SSM整合 级联查询 基于【SSM整合篇】三
github源码(day57-cascade) https://github.com/1196557363/ideaMavenProject
项目准备
-
本项目基于 【SSM整合篇】 三
SSM整合篇】三. SSM整合+事务+单元测试案例 第一章(共四章)https://blog.csdn.net/TheNew_One/article/details/103888883
SSM整合篇】三. SSM整合+事务+单元测试案例 第二章(共四章)https://blog.csdn.net/TheNew_One/article/details/103896990
SSM整合篇】三. SSM整合+事务+单元测试案例 第三章(共四章)https://blog.csdn.net/TheNew_One/article/details/103897975
SSM整合篇】三. SSM整合+事务+单元测试案例 完结 (共四章)https://blog.csdn.net/TheNew_One/article/details/103898570 -
新建项目day57-cascade并将上一天的项目的代码资源文件全部拿过来。
-
删除User对象,用于测试事务的,在该案例用不到。
-
mysql我这里还是用day56-ssm-transaction
-
加个log4j.properties 日志配置文件
#是日志记录的优先级,分为OFF、FATAL、ERROR、WARN、INFO、DEBUG、ALL或
log4j.rootLogger = debug,stdout,E,MAIL
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.Target=System.out
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.Encoding=UTF-8
log4j.appender.stdout.layout.ConversionPattern= %d{yyy-MM-dd HH\:mm\:ss} %p %c{0}\:%L - %m%n
1. 查询所有部门
1.1 写dao,mapper,service及其impl
1.1.1 IDeptDao
package com.wpj.dao;
import com.wpj.bean.*;
import java.util.*;
/**
* ClassName: IDeptDao
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 19:12
* @since JDK 1.8
*/
public interface IDeptDao {
/**
* 获取所有的部门
* @return
*/
List<Dept> getAllDept();
}
1.1.2 IDeptDao.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wpj.dao.IDeptDao">
<resultMap id="deptMap" type="dept">
<id property="deptId" column="dept_id" />
<result property="deptName" column="dept_name" />
<result property="deptAddr" column="dept_addr" />
</resultMap>
<!-- 获取所有的部门 -->
<select id="getAllDept" resultMap="deptMap">
select * from dept
</select>
</mapper>
1.1.3 IDeptService
package com.wpj.service;
import com.wpj.bean.*;
import org.springframework.stereotype.*;
import java.util.*;
/**
* ClassName: IDeptService
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 19:46
* @since JDK 1.8
*/
public interface IDeptService {
/**
* 获取所有的部门
* @return
*/
List<Dept> getAllDept();
}
1.1.4 DeptServiceImpl
package com.wpj.service.impl;
import com.wpj.bean.*;
import com.wpj.dao.*;
import com.wpj.service.*;
import org.springframework.beans.factory.annotation.*;
import org.springframework.stereotype.*;
import java.util.*;
/**
* ClassName: DeptServiceImpl
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 20:00
* @since JDK 1.8
*/
@Service
public class DeptServiceImpl implements IDeptService {
@Autowired
private IDeptDao iDeptDao;
public List<Dept> getAllDept() {
return iDeptDao.getAllDept();
}
}
1.2 写controller
package com.wpj.web.controller;
import com.wpj.bean.*;
import com.wpj.service.*;
import org.springframework.beans.factory.annotation.*;
import org.springframework.stereotype.*;
import org.springframework.ui.*;
import org.springframework.web.bind.annotation.*;
import java.util.*;
/**
* ClassName: DeptController
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\9 0009 11:55
* @since JDK 1.8
*/
@Controller
@RequestMapping("/dept")
public class DeptController {
@Autowired
private IDeptService iDeptService;
@RequestMapping("getAllDept")
public String getAllDept(ModelMap map){
List<Dept> deptList = iDeptService.getAllDept();
map.put("deptList",deptList);
return "deptList";
}
}
1.2 创建deptList.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<table>
<tr>
<td>id</td>
<td>name</td>
<td>address</td>
</tr>
<tr th:each="dept:${deptList}">
<td th:text="${dept.deptId}"></td>
