ctx.stroke();
<!--缩放-->
ctx.beginPath();
ctx.scale(2,1);//(X,Y)
ctx.strokeRect(100,100,100,100);
②canvas的save和restore
ctx.save();//保存坐标系的平移数据,缩放数据,旋转数据;
ctx.beginPath();
ctx.translate(100,100);
ctx.rotate(Math.PI / 4);
ctx.strokeRect(0,0,100,50);
ctx.beginPath();
ctx.restore();//重置画板,新图和旧图互不干扰
ctx.translate(100,100);
ctx,fillRect(200,0,100,50);
③canvas背景填充
//填充颜色
ctx.fillStyle = "blue";
//填充图片,从画板原点开始
var img = new Image();
img.src = "./good.png";
img.onload = function(){
ctx.beginPath();
//修改画板原点
ctx.translate(100,100);
var bg = ctx.creatPattern(img,"no-repeat");
ctx.fillStyle = bg;
ctx.fillRect(0,0,200,100);
}
④canvas线性渐变
var bg = ctx.createLinearGradient(0,0,200,200);
//渐变颜色
bg.addColorStop(0,"white");
bg.addColorStop(1,"black");
ctx.fillStyle = bg;
//修改坐标原点
ctx.translate(100,100);
ctx.fillRect(0,0,200,200);
⑤canvas辐射渐变
var bg = ctx.createRadialGradient(100,100,30,100,100,100);
bg.addColorStop(0,"white");
bg.addColorStop(0.5,"black");
ctx.fillStyle = bg
ctx.fillRect(0,0,200,200);
⑥canvas的阴影
ctx.shadowColor = "blue";
ctx.shadowBlur = 30;
ctx.shadowOffsetX = 15;//X方向平移了15
ctx.strokeRect(0,0,200,200);
⑦canvas渲染文字
ctx.font = "30px Georgia";
//文字描边(“文字”,x坐标,y坐标)
ctx.strokeText("wjk",200,300);
//文字填充
ctx.fillText("wjk",200,350);
⑧canvas线端样式
//线两端变圆|加方形帽子
ctx.lineCap = "round|square";
//交汇线的定点变圆滑|去掉尖|去尖锐
ctx.lineJoin = "round|bevel|miter";
⑨canvas图形叠加
context.globalCompositeOperation = 'lighter';//可以加很多值实现叠加各种效果,不一一说了;
3.svg画线与矩形
SVG:矢量图,放大不会失真,适合大面积的贴图,通常动画较少或者较简单,标签和css去画;Canvas:适合小面积的绘图,适合动画
<style>
.line1{
stroke: black;
stroke-width:3px;
}
.line2{
stroke: red;
stroke-width: 5px;
}
</style>
</head>
<body>
<svg width = "500px" height = "300px" style="border: 1px solid">
<line x1 = "100" y1 = "100" x2 = "200" y2 = "100" class="line1"></line>
<line x1 = "200" y1 = "100" x2 = "200" y2 = "200" class="line1"></line>
<!-- rx|ry:圆角 -->
<!-- 画矩形 -->
<rect height = "50" width = "100" x = "0" y = "0" rx = "10" ry = "10"></rect>
</svg>
</body>
②svg画圆和椭圆,折线
<style>
circle{
fill:transparent;
stroke:red;
}
polyline{
fill:transparent;
stroke:blueviolet;
stroke-width:3px;
}
</style>
//画圆(半径,x,y)
<circle r = "50" cx = "50" cy = "220"></circle>
//画椭圆
<ellipse rx = "100" ry = "30" cx = "400" cy = "200"></ellipse>
//画折线
<polyline points = "0 0,50 50,100 100"></polyline>
③svg画多边形和文本
//多边形
<polyline points = "0 0,5 5,"></polyline>//不会首尾相连
<polygon points = '0 0,8 8'></polygon>//首尾相连
//文本
<text x = "300" y = "50">wjk<text>
④svg透明度与线条样式
polyline{
<!-- 线条透明度-->
stroke-opacity:0.5;
<!--填充透明度-->
fill-opacity:0.3;
stroke-linecap:square|round;
//两线相交
stroke-linejoin:round|miter;
}
⑤svg的path标签
path{
stroke:red;
}
//M:移动到..开始画 L:画到..(绝对位置) l(相对位置):
<path d = "M 100 100 L 100 100 l 100 100"></path>
//M:起点 H:水平方向 Z:闭合区间 V:纵向移动
<path d = "M 100 100 H 100 V 100"></path>
⑥svg的path画弧
//椭圆
<path d = "M 100 100 A 100(x轴半径) 50(y轴半径) 0(旋转角度) 1 1(顺时针,逆时针) "></path>
⑦svg的path线性渐变
<defs>//先定义
<linearGradient id = "bg1" x1 = "0" y1 = "0" x2 = "0" y2 = "100%">
<stop offset = "0%" style = "stop-color: rgb(255,255,0)"></step>
<stop offset = "0%" style = "stop-color: rgb(255,0,0)"></step>
</linearGradient>
</defs>
⑦svg的高斯模糊
<defs>
<filter>
<feGaussianBlur in sourceGraphic stdDeviation = "20"></feGaussianBlur>
</filter>
<rect x = "100" y = "100" height = "100" width = "200" style = "filter:url(#Gaussian)">
<defs>
⑧svg的虚线及简单动画
.line1{
stroke:black;
stroke-width:10px;
//虚线
stroke-dasharray:200px;
//偏移(左偏)
stroke-dashoffset:200px;
animation:move 2s linear infinite alternate-reverse;
@keyframes move{
0%{
stroke-dashoffset:200px;
100%{
stroke-dashoffset:0px;
}
}
}
}
⑦svg的viewbox(比例尺)
//放大一倍
<svg width = "500px" height = "300px" viewBox = "0(x),0(y),250,150" style = "border:1 px solid">
4.audio与video播放器
<audio src = "xxx.mov"></audio>
<video src = "xxx.mov"></video>
做视频播放器案例,明天补上。
-------------------------------待续---------------------------------------------