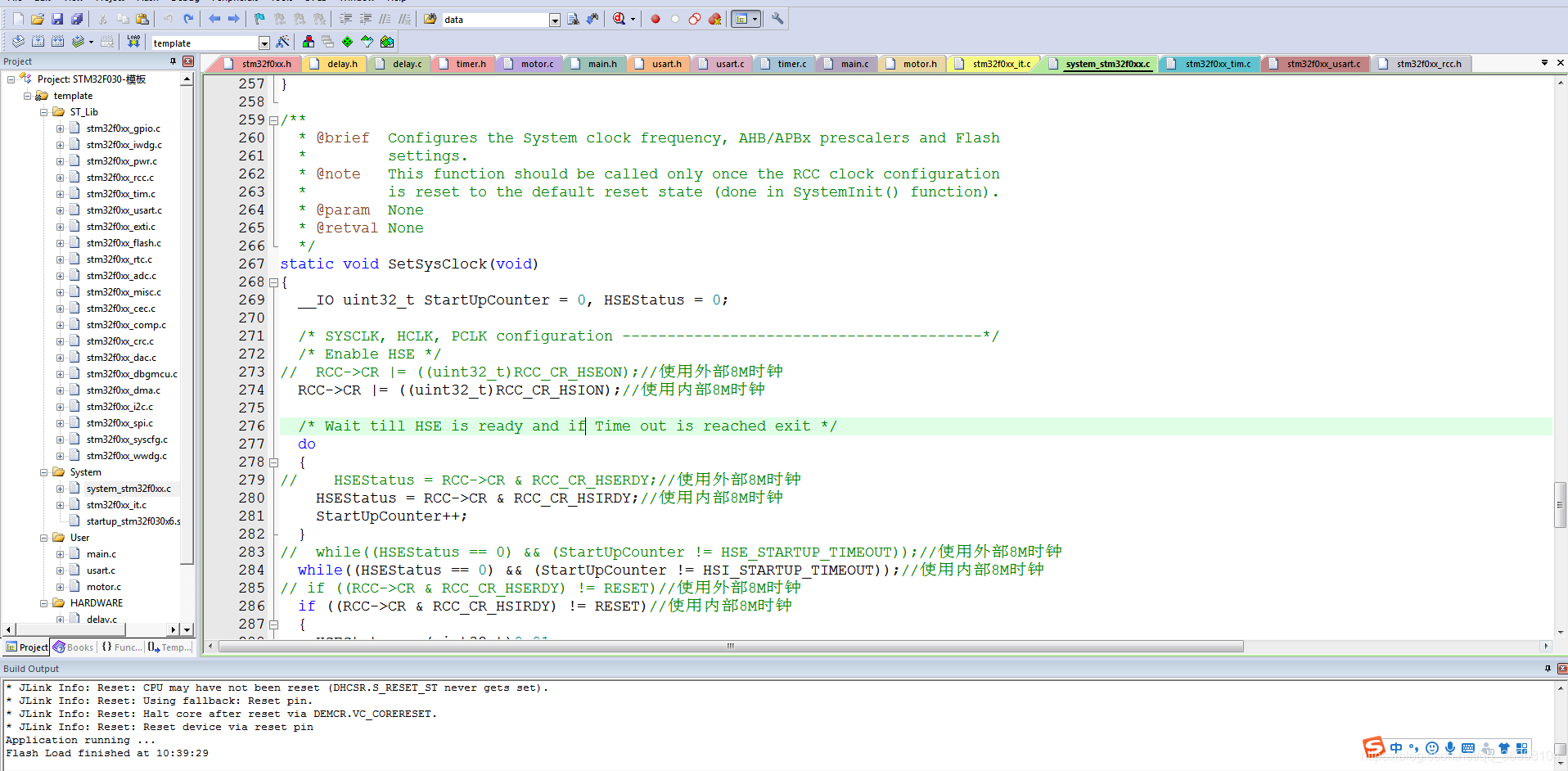
static void SetSysClock(void)
{
__IO uint32_t StartUpCounter = 0, HSEStatus = 0;
/* SYSCLK, HCLK, PCLK configuration ----------------------------------------*/
/* Enable HSE */
// RCC->CR |= ((uint32_t)RCC_CR_HSEON);//使用外部8M时钟
RCC->CR |= ((uint32_t)RCC_CR_HSION);//使用内部8M时钟
/* Wait till HSE is ready and if Time out is reached exit */
do
{
// HSEStatus = RCC->CR & RCC_CR_HSERDY;//使用外部8M时钟
HSEStatus = RCC->CR & RCC_CR_HSIRDY;//使用内部8M时钟
StartUpCounter++;
}
// while((HSEStatus == 0) && (StartUpCounter != HSE_STARTUP_TIMEOUT));//使用外部8M时钟
while((HSEStatus == 0) && (StartUpCounter != HSI_STARTUP_TIMEOUT));//使用内部8M时钟
// if ((RCC->CR & RCC_CR_HSERDY) != RESET)//使用外部8M时钟
if ((RCC->CR & RCC_CR_HSIRDY) != RESET)//使用内部8M时钟
{
HSEStatus = (uint32_t)0x01;
}
else
{
HSEStatus = (uint32_t)0x00;
}
if (HSEStatus == (uint32_t)0x01)
{
/* Enable Prefetch Buffer */
FLASH->ACR |= FLASH_ACR_PRFTBE;
FLASH->ACR |= (uint32_t)FLASH_ACR_LATENCY;
/* HCLK = SYSCLK */
RCC->CFGR |= (uint32_t)RCC_CFGR_HPRE_DIV1;
/* PCLK = HCLK */
RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE_DIV1;
/* PLL configuration: = HSE * 6 = 48 MHz */
// RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_PLLSRC | RCC_CFGR_PLLXTPRE | RCC_CFGR_PLLMULL));//使用外部8M时钟
// RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLSRC_PREDIV1 | RCC_CFGR_PLLXTPRE_PREDIV1 | RCC_CFGR_PLLMULL6);//使用外部8M时钟
// RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLSRC_PREDIV1|RCC_CFGR_PLLXTPRE_PREDIV1_Div2 | RCC_CFGR_PLLMULL9);//内外都不使用
RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLSRC_HSI_Div2|RCC_CFGR_PLLXTPRE_PREDIV1|RCC_CFGR_PLLMULL9);//使用内部8M时钟
/* Enable PLL */
RCC->CR |= RCC_CR_PLLON;
/* Wait till PLL is ready */
while((RCC->CR & RCC_CR_PLLRDY) == 0)
{
}
/* Select PLL as system clock source */
RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_SW));
RCC->CFGR |= (uint32_t)RCC_CFGR_SW_PLL;
/* Wait till PLL is used as system clock source */
while ((RCC->CFGR & (uint32_t)RCC_CFGR_SWS) != (uint32_t)0x08)
{
}
}
else
{ /* If HSE fails to start-up, the application will have wrong clock
configuration. User can add here some code to deal with this error */
}
}
