引入布局
问题:我们的程序中可能有很多活动都需要标题栏,如果在每个活动的布局中都编写一遍同样的标题栏代码,明显会导致代码的大量重复
解决方案:引入布局
一、title.xml:设置标题栏布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#ff0000">
<Button
android:id="@+id/title_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:text="Back"
android:textColor="#fff"
/>
<TextView
android:id="@+id/title_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_gravity="center"
android:gravity="center"
android:text="Title Text"
android:textColor="#fff"
android:textSize="24sp"
/>
<Button
android:id="@+id/title_edit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:text="Edit"
android:textColor="#fff"
/>
</LinearLayout>
二、如何在程序中使用该标题栏:activity_main.xml
通过一个include语句引入进来即可
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 将标题栏布局引入进来 -->
<include
layout="@layout/title"
/>
</LinearLayout>
创建自定义控件
引入布局的技巧解决了重复编写布局代码的问题。
问题:但是如果布局中某些控件要求能够响应外部事件,需要在每个活动中单独编写一次事件编写的代码。比如说:标题栏的返回按钮---->不管在哪个活动中,这个按钮的功能都相同,即销毁当前活动。而如果在每个活动中都需要重新注册一遍返回按钮的点击事件,无疑会增加很多重复代码
解决方案:自定义控件
一、TitleLayout,继承自LinearLayout,自定义的标题栏控件
package com.example.diyviews;
import android.content.Context;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.widget.LinearLayout;
import androidx.annotation.Nullable;
public class TitleLayout extends LinearLayout {
public TitleLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
LayoutInflater.from(context).inflate(R.layout.title,this);
}
}
二、activity_main.xml:在布局文件中添加上面的自定义控件
扫描二维码关注公众号,回复:
8973291 查看本文章
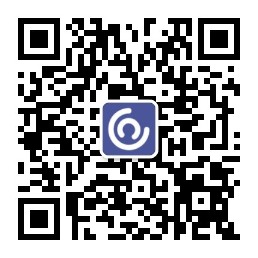
添加自定义控件个普通该控件的方式基本一样,只不过添加自定义控件,需要指明控件的完整类名
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 添加自定义控件,需要指明控件的完整类名 -->
<com.example.diyviews.TitleLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
三、修改TitleLayout代码:为标题栏的按钮注册点击事件
package com.example.diyviews;
import android.app.Activity;
import android.content.Context;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.Toast;
import androidx.annotation.Nullable;
public class TitleLayout extends LinearLayout {
public TitleLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
LayoutInflater.from(context).inflate(R.layout.title,this);
Button titleBack=(Button)findViewById(R.id.title_back);
Button titleEdit=(Button)findViewById(R.id.title_edit);
titleBack.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
((Activity)getContext()).finish();
}
});
titleEdit.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getContext(),"You clicked Edit Button",Toast.LENGTH_SHORT).show();
}
});
}
}
疑问:
((Activity)getContext()).finish();
Toast.makeText(getContext(),"You clicked Edit Button",Toast.LENGTH_SHORT).show();
答疑:
getContext():这个是View类中提供的方法,在继承了View的类中才可以调用。返回的是当前View运行在哪个Activity Contex中(获取当前context的实例)
四、MainActivity.java
package com.example.diyviews;
import androidx.appcompat.app.ActionBar;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//将系统自带的标题栏隐藏掉
ActionBar actionBar=getSupportActionBar();//获取ActionBar实例
if(actionBar!=null){
actionBar.hide();
}
}
}
getSupportActionBar() 获取当前ActionBar的实例
hide() 将标题栏隐藏起来
五、效果图