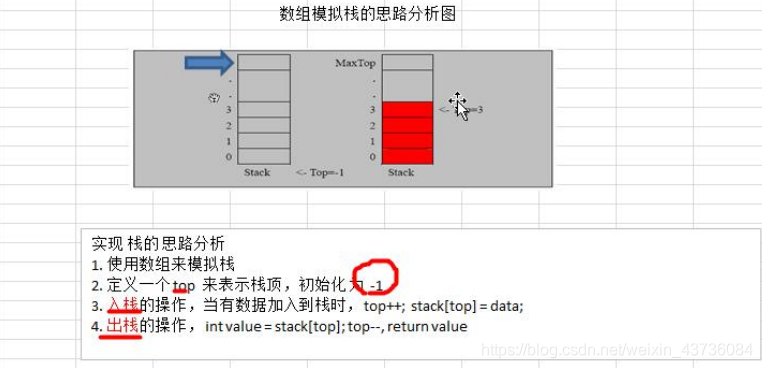
数组模拟栈操作
package stack;
import java.util.Scanner;
public class ArrayStackDemo {
public static void main(String[] args) {
ArrayStack arrayStack = new ArrayStack(4);
String key = "";
boolean loop = true;
Scanner sc = new Scanner(System.in);
while (loop){
System.out.println("show:表示显示栈");
System.out.println("exit:退出程序");
System.out.println("push:表示入栈");
System.out.println("pop:表示出栈");
key = sc.next();
switch (key){
case "show":
arrayStack.list();
break;
case "push":
System.out.println("请输入一个数");
int value = sc.nextInt();
arrayStack.push(value);
break;
case "pop":
try{
System.out.println("出栈的数据是:"+arrayStack.pop());
}catch (Exception e){
System.out.println(e.getMessage());
}
break;
case "exit":
sc.close();
loop = false;
break;
}
}
System.out.println("程序退出");
}
}
class ArrayStack{
private int maxSize;
private int[] stack;
private int top = -1;
public ArrayStack(int maxSize) {
this.maxSize = maxSize;
stack = new int[this.maxSize];
}
public boolean isFull(){
return top == maxSize - 1;
}
public boolean isEmpty(){
return top == -1;
}
public void push(int value){
if (isFull()){
System.out.println("栈满,不能入栈");
return;
}
top++;
stack[top] = value;
}
public int pop(){
if (isEmpty()){
throw new RuntimeException("栈空");
}
int value = stack[top];
top--;
return stack[value];
}
public void list(){
if (isEmpty()){
System.out.println("栈空");
}
for (int i = top; i>=0; i--){
System.out.printf("stack[%d] = %d\n",i,stack[i]);
}
}
}