/******************************************************************************/
/* */
/* Reset and Clock Control */
/* */
/******************************************************************************/
/******************** Bit definition for RCC_CR register ********************/
#define RCC_CR_HSION ((uint32_t)0x00000001) /*!< Internal High Speed clock enable */
#define RCC_CR_HSIRDY ((uint32_t)0x00000002) /*!< Internal High Speed clock ready flag */
#define RCC_CR_HSITRIM ((uint32_t)0x000000F8) /*!< Internal High Speed clock trimming */
#define RCC_CR_HSICAL ((uint32_t)0x0000FF00) /*!< Internal High Speed clock Calibration */
#define RCC_CR_HSEON ((uint32_t)0x00010000) /*!< External High Speed clock enable */
#define RCC_CR_HSERDY ((uint32_t)0x00020000) /*!< External High Speed clock ready flag */
#define RCC_CR_HSEBYP ((uint32_t)0x00040000) /*!< External High Speed clock Bypass */
#define RCC_CR_CSSON ((uint32_t)0x00080000) /*!< Clock Security System enable */
#define RCC_CR_PLLON ((uint32_t)0x01000000) /*!< PLL enable */
#define RCC_CR_PLLRDY ((uint32_t)0x02000000) /*!< PLL clock ready flag */
/******************* Bit definition for RCC_CFGR register *******************/
/*!< SW configuration */
#define RCC_CFGR_SW ((uint32_t)0x00000003) /*!< SW[1:0] bits (System clock Switch) */
#define RCC_CFGR_SW_0 ((uint32_t)0x00000001) /*!< Bit 0 */
#define RCC_CFGR_SW_1 ((uint32_t)0x00000002) /*!< Bit 1 */
#define RCC_CFGR_SW_HSI ((uint32_t)0x00000000) /*!< HSI selected as system clock */
#define RCC_CFGR_SW_HSE ((uint32_t)0x00000001) /*!< HSE selected as system clock */
#define RCC_CFGR_SW_PLL ((uint32_t)0x00000002) /*!< PLL selected as system clock */
/*!< SWS configuration */
#define RCC_CFGR_SWS ((uint32_t)0x0000000C) /*!< SWS[1:0] bits (System Clock Switch Status) */
#define RCC_CFGR_SWS_0 ((uint32_t)0x00000004) /*!< Bit 0 */
#define RCC_CFGR_SWS_1 ((uint32_t)0x00000008) /*!< Bit 1 */
#define RCC_CFGR_SWS_HSI ((uint32_t)0x00000000) /*!< HSI oscillator used as system clock */
#define RCC_CFGR_SWS_HSE ((uint32_t)0x00000004) /*!< HSE oscillator used as system clock */
#define RCC_CFGR_SWS_PLL ((uint32_t)0x00000008) /*!< PLL used as system clock */