<td th:text="${dept.deptName}"></td>
<td th:text="${dept.deptAddr}"></td>
</tr>
</table>
</body>
</html>
1.4 运行
http://localhost:8080/dept/getAllDept
1.5 结果
2. 根据查询来的部门查询该部门的员工
2.1 修改dept.html的部门为超链接
<tr th:each="dept:${deptList}">
<td th:text="${dept.deptId}" />
<td>
<a th:text="${dept.deptName}" th:href="|emp/getEmpByDeptId/${dept.deptId}|" />
</td>
<td th:text="${dept.deptAddr}" />
</tr>
2.2 写dao,mapper,service及其impl
2.2.1 IEmpDao 定义getEmpByDeptId方法
package com.wpj.dao;
import com.wpj.bean.*;
import org.apache.ibatis.annotations.*;
import java.util.*;
/**
* ClassName: IEmpDao
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 19:11
* @since JDK 1.8
*/
public interface IEmpDao {
/**
* 获取所有的Emp
* @return
*/
List<Emp> getAllEmp();
/**
* 根据部门ID获取该部门下所有员工
* @return
*/
List<Emp> getEmpByDeptId(@Param("deptId") Integer deptId);
}
2.2.2 IEmpDao.xml 映射getEmpByDeptId
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wpj.dao.IEmpDao">
<resultMap id="empMap" type="emp">
<id property="empId" column="emp_id"/>
<result property="empName" column="emp_name" />
<result property="job" column="job" />
<result property="superId" column="super_id" />
<result property="deptNo" column="dept_no" />
</resultMap>
<select id="getEmpByDeptId" resultMap="empMap">
select * from emp where dept_no = #{deptId}
</select>
</mapper>
2.2.3 IEmpService
package com.wpj.service;
import com.wpj.bean.*;
import org.apache.ibatis.annotations.*;
import java.util.*;
/**
* ClassName: IEmpService
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 19:47
* @since JDK 1.8
*/
public interface IEmpService {
/**
* 根据部门ID获取该部门下所有员工
* @return
*/
List<Emp> getEmpByDeptId(Integer deptId);
}
2.2.4 IEmpServiceImpl
package com.wpj.service.impl;
import com.wpj.bean.*;
import com.wpj.dao.*;
import com.wpj.service.*;
import org.springframework.beans.factory.annotation.*;
import org.springframework.stereotype.*;
import java.util.*;
/**
* ClassName: EmpServiceImpl
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 20:03
* @since JDK 1.8
*/
@Service
public class EmpServiceImpl implements IEmpService {
@Autowired
private IEmpDao iEmpDao;
public List<Emp> getEmpByDeptId(Integer deptId) {
return iEmpDao.getEmpByDeptId(deptId);
}
}
2.3 写controller
package com.wpj.web.controller;
import com.wpj.bean.*;
import com.wpj.service.*;
import org.springframework.beans.factory.annotation.*;
import org.springframework.stereotype.*;
import org.springframework.ui.*;
import org.springframework.validation.annotation.*;
import org.springframework.web.bind.annotation.*;
import java.util.*;
/**
* ClassName: Controller
* Description:
*
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 21:55
* @since JDK 1.8
*/
@Controller
@RequestMapping("/emp")
public class EmpController {
@Autowired
private IEmpService iEmpService;
@RequestMapping("/getEmpByDeptId/{deptId}")
public String getEmpByDeptId(@PathVariable Integer deptId,ModelMap map){
List<Emp> empList = iEmpService.getEmpByDeptId(deptId);
map.put("empList",empList);
return "empList";
}
}
2.4 创建empList.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<base th:href="${#request.getContextPath()+'/'}">
</head>
<table>
<tr>
<td>id</td>
<td>name</td>
<td>job</td>
<td>superId</td>
<td>deptNo</td>
<td>operation</td>
</tr>
<tr th:each="emp:${empList}">
<td th:text="${emp.empId}" />
<td th:text="${emp.empName}" />
<td th:text="${emp.job}" />
<td th:text="${emp.superId}" />
