Posted on 2008-01-17 16:30 sunrack 阅读(167) 评论(0) 编辑 收藏 网摘 所属分类: LINQ
一、什么时候使用Lambda表达式
总的来说,Lambda 表达式可以用在任何需要使用匿名方法,或是代理的地方。编译器会将Lambda表达式编译为标准的匿名方法(可以使用ildasm.exe or reflector.exe得到确认)。
比如:
List<int> evenNumbers = list.FindAll(i => (i % 2) == 0);
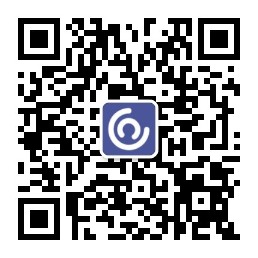
被编译为
List<int> evenNumbers = list.FindAll(delegate (int i)
{
return (i % 2) == 0;
});
二、Lambda表达式的解读
Lambda表达式的写法
ArgumentsToProcess (参数)=> StatementsToProcessThem(报表)
比如
// "i" is our parameter list.
// "(i % 2) == 0" is our statement set to process "i".
List<int> evenNumbers = list.FindAll(i => (i % 2) == 0);
应该这样来理解
// My list of parameters (in this case a single integer named i)
// will be processed by the expression (i % 2) == 0.
List<int> evenNumbers = list.FindAll((i) => ((i % 2) == 0));
可以显式指定输入参数的类型
List<int> evenNumbers = list.FindAll((int i) => (i % 2) == 0);
可以使用括号把输入参数和表达式括起来,如果参数或处理表达式只有一个,可以省略括号
List<int> evenNumbers = list.FindAll((i) => ((i % 2) == 0));
有多行处理表达式时,需要使用花括号包起来
List<int> evenNumbers = list.FindAll((i) =>
{
Console.WriteLine("value of i is currently: {0}", i);
bool isEven = ((i % 2) == 0);
return isEven;
});
当输入参数有多个时
SimpleMath m = new SimpleMath();
m.SetMathHandler((msg, result) =>
{Console.WriteLine("Message: {0}, Result: {1}", msg, result);});
或者显式指定输入参数类型
m.SetMathHandler((string msg, int result) =>
{Console.WriteLine("Message: {0}, Result: {1}", msg, result);});
当没有输入参数时
VerySimpleDelegate d = new VerySimpleDelegate( () => {return "Enjoy your string!";} );
Posted on 2008-02-18 09:56 sunrack 阅读(136) 评论(0) 编辑 收藏 网摘 所属分类: LINQ
Lambda表达式可以在使用代理和匿名代理的地方
1、命名函数
public class Common
{
public delegate bool IntFilter(int i);
public static int[] FilterArrayOfInts(int[] ints, IntFilter filter)
{
ArrayList aList = new ArrayList();
foreach (int i in ints)
{
if (filter(i))
{
aList.Add(i);
}
}
return ((int[])aList.ToArray(typeof(int)));
}
}
public class Application
{
public static bool IsOdd(int i)
{
return ((i & 1) == 1);
}
}
using System.Collections;
int[] nums = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int[] oddNums = Common.FilterArrayOfInts(nums, Application.IsOdd);
foreach (int i in oddNums)
Console.WriteLine(i);
2、匿名函数
int[] nums = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int[] oddNums =
Common.FilterArrayOfInts(nums, delegate(int i) { return ((i & 1) == 1); });
foreach (int i in oddNums)
Console.WriteLine(i);
3、Lambda表达式
int[] nums = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int[] oddNums = Common.FilterArrayOfInts(nums, i => ((i & 1) == 1));
foreach (int i in oddNums)
Console.WriteLine(i);
三种方式的比较
int[] oddNums = // using named method
Common.FilterArrayOfInts(nums, Application.IsOdd);
int[] oddNums = // using anonymous method
Common.FilterArrayOfInts(nums, delegate(int i){return((i & 1) == 1);});
int[] oddNums = // using lambda expression
Common.FilterArrayOfInts(nums, i => ((i & 1) == 1));
命名函数虽然简短,但是而外还要定义处理函数,优点是可以重用
学关于Lambda表达式的一二三
我是白痴,所以我不知道什么是lambda,看了网上N篇文章仍然还是个白痴。还不知道。于是。
给自己写篇自己能看得懂的文章,希望以后再白痴的时候看看就不白痴了。
委托
委托是一种引用方法的类型。一旦为委托分配了方法,委托将与该方法具有完全相同的行为。委托方法的使用可以像其他任何方法一样,具有参数和返回值。
委托就是给一个函数起一个别的名字。
using System;
// Declare delegate -- defines required signature:
delegate void SampleDelegate(string message);
class MainClass
{
// Regular method that matches signature:
static void SampleDelegateMethod(string message)
{
Console.WriteLine(message);
}
static void Main()
{
// Instantiate delegate with named method:
SampleDelegate d1 = SampleDelegateMethod;
// Instantiate delegate with anonymous method:
SampleDelegate d2 = delegate(string message)
{
Console.WriteLine(message);
};
d1("Hello");
d2("World,http://www.dc9.cn/");
}
}
这玩意也是委托
public Form1()
{
InitializeComponent();
this.Click += this.MultiHandler;
}
private void MultiHandler(object sender, System.EventArgs e)
{
MessageBox.Show(((MouseEventArgs)e).Location.ToString());
}
匿名方法
匿名方法就是没名儿的委托。虽然没名,但是必须加”delegate“来表示我没名。
// Create a delegate instance
delegate void Del(int x);
// Instantiate the delegate using an anonymous method
Del d = delegate(int k) { /* ... */ };
