647. Palindromic Substrings**
https://leetcode.com/problems/palindromic-substrings/
题目描述
Given a string, your task is to count how many palindromic substrings in this string.
The substrings with different start indexes or end indexes are counted as different substrings even they consist of same characters.
Example 1:
Input: "abc"
Output: 3
Explanation: Three palindromic strings: "a", "b", "c".
Example 2:
Input: "aaa"
Output: 6
Explanation: Six palindromic strings: "a", "a", "a", "aa", "aa", "aaa".
Note:
- The input string length won’t exceed 1000.
C++ 实现 1
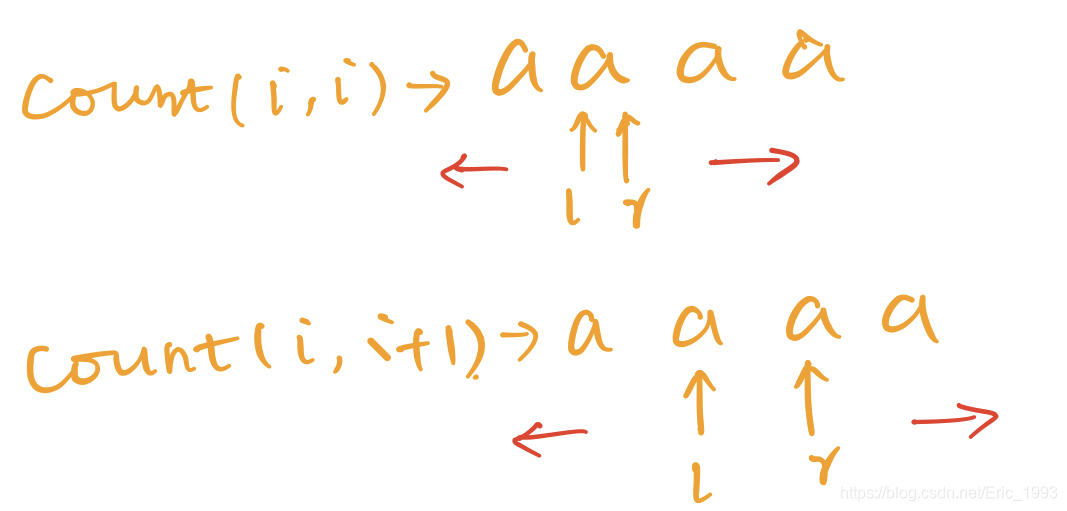
class Solution {
private:
int count(const string &s, int l, int r) {
int res = 0;
while (l >= 0 && r < s.size() && s[l--] == s[r++])
res ++;
return res;
}
public:
int countSubstrings(string s) {
int res = 0, n = s.size();
for (int i = 0; i < n; ++i) {
res += count(s, i, i);
res += count(s, i, i + 1);
}
return res;
}
};
C++ 实现 2
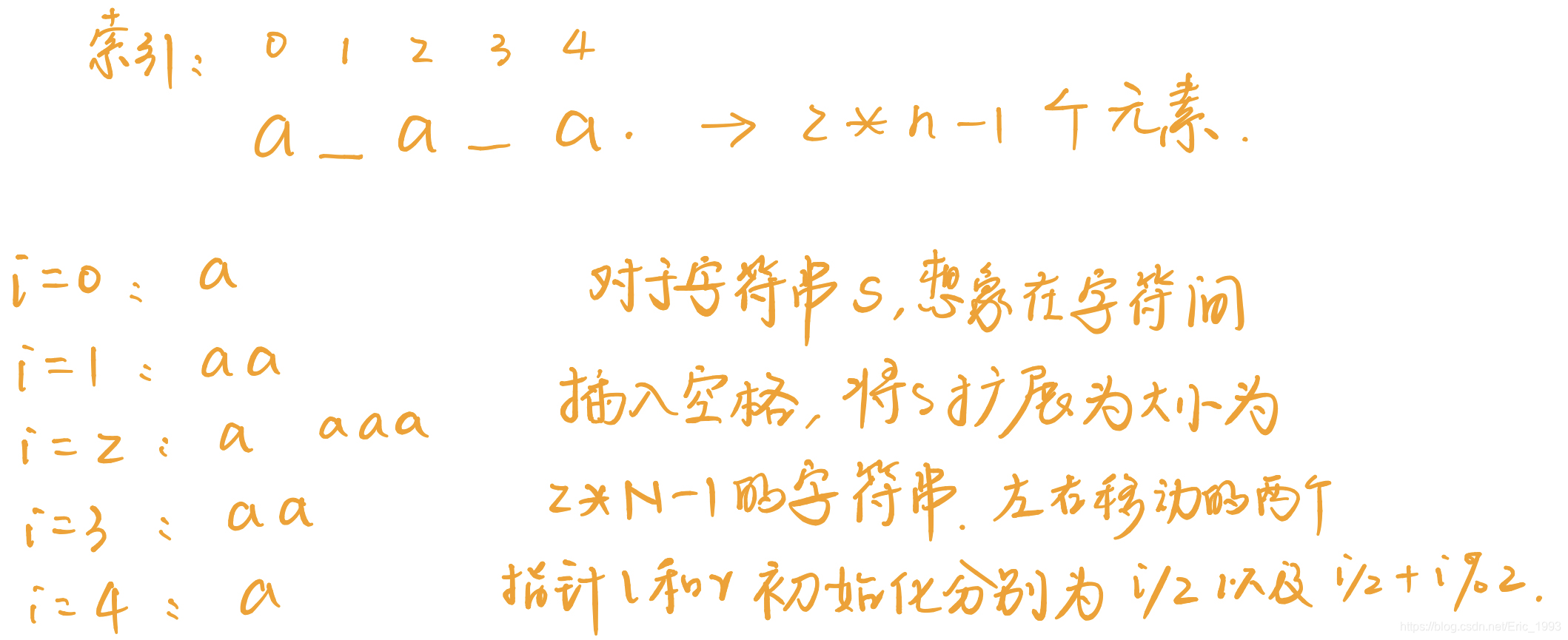
参考了 Approach #1: Expand Around Center [Accepted]
