TensorRT/samples/common/argsParser.h源碼研讀
argsParser.h
/*
* Copyright (c) 2019, NVIDIA CORPORATION. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
#ifndef TENSORRT_ARGS_PARSER_H
#define TENSORRT_ARGS_PARSER_H
#include <string>
#include <vector>
#ifdef _MSC_VER
#include "..\common\windows\getopt.h"
#else
#include <getopt.h>
#endif
#include <iostream>
namespace samplesCommon
{
//!
//! \brief The SampleParams structure groups the basic parameters required by
//! all sample networks.
//!
struct SampleParams
{
int batchSize{1}; //!< Number of inputs in a batch
int dlaCore{-1}; //!< Specify the DLA core to run network on.
bool int8{false}; //!< Allow runnning the network in Int8 mode.
bool fp16{false}; //!< Allow running the network in FP16 mode.
std::vector<std::string> dataDirs; //!< Directory paths where sample data files are stored
std::vector<std::string> inputTensorNames;
std::vector<std::string> outputTensorNames;
};
//!
//! \brief The CaffeSampleParams structure groups the additional parameters required by
//! networks that use caffe
//!
// 在SampleParams的基礎上加上caffe特有的參數
struct CaffeSampleParams : public SampleParams
{
std::string prototxtFileName; //!< Filename of prototxt design file of a network
std::string weightsFileName; //!< Filename of trained weights file of a network
std::string meanFileName; //!< Filename of mean file of a network
};
//!
//! \brief The OnnxSampleParams structure groups the additional parameters required by
//! networks that use ONNX
//!
struct OnnxSampleParams : public SampleParams
{
std::string onnxFileName; //!< Filename of ONNX file of a network
};
//!
//! \brief The UffSampleParams structure groups the additional parameters required by
//! networks that use Uff
//!
struct UffSampleParams : public SampleParams
{
std::string uffFileName; //!< Filename of uff file of a network
};
//!
//! /brief Struct to maintain command-line arguments.
//!
//parseArgs解析完命令行的參數後,會把得到的資訊存入此結構體內
struct Args
{
bool runInInt8{false};
bool runInFp16{false};
bool help{false};
int useDLACore{-1};
int batch{1};
std::vector<std::string> dataDirs;
//?
bool useILoop{false};
};
//!
//! \brief Populates the Args struct with the provided command-line parameters.
//!
//! \throw invalid_argument if any of the arguments are not valid
//!
//! \return boolean If return value is true, execution can continue, otherwise program should exit
//!
//解析argv,將所得到的資訊存入args這個struct Args型別的變數內
inline bool parseArgs(Args& args, int argc, char* argv[])
{
//無窮迴圈。等到參數解析完了,arg會自動變成-1,接著跳出迴圈
while (1)
{
int arg;
static struct option long_options[] = {{"help", no_argument, 0, 'h'}, {"datadir", required_argument, 0, 'd'},
{"int8", no_argument, 0, 'i'}, {"fp16", no_argument, 0, 'f'}, {"useILoop", no_argument, 0, 'l'},
{"useDLACore", required_argument, 0, 'u'}, {"batch", required_argument, 0, 'b'}, {nullptr, 0, nullptr, 0}};
int option_index = 0;
// getopt_long用於解析傳入的參數,定義於Linux系統自帶的getopt.h,
// 亦或是samples/common/windows/getopt.c
// 在getopt_long被呼叫的過程中,option_index會隨之變化,代表該次解析的是long_options裡的第幾個參數
//getopt_long的回傳值是struct option裡最後一個元素,即'h','d','i','f','l','u','b'或0
//注意到d後面跟著一個冒號,這代表-d後面還跟著一個參數
//為何這裡optstring只有'h','d','i','u',少了'f','l','b'及0?
arg = getopt_long(argc, argv, "hd:iu", long_options, &option_index);
// 如果所有可選參數都解析完了,則getopt_long回傳-1
if (arg == -1)
{
break;
}
switch (arg)
{
//parseArgs的參數args傳入時是空的,在這個block裡依不同情況來為args設值
case 'h': args.help = true; return true;
case 'd':
//optarg定義於samples/common/windows/getopt.c,由getopt_long間接地被賦值,表示可選參數
//-d後跟著的參數會被解析到optarg內
if (optarg)
{
args.dataDirs.push_back(optarg);
}
else
{
std::cerr << "ERROR: --datadir requires option argument" << std::endl;
return false;
}
break;
case 'i': args.runInInt8 = true; break;
//'f'不在optstring內,能被解析出來?
case 'f': args.runInFp16 = true; break;
//?
//'l'不在optstring內,能被解析出來?
case 'l': args.useILoop = true; break;
case 'u':
//在optstring中,u後面沒加冒號,為何這裡可以有參數?
if (optarg)
{
args.useDLACore = std::stoi(optarg);
}
break;
case 'b':
//'b'不在optstring內,能被解析出來?
if (optarg)
{
args.batch = std::stoi(optarg);
}
break;
//如果傳入的參數不是上面的任一個,則程序執行失敗
default: return false;
}
}
return true;
}
} // namespace samplesCommon
#endif // TENSORRT_ARGS_PARSER_H
namespace
代碼中定義了名為samplesCommon
的namespace。關於namespace,詳見C++ namespace。
struct的繼承
CaffeSampleParams
結構體是繼承自SampleParams
結構體,
關於struct的繼承,詳見C++ struct的繼承。
caffe特有參數
-
prototxtFileName:預期的參數是以
.prototxt
結尾的檔名,代表網路結構定義 -
weightsFileName:預期的參數是以
.caffemodel
結尾的檔名,代表網路權重 -
meanFileName:預期的參數是以
.binaryproto
結尾的檔名,代表圖像的平均值
UFF格式
摘自4.3.1. Example 1: Adding A Custom Layer To A TensorFlow Model:
In order to run a TensorFlow network with TensorRT, you must first convert it to the UFF format.
UFF格式似乎是為了將TensorFlow與TensorRT的模型互相轉換而定義的一種中間表示方式?
不需要typedef struct xxx yyy?
記得在C語言中,定義完一個struct之後,我們通常還會加上typedef
,用以簡化之後與該struct相關的代碼。但是在argsParser.h
中,定義完Args
這個結構體後,我們卻可以直接在parseArgs
函數裡使用它,這是為什麼呢?詳見C++ typedef struct v.s. struct。
struct初始化
在parseArgs
函數中定義了一個struct option
型別的變數long_options
,對它初始化的方式就像在初始化array一樣。關於struct的初始化方式,詳見C++ struct初始化。
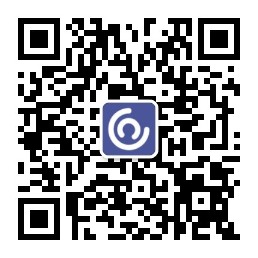
struct option,getopt_long
在parseArgs
函數中定義了一個struct option
型別的變數long_options
,還用到了getopt_long
這個函數,詳見C getopt.h。
inline
parseArgs
函數被宣告為inline function,關於inline function,詳見C++ inline function。
DLA
NVDLA是NVIDIA開源的深度學習加速架構,包含了硬件及軟件部份。
參考連結
4.3.1. Example 1: Adding A Custom Layer To A TensorFlow Model
THE NVIDIA DEEP LEARNING ACCELERATOR