<td th:text="${emp.deptNo}" />
<td>
<a href="#">编辑</a>
<a href="#">删除</a>
</td>
</tr>
</table>
</body>
</html>
2.5 运行
2.6 结果
3. 关联查询Emp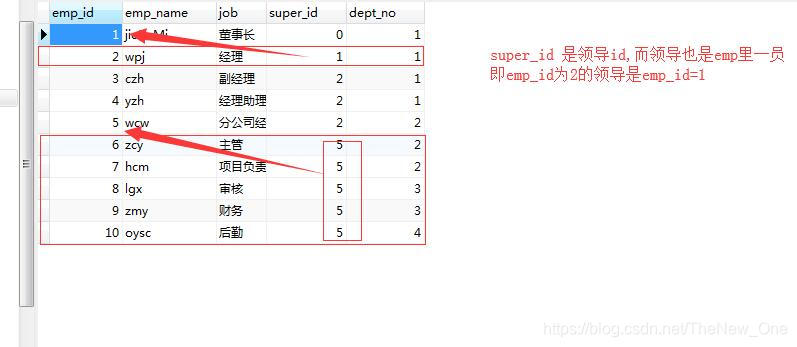
3.1 修改实体类Emp
添加2个对象属性并提供无参,全参,getter,setter和toString()
package com.wpj.bean;
/**
* ClassName: Emp
* Description:
* 提供无参,全参,getter,setter和toString()
* @author JieKaMi
* @version 1.0
* @date: 2020\1\8 0008 19:07
* @since JDK 1.8
*/
public class Emp {
private Integer empId;
private String empName;
private String job;
// 领导id
private Integer superId;
// 领导对象
private Emp superEmp;
// 部门id
private Integer deptNo;
// 部门对象
private Dept dept;
public Emp() {}
public Emp(Integer empId, String empName, String job, Integer superId, Integer deptNo) {
this.empId = empId;
this.empName = empName;
this.job = job;
this.superId = superId;
this.deptNo = deptNo;
}
public Emp(Integer empId, String empName, String job, Integer superId, Emp superEmp, Integer deptNo, Dept dept) {
this.empId = empId;
this.empName = empName;
this.job = job;
this.superId = superId;
this.superEmp = superEmp;
this.deptNo = deptNo;
this.dept = dept;
}
public Integer getEmpId() { return empId; }
public void setEmpId(Integer empId) { this.empId = empId; }
public String getEmpName() { return empName; }
public void setEmpName(String empName) { this.empName = empName; }
public String getJob() { return job; }
public void setJob(String job) { this.job = job; }
public Integer getSuperId() { return superId; }
public void setSuperId(Integer superId) { this.superId = superId; }
public Integer getDeptNo() { return deptNo; }
public void setDeptNo(Integer deptNo) { this.deptNo = deptNo; }
public Emp getSuperEmp() { return superEmp; }
public void setSuperEmp(Emp superEmp) { this.superEmp = superEmp; }
public Dept getDept() { return dept; }
public void setDept(Dept dept) { this.dept = dept; }
@Override
public String toString() {
return "Emp{" +
"empId=" + empId +
", empName='" + empName + '\'' +
", job='" + job + '\'' +
", superId=" + superId +
", superEmp=" + superEmp +
", deptNo=" + deptNo +
", dept=" + dept +
'}';
}
}
3.2 修改mapper及其里面的<resultMap>
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wpj.dao.IEmpDao">
<resultMap id="empMap" type="emp">
<id property="empId" column="emp_id"/>
<result property="empName" column="emp_name" />
<result property="job" column="job" />
<result property="superId" column="super_id" />
<result property="deptNo" column="dept_no" />
<association property="superEmp" javaType="emp">
<result property="empName" column="superName" />
</association>
</resultMap>
<select id="getEmpByDeptId" resultMap="empMap">
SELECT
e1.*, e2.emp_name AS superName
FROM
emp e1
LEFT JOIN emp e2 ON (e1.super_id = e2.emp_id)
WHERE
e1.dept_no = #{deptId}
</select>
</mapper>
3.2 修改IEmpList.html
添加一行td 显示领导名字
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<base th:href="${#request.getContextPath()+'/'}">
</head>
<table>
<tr>
<td>id</td>
<td>name</td>
<td>job</td>
<td>superId</td>
<td>superName</td>
<td>deptNo</td>
<td>operation</td>
</tr>
<tr th:each="emp:${empList}">
<td th:text="${emp.empId}" />
<td th:text="${emp.empName}" />
<td th:text="${emp.job}" />
<td th:text="${emp.superId}" />
<!-- 如果该员工就是最上级 即没有领导就进行判断 -->
<td th:text="${emp.superEmp !=null?emp.superEmp.empName:''}" />
<td th:text="${emp.deptNo}" />
<td>
<a href="#">编辑</a>
<a href="#">删除</a>
</td>
</tr>
</table>
</body>
</html>