class Solution {
public:
int countSubstrings(string s) {
int res = 0, n = s.size();
for (int i = 0; i < 2 * n - 1; ++i) {
int l = i / 2;
int r = l + i % 2;
while (l >= 0 && r < n && s[l--] == s[r++])
res ++;
}
return res;
}
};
C++ 实现 3
动态规划的方法. 使用 dp[i][j]
表示字符串 s[i,....j]
是否为回文串. 此时查找 dp[i][j]
和 dp[i + 1][j - 1]
的关系就是查找 s[i, ..., j]
与 s[i + 1,..., j - 1]
的关系. 由于 s[i, ..., j]
为回文串的要求是 s[i + 1,..., j - 1]
为回文串并且 s[i] == s[j]
, 因此有:
dp[i][j] = (s[i] == s[j]) && (dp[i + 1][j - 1])
另外注意, 对于 a
, aa
, aba
这样的子字符串, 大小 j - i < 3
, 只需判断 s[i]
是否等于 s[j]
, 因此将递推公式改为:
dp[i][j] = (s[i] == s[j]) && (j - i < 3 || dp[i + 1][j - 1])
可以简化边界情况的考虑.
代码中 i
从 n - 1
变化到 0
, 从后向前考虑.
扫描二维码关注公众号,回复:
8917210 查看本文章
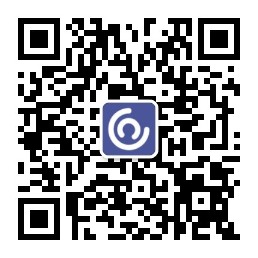
class Solution {
public:
int countSubstrings(string s) {
int res = 0, n = s.size();
vector<vector<bool>> dp(n, vector<bool>(n, false));
for (int i = n - 1; i >= 0; --i)
for (int j = i; j < n; ++j) {
dp[i][j] = (s[i] == s[j]) && (j - i < 3 || dp[i + 1][j - 1]);
if (dp[i][j]) ++ res;
}
return res;
}
};