上一篇搭建了一个OAuth2认证服务器,可以生成token,这篇来改造下之前的订单微服务,使其能够认这个token令牌。
本篇针对订单服务要做三件事:
1,要让他知道自己是资源服务器,他知道这件事后,才会在前边加一个过滤器去验令牌(配置@EnableResourceServer 配置类)
2,要让他知道自己是什么资源服务器(配置资源服务器ID)
3,配置去哪里验令牌,怎么验令牌,要带什么信息去验 (配置@EnableWebSecurity 配置TokenServices,配置AuthenticationManager)
搭建资源服务器
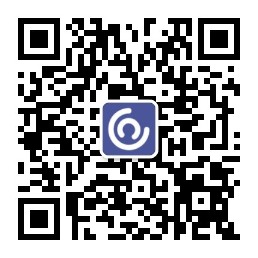
++++++++++++++++++资源服务器现有的各个类++++++++++++++++++++++++++++
pom.xml:

<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.nb.security</groupId> <artifactId>nb-order-api</artifactId> <version>0.0.1-SNAPSHOT</version> <properties> <java.version>1.8</java.version> </properties> <dependencyManagement> <dependencies> <dependency> <!-- Import dependency management from Spring Boot --> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>2.1.6.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> <!--spring cloud--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Greenwich.SR2</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!--OAuth2--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-oauth2</artifactId> </dependency> <!--lombok--> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> </dependency> </dependencies> <build> <plugins> <!--指定JDK编译版本 --> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> <encoding>UTF-8</encoding> </configuration> </plugin> <!-- 打包跳过测试 --> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <configuration> <skipTests>true</skipTests> </configuration> </plugin> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
application.yml:

server:
port: 9060
OrderInfo.java :

package com.nb.security.order; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Data @NoArgsConstructor @AllArgsConstructor public class OrderInfo { private Long productId; }
OrderController:

package com.nb.security.order; import lombok.extern.slf4j.Slf4j; import org.springframework.web.bind.annotation.*; import org.springframework.web.client.RestTemplate; @Slf4j @RestController @RequestMapping("/orders") public class OrderController { private RestTemplate restTemplate = new RestTemplate(); @PostMapping public OrderInfo create(@RequestBody OrderInfo info){ //查询价格 // PriceInfo price = restTemplate.getForObject("http://localhost:9080/prices/"+info.getProductId(),PriceInfo.class); // log.info("price is "+price.getPrice()); return info; } @GetMapping public OrderInfo getInfo(@PathVariable Long id){ log.info("getInfo: id is "+id); return new OrderInfo(id); } }
启动类:

package com.nb.security; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class NbOrderApiApplication { public static void main(String[] args) { SpringApplication.run(NbOrderApiApplication.class, args); } }
PriceInfo.java (暂时先不用):

package com.nb.security.order; import lombok.Data; import java.math.BigDecimal; @Data public class PriceInfo { private Long id; private BigDecimal price; }
++++++++++++++++++资源服务器现有的各个类结束++++++++++++++++++++++++++++
1,在资源服务器pom哩加上 oauth2的依赖:
<!--OAuth2--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-oauth2</artifactId> </dependency>
2,新建资源服务器配置类,继承 ResourceServerConfigurerAdapter
package com.nb.security.resource.server; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer; import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter; import org.springframework.security.oauth2.config.annotation.web.configurers.ResourceServerSecurityConfigurer; /** * 资源服务器 * 配置了@EnableResourceServer ,所有发往nb-order-api的请求,都会去请求头里找token,找不到不让你过 */ @Configuration @EnableResourceServer//告诉nb-order-api,你就是资源服务器 public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter { @Override public void configure(ResourceServerSecurityConfigurer resources) throws Exception { //配置资源服务器的id,“现在我就是资源服务器order-server!!!” resources.resourceId("order-server"); } @Override public void configure(HttpSecurity http) throws Exception { /** * 进入nb-order-api的所有请求,哪些要拦截,哪些要放过,在这里配置 */ http.authorizeRequests() .antMatchers("/hello") .permitAll() //放过/haha不拦截 .anyRequest().authenticated();//其余所有请求都拦截 } }
ResourceServerConfigurerAdapter 有两个方法:
开篇说的1、2、3中的1和2已经完成了,下面做第3件事,怎么验令牌:
新建配置类:
package com.nb.security.resource.server; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager; import org.springframework.security.oauth2.provider.token.RemoteTokenServices; import org.springframework.security.oauth2.provider.token.ResourceServerTokenServices; /** * 怎么验发往本服务的请求头的令牌 * 1,自定义tokenServices ,说明去哪里去验token * 2,重写authenticationManagerBean()方法,将AuthenticationManager暴露为一个Bean * 要认证跟用户相关的信息,一般用 AuthenticationManager * * 这样配置了后,所有发往nb-order-api的请求, * 需要验token的时候就会发请求去http://localhost:9090/oauth/check_token验token,获取到token对应的用户信息 */ @Configuration @EnableWebSecurity public class OAuth2WebSecurityConfig extends WebSecurityConfigurerAdapter{ /** * 通过这个Bean,去远程调用认证服务器,验token * @return */ @Bean public ResourceServerTokenServices tokenServices(){ RemoteTokenServices tokenServices = new RemoteTokenServices(); tokenServices.