1.代码
#include<iostream>
#include<vector>
using namespace std;
namespace zz {
template<class T>
struct Node
{
Node(const T& data = T())
:_pNext(nullptr)
, _pPre(nullptr)
, _data(data)
{}
T _data;
Node<T> *_pNext;
Node<T> *_pPre;
};
template<class T>
class list_iterator
{
typedef Node<T> Node;
typedef list_iterator<T> self;
public:
list_iterator(Node *ptr)
:_ptr(ptr)
{}
T& operator *()
{
return _ptr->_data;
}
T* operator ->()
{
return &(_ptr->_data);
}
self& operator ++()
{
_ptr = _ptr->_pNext;
return *this;
}
self operator ++(T)
{
self tmp(*this);
//self tmp(_ptr);
_ptr = _ptr->_pNext;
return tmp;
}
self& operator --()
{
_ptr = _ptr->_pPre;
return *this;
}
self operator --(T)
{
self tmp(_ptr);
_ptr = _ptr->_pPre;
return tmp;
}
bool operator !=(const self& it)
{
return _ptr != it._ptr;
}
bool operator ==(const self& it)
{
return _ptr == it._ptr;
}
Node* _ptr;
};
template<class iterator, class T>
struct list_riterator
{
typedef Node<T> Node;
typedef list_riterator<iterator, T> self;
list_riterator(Node *ptr)
:_it(ptr)
{}
T& operator *()
{
auto it = _it;
--it;
return *it;
}
T* operator ->()
{
return &(operator *());
}
self& operator ++()
{
--_it;
return *this;
}
self operator ++(T)
{
self tmp(_it._ptr);
--_it;
return tmp;
}
self& operator --()
{
++_it;
return *this;
}
self operator --(T)
{
self tmp(_it._ptr);
++_it;
return tmp;
}
bool operator !=(self ptr)
{
return _it != ptr._it;
}
bool operator ==(self ptr)
{
return _it == ptr._it;
}
iterator _it;
};
template<class T>
class list
{
typedef Node<T> Node;
typedef list_iterator<T> iterator;
typedef const list_iterator<T> const_iterator;
typedef list_riterator<iterator, T> riterator;
public:
list()
{
CreateHead();
}
list(int n, const T& data)
{
CreateHead();
for (int i = 0; i < n; i++)
push_back(data);
}
template<class Iterator>
list(Iterator first, Iterator last)
{
CreateHead();
while (first != last)
{
push_back(*first);
++first;
}
}
list(const list<T>& L)
{
CreateHead();
iterator it = L.begin();
while (it != L.end())
{
push_back(*it);
++it;
}
}
list<T>& operator=(const list<T>& L)
{
if (this != &L)
{
clear();
auto it = L.begin();
while (it != L.end())
{
push_back(*it);
++it;
}
}
return *this;
}
~list()
{
clear();
delete _pHead;
}
iterator begin()
{
return iterator(_pHead->_pNext);
}
iterator begin()const
{
return iterator(_pHead->_pNext);
}
iterator end()
{
return iterator(_pHead);
}
iterator end()const
{
return iterator(_pHead);
}
riterator rbegin()
{
return riterator(_pHead);
}
riterator rend()
{
return riterator(_pHead->_pNext);
}
size_t size()const
{
size_t count = 0;
auto it = begin();
while (it != end())
{
++it;
++count;
}
return count;
}
bool empty()const
{
return _pHead->_pNext == _pHead;
}
void resize(int n, const T& data = T())
{
int oldsize = size();
if (n > oldsize)
{
for (int i = oldsize; i < n; i++)
push_back(data);
}
else
{
for (int i = n; i < oldsize; i++)
pop_back();
}
}
T& front()
{
return _pHead->_pNext->_data;
}
const T& front()const
{
return _pHead->_pNext->_data;
}
T& back()
{
return _pHead->_pPre->_data;
}
const T& back()const
{
return _pHead->_pPre->_data;
}
void push_back(const T& data)
{
insert(end(), data);
}
void clear()
{
Node* it = _pHead->_pNext;
while (it != _pHead)
{
_pHead->_pNext = it->_pNext;
delete it;
it = _pHead->_pNext;
}
_pHead->_pNext = _pHead;
_pHead->_pPre = _pHead;
}
void pop_back()
{
erase(--end());
}
iterator insert(iterator pos, const T& data)
{
Node *node = pos._ptr;
Node *pt = new Node(data);
pt->_pPre = node->_pPre;
pt->_pNext = node;
node->_pPre = pt;
pt->_pPre->_pNext = pt;
return iterator(pt);
}
iterator erase(iterator pos)
{
Node *pt = pos._ptr;
if (pt == _pHead)
return end();
Node *ret = pt->_pNext;
pt->_pPre->_pNext = pt->_pNext;
pt->_pNext->_pPre = pt->_pPre;
delete pt;
return iterator(ret);
}
void push_front(const T& data)
{
insert(begin(), data);
}
void pop_front()
{
erase(begin());
}
void swap(list<T> L)
{
swap(_pHead, L._pHead);
}
private:
void CreateHead()
{
_pHead = new Node();
_pHead->_pNext = _pHead;
_pHead->_pPre = _pHead;
_pHead->_data = NULL;
}
protected:
Node* _pHead;
};
}
void test() {
zz::list<int> l1;
zz::list<int> l2(10, 5);
vector <int> v{ 1,2,3,4,5,6,7,8,9,0 };
l2.push_back(9);
zz::list<int> l3(v.begin(), v.end());
zz::list<int> l4(l2);
auto it = l2.begin();
while (it != l2.end()) {
cout << *it << " ";
it++;
}
cout << endl;
for (auto e : l3)
cout << e << " ";
cout << endl;
}
int main() {
test();
return 0;
}
二.截图实现
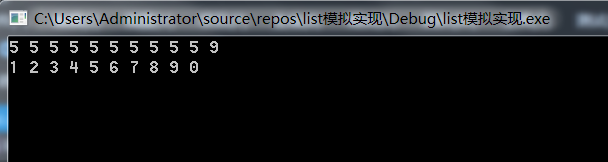