09_1_线程的基本概念
1. 线程的基本概念
线程的一个程序内部的顺序控制流。
线程和进程的区别
每个进程都有独立的代码和数据空间(进程上下文),进程间的切换会有较大的开销。
线程可以看成是轻量级的进程,同一类线程共享代码和数据空间,每个线程有独立的运行栈和程序计数器(PC),线程切换的开销小。
多进程:在程序系统中同时运行多个任务(程序)。
多线程:在同一个应用程序中有多个顺序流同时执行
Java的线程是通过java.lang.Thread类来实现的。
VM启动时会有一个自主方法(public static void main() {})所定义的线程。
可以通过创建Thread的实例来创建新的线程。
每个线程都是通过某个特定Thread对象所对应的方法run()来完成其操作的,方法run()称为线程体。
通过调用Thread类的start()方法来启动一个线程。
扫描二维码关注公众号,回复:
83307 查看本文章
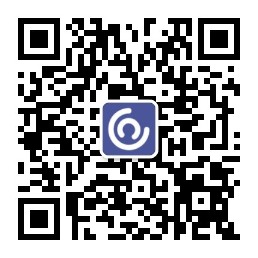
1.1实现线程的两种方式之一
TestThread1
package Test; public class TestThread1 { public static void main(String[] args) { Runner1 t = new Runner1(); new Thread(t).start(); for (int i = 0; i < 1000; i++) { System.out.println("Main Thread" + i); } } } class Runner1 implements Runnable { @Override public void run() { for (int i = 0; i < 1000; i++) { System.out.println("Runner1 Thread" + i); } } }
1.2实现线程的两种方式之一
TestThread2
package Test; public class TestThread2 { public static void main(String[] args) { Runner2 t = new Runner2(); t.start(); for (int i = 0; i < 1000; i++) { System.out.println("Main Thread" + i); } } } class Runner2 extends Thread { @Override public void run() { for (int i = 0; i < 1000; i++) { System.out.println("Runner1 Thread" + i); } } }
2. sleep方法
sleep方法
可以调用Thread的静态方法:
public static void sleep(long millis) throws InterruptedException
使得当前线程休眠(暂时停止执行millis毫秒)
由于是静态方法,sleep可以由类名直接调用:
Thread.sleep()
例子:
package Test; import java.util.Date; public class TestThread3 { public static void main(String[] args) { Runner3 t = new Runner3(); t.start(); try { Thread.sleep(10000); } catch (InterruptedException e) { } t.interrupt(); } } class Runner3 extends Thread { @Override public void run() { for (int i = 0; i < 1000; i++) { try { Thread.sleep(1000); } catch (InterruptedException e) { return; } System.out.println("-------------" + new Date() + "---------------------"); } } }
3. 生产者消费者问题
public class ProducerConsumer { public static void main(String[] args) { SyncStack ss = new SyncStack(); Producer p = new Producer(ss); Consumer c = new Consumer(ss); new Thread(p).start(); new Thread(p).start(); new Thread(p).start(); new Thread(c).start(); } } class WoTou { int id; WoTou(int id) { this.id = id; } public String toString() { return "WoTou : " + id; } } class SyncStack { int index = 0; WoTou[] arrWT = new WoTou[6]; public synchronized void push(WoTou wt) { while(index == arrWT.length) { try { this.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } this.notifyAll(); arrWT[index] = wt; index ++; } public synchronized WoTou pop() { while(index == 0) { try { this.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } this.notifyAll(); index--; return arrWT[index]; } } class Producer implements Runnable { SyncStack ss = null; Producer(SyncStack ss) { this.ss = ss; } public void run() { for(int i=0; i<20; i++) { WoTou wt = new WoTou(i); ss.push(wt); System.out.println("生产了:" + wt); try { Thread.sleep((int)(Math.random() * 200)); } catch (InterruptedException e) { e.printStackTrace(); } } } } class Consumer implements Runnable { SyncStack ss = null; Consumer(SyncStack ss) { this.ss = ss; } public void run() { for(int i=0; i<20; i++) { WoTou wt = ss.pop(); System.out.println("消费了: " + wt); try { Thread.sleep((int)(Math.random() * 1000)); } catch (InterruptedException e) { e.printStackTrace(); } } } }