package operator;
public class Demo04 {
public static void main(String[] args) {
// ++(自增) --(自减) 一元运算符
int a = 3;
int b = a++; //执行完这行代码后,先给b赋值,然后自增
// a++ 相当于 a = a + 1
System.out.println(a);
int c = ++a; //执行完这行代码前,先自增,再给c赋值
// ++a 相当于 a = a + 1
System.out.println(a);
System.out.println(b);
System.out.println(c);
//扩充:幂运算 2^3 = 2*2*2 = 8
double pow = Math.pow(2, 3);
System.out.println(pow);
}
}
package operator;
//逻辑运算符
public class Demo05 {
public static void main(String[] args) {
// 与 (and) 或 (or) 非(取反)
boolean a = true;
boolean b = false;
System.out.println("a&&b:"+(a&&b)); //逻辑与运算:两个变量都为真,结果才为true
System.out.println("a||b:"+(a||b)); //逻辑或运算:两个变量有一个为真,则结果才为true
System.out.println("!(a&&b:)"+!(a&&b));//如果是真则为假,如果是假则为真
//短路运算
int c = 5;
boolean d = (c<4)&&(c++<4);
System.out.println(d);
System.out.println(c);
}
}
package operator;
//位运算
public class Demo06 {
public static void main(String[] args) {
/*
A = 0011 1100
B = 0000 1101
A&B = 0000 1100 A与B:都是0为0,0与1为0
A|B = 0011 1101 A或B:都是0为0,0或1为1
A^B = 0011 0001 A亦或B(取反):两个位置相同则为0,否则为1
~B = 1111 0010 B取反
2*8 = 16 2*2*2*2
位运算效率极高!!!
<<:左移 相当于 *2
>>:右移 相当于 /2
0000 0000 0
0000 0001 1
0000 0010 2
0000 0011 3
0000 0100 4
0000 0101 5
0000 0110 6
0000 0111 7
0000 1000 8
0001 0000 16
*/
System.out.println(2<<3);
}
}
package operator;
public class Demo07 {
public static void main(String[] args) {
int a = 10;
int b = 20;
a+=b; //a=a+b
a-=b; //a=a-b
System.out.println(a);
/*字符串连接符 + ;
如果在+号字符串中出现了String字符串类型,
它会自动把字符串都换成String然后进行连接
*/
System.out.println(a+b);
System.out.println(""+a+b);
System.out.println(a+b+"");
}
}
package operator;
//三元运算符
public class Demo08 {
public static void main(String[] args) {
// x ? y: z
//如果X==true:则结果为y,否则结果为z
int score = 50;
String type = score <60 ?"不及格":"及格";
System.out.println(type);
}
}
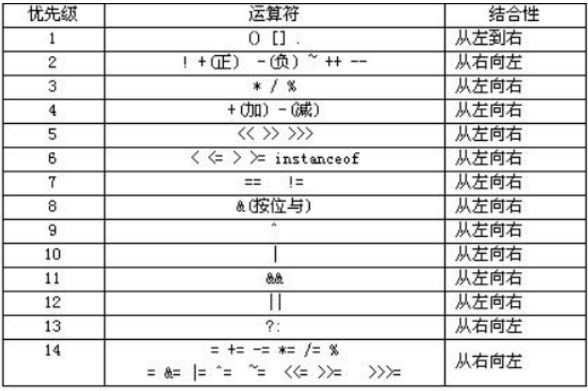