Del d = delegate() { System.Console.WriteLine("Copy #:{0}", ++n); };
delegate void Printer(string s);
Printer p = delegate(string j)
{
System.Console.WriteLine(j);
};
p("The delegate using the anonymous method is called.");
delegate string Printer(string s);
private void button1_Click(object sender, EventArgs e)
{
Printer p =delegate(string j)
{
return (j)+"
烦死了";
};
Console.WriteLine(p("The delegate using the anonymous method is called."));
}
或者有名。。。。的匿名委托。。。。哈哈,我在抽风还是微软在抽风。让我死吧。
delegate void Printer(string s);
private void button1_Click(object sender, EventArgs e)
{
Printer p = new Printer(Form1.DoWork);
p("http://www.dc9.cn/");
}
static void DoWork(string k)
{
System.Console.WriteLine(k);
}
匿名方法就完了。
Lamdba 就是 (int x)=>{x+1}就是这样的。。。例子呢就是上面写了的一段用lambda就这样写
delegate string Printer(string s);
private void button1_Click(object sender, EventArgs e)
{
Printer p = j => j+"
烦死了!!!";
Console.WriteLine(p("The delegate using the anonymous method is called."));
}
还能这么用
和上面那个一样,就是简化了。
public Form1()
{
InitializeComponent();
this.Click += (s, e) => { MessageBox.Show(((MouseEventArgs)e).Location.ToString());};
}
超难+===>?其实不难。难的是转换成一般的函数怎么写呢????
。。。。。
Where是Enumerable的一个方法。3.5才有的。里面的参数是Func<(Of <(T, TResult>)>) 泛型委托
Func<(Of <(T, TResult>)>) 泛型委托
using System;
delegate string ConvertMethod(string inString);
public class DelegateExample
{
public static void Main()
{
// Instantiate delegate to reference UppercaseString method
ConvertMethod convertMeth = UppercaseString;
string name = "Dakota";
// Use delegate instance to call UppercaseString method
Console.WriteLine(convertMeth(name));
}
private static string UppercaseString(string inputString)
{
return inputString.ToUpper();
}
}
写成泛型委托是
using System;
public class GenericFunc
{
public static void Main()
{
// Instantiate delegate to reference UppercaseString method
Func<string, string> convertMethod = UppercaseString;
string name = "Dakota";
// Use delegate instance to call UppercaseString method
Console.WriteLine(convertMethod(name));
}
private static string UppercaseString(string inputString)
{
return inputString.ToUpper();
}
}
于是应用到Linq,再变换到lambda
delegate bool TestFunc(string fruit);
private void button1_Click(object sender, EventArgs e)
{
List<string> fruits =new List<string> { "apple", "http://www.dc9.cn", "banana", "mango",
"orange", "blueberry", "grape", "strawberry" };
TestFunc f = new TestFunc(DoWork);
Func<string, bool> f2 = DoWork;
IEnumerable<string> query = fruits.Where(f2);
foreach (string fruit in query)
Console.WriteLine(fruit);
}
private static bool DoWork(string k)
{
return k.Length < 6;
}
能用。
delegate bool TestFunc(string fruit);
private void button1_Click(object sender, EventArgs e)
{
List<string> fruits =new List<string> { "apple", "passionfruit", "banana", "mango",
"orange", "blueberry", "grape", "http://www.dc9.cn" };
TestFunc f = DoWork;
Func<string, bool> f2 =k=> k.Length < 6;
IEnumerable<string> query = fruits.Where(f2);
foreach (string fruit in query)
Console.WriteLine(fruit);
}
private static bool DoWork(string k)
{
return k.Length < 6;
}
也能用
private void button1_Click(object sender, EventArgs e)
{
List<string> fruits =new List<string> { "apple", "passionfruit", "banana", "mango",
"orange", "blueberry", "grape", "http://www.dc9.cn" };
IEnumerable<string> query = fruits.Where(
delegate(string k){
return k.Length < 6;
}
);
foreach (string fruit in query)
Console.WriteLine(fruit);
}
能用~
private void button1_Click(object sender, EventArgs e)
{
List<string> fruits =new List<string> { "apple", "passionfruit", "banana", "mango",
"orange", "blueberry", "grape", "http://www.dc9.cn" };
IEnumerable<string> query = fruits.Where(k=>k.Length<6);
foreach (string fruit in query)
Console.WriteLine(fruit);
}
最后,lambda,能用~~~~就酱紫了~~~~
于是,我这个白痴,被自己写的教程搞明白了。
于是我也会了。
但是心情依旧很糟糕。
。。。。。。。。。
~~~~~~~~~
啊啊啊啊啊~最后来个有意思的!!
public delegate int mydg(int a, int b);
public static class LambdaTest
{
public static int oper(this int a, int b, mydg dg)
{
return dg(a, b);
}
}
Console.WriteLine(1.oper(2, (a, b) => a + b));
Console.WriteLine(2.oper(1, (a, b) => a - b));