本章将以一个文本框数值验证作为案例讲解指令。
首先先定义一个私有命令
<template>
<div>
<input width="200px" v-errorInfo.color="'red'" v-model="msg">
</div>
</template>
<script>
/**
* 自定义指令
*/
export default {
name: "Vue03",
data(){
return{
msg:''
}
},
directives: {
errorInfo: {
bind: function (el, binding, vnode) {
//创建了一个元素,行为不要写在此处,因为还未插入到页面中
console.log('bind---------')
},
inserted: function (el, binding, vnode) {
//将元素插入页面
console.log('inserted-------')
console.log('el',el)
console.log('name',binding.name)
console.log('value',binding.value)
console.log('argument',binding.arg)
console.log('modifiers',binding.modifiers)
},
update: function (el, binding, vnode,oldVnode) {
//绑定值更新后
console.log('update-------')
console.log('el',el)
console.log('binding',binding)
console.log('vnode',vnode)
console.log('oldVnode',oldVnode)
}
}
}
}
</script>
<style scoped>
</style>
我们看一下输出
在bind的时候判断一下,dom对象是不是input元素
bind: function (el, binding, vnode) {
//创建了一个元素,行为不要写在此处,因为还未插入到页面中
console.log('bind---------')
if (el.nodeName.toLocaleLowerCase()!='input'){
console.error("绑定类型不对,请检查")
}
},
之后再更新的时候判断值,如果值不是数字则将其变成设置的颜色
<template>
<div>
<input width="200px" v-errorInfo.color="'red'" v-model="msg">
</div>
</template>
<script>
/**
* 自定义指令
*/
export default {
name: "Vue03",
data(){
return{
msg:''
}
},
directives: {
errorInfo: {
bind: function (el, binding, vnode) {
//创建了一个元素,行为不要写在此处,因为还未插入到页面中
console.log('bind---------')
if (el.nodeName.toLocaleLowerCase()!='input'){
console.error("绑定类型不对,请检查")
}
},
inserted: function (el, binding, vnode) {
//将元素插入页面
console.log('inserted-------')
},
update: function (el, binding, vnode,oldVnode) {
//绑定值更新后
console.log('update-------')
if(isNaN(el.value)){
//如果不是一场将将颜色变为设置的颜色
el.style.color=binding.value;
}else{
el.style.color='black';
}
}
}
}
}
</script>
<style scoped>
</style>
全局指令
文件结构如下
directive/index.js
扫描二维码关注公众号,回复:
8895008 查看本文章
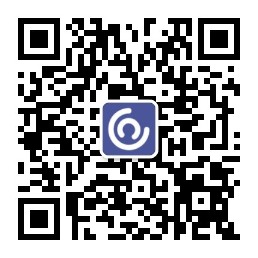
const directives={
numErrorInfo: {
bind: function (el, binding, vnode) {
//创建了一个元素,行为不要写在此处,因为还未插入到页面中
console.log('bind---------')
if (el.nodeName.toLocaleLowerCase()!='input'){
console.error("绑定类型不对,请检查")
}
},
inserted: function (el, binding, vnode) {
//将元素插入页面
console.log('inserted-------')
},
update: function (el, binding, vnode,oldVnode) {
//绑定值更新后
console.log('update-------')
if(isNaN(el.value)){
//如果不是一场将将颜色变为设置的颜色
el.style.color=binding.value;
}else{
el.style.color='black';
}
}
}
}
export default directives;
main.js中注册
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
import filters from "./filter";
import directives from "./directive";
Vue.config.productionTip = false
for (let key in filters) {
Vue.filter(key, filters[key]);
}
for (let key in directives) {
Vue.directive(key, directives[key]);
}
new Vue({
el: '#app',
router,
components: { App },
template: '<App/>'
})