文件名与要引用的包名同名
比如你要引用requests,但是自己给自己的文件起名也叫requests.py,这样执行下面代码
import requests
requests.get('http://www.baidu.com')
就会报如下错误
AttributeError: module 'requests' has no attribute 'get'
解决方法是给你的python文件名换个名字,只要不和包名相同就行,如果实在不想改文件名,可以用下面的办法
import sys
_cpath_ = sys.path[0]
print(sys.path)
print(_cpath_)
sys.path.remove(_cpath_)
import requests
sys.path.insert(0, _cpath_)
requests.get('http://www.baidu.com')
主要原理是将当前目录排除在python运行是的查找目录,这种处理后在命令行执行python requests.py是可以正常运行的,但是在pycharm里调试和运行通不过。
格式不对齐的问题
下面是一段正常代码
def fun():
a=1
b=2
if a>b:
print("a")
else:
print("b")
fun()
1.如果else不对齐
def fun():
a=1
b=2
if a>b:
print("a")
else:
print("b")
fun()
就会报
IndentationError: unindent does not match any outer indentation level
2.如果else和if没有成对出现,比如直接写一个else或者多写了一个else,或者if和else后面的冒号漏写
def fun():
a=1
b=2
else:
print("b")
fun()
def fun():
a=1
b=2
if a>b:
print("a")
else:
print("b")
else:
print("b")
fun()
def fun():
a=1
b=2
if a>b:
print("a")
else
print("b")
fun()
都会报
SyntaxError: invalid syntax
3.如果if和else下面的语句没有缩进
def fun():
a=1
b=2
if a>b:
print("a")
else:
print("b")
fun()
就会报
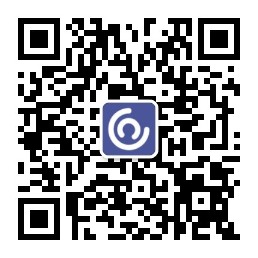
IndentationError: expected an indented block
字符串使用中文的引号
比如下面用中文引号
print(“a”)
就会报
SyntaxError: invalid character in identifier
正确的方式是使用英文的单引号或者双引号
print('b')
print("b")
没用调用函数,误认为函数不执行
比如下面的代码
class A(object):
def run(self):
print('run')
a = A()
a.run
程序是正常的,运行也没有报错,但是可能好奇为什么没有打印输出,原因当然是因为a.run返回的函数的引用,并没有执行,要调用a.run()才会发现有打印输出。
如果修改下代码,改成
class A(object):
def run(self):
return 1
a = A()
print(a.run+1)
就会看到报错
TypeError: unsupported operand type(s) for +: 'method' and 'int'
改成a.run()+1后便正常了。
字符串格式化
这是一段正常的程序
a = 1
b = 2
print('a = %s'%a)
print('a,b = %s,%s'%(a,b))
1.如果少写了%s
a = 1
b = 2
print('a = '%a) # 错误
print('a,b = %s'%(a,b)) # 错误
会报
TypeError: not all arguments converted during string formatting
2.如果多写了%s
a = 1
b = 2
print('a = %s,%s'%a)
print('a,b = %s,%s,%s'%(a,b))
``
会报
```bash
TypeError: not enough arguments for format string
3.如果格式化的字符串不对,比如写成大写的s
a = 1
b = 2
print('a = %S'%a)
print('a,b = %S,%S'%(a,b))
会报
ValueError: unsupported format character 'S' (0x53) at index 5
4.如果%后面什么都没写
a = 1
b = 2
print('a = %'%a)
print('a,b = %,%'%(a,b))
会报
ValueError: incomplete format
对None操作的报错
比如这段代码
a = [3,2,1]
b = a.sort()
print(a)
print(b)
a排序后的值是[1,2,3],sort函数的返回值是None,所以b的值其实时None,并不是[1,2,3]。
如果这时候去操作b
比如取b的第一个元素
print(b[0])
则会报错
TypeError: 'NoneType' object is not subscriptable
比如复制数组
print(b*2)
则会报错
TypeError: unsupported operand type(s) for *: 'NoneType' and 'int'
比如执行extend函数
b.extend([4])
则会报错
AttributeError: 'NoneType' object has no attribute 'extend'
整数相除
python2里面默认整数相除是返回整数部分
print(3/2)
返回是1
而python3里面返回是小数
print(3/2)
返回是1.5
所以下面这样的代码
a = [1,2,3]
print(a[len(a)/2])
在python2里可以得到值为2,而在python3里执行确会得到报错
TypeError: list indices must be integers or slices, not float
修改的方法有两种
1.把结果转为整数
a = [1,2,3]
print(a[int(len(a)/2)])
2.用//号
a = [1,2,3]
print(a[len(a)//2])
这两种写法同样适用于python2,所以期望整数返回的话建议用双除号,显式表达自己的意图
主键不存在
获取的建字典中不存在
a = {'A':1}
print(a['a'])
会报错
KeyError: 'a'
pip安装包的时候网络超时
类似如下错误
bash WARNING: Retrying (Retry(total=3, connect=None, read=None, redirect=None, status=None)) after connection broken by 'ConnectTimeoutError(<pip._vendor.urllib3.connection.VerifiedHTTPSConnection object at 0x7f3b6ea319b0>, 'Connection to files.pythonhosted.org timed out. (connect timeout=15)')': /packages/xxxxxx
建议设置镜像源,比如更换为清华源,参考传送门
也有可能碰到没有加trusted-host参数而出现如下错误
Collecting xxx
The repository located at pypi.tuna.tsinghua.edu.cn is not a trusted or secure host and is being ignored. If this repository is available via HTTPS it is recommended to use HTTPS instead, otherwise you may silence this warning and allow it anyways with ‘--trusted-host pypi.tuna.tsinghua.edu.cn’.
Could not find a version that satisfies the requirement xxx (from versions: )
No matching distribution found for xxx
安装命令增加–trust-host即可
pip install -i https://pypi.tuna.tsinghua.edu.cn/simple --trusted-host pypi.tuna.tsinghua.edu.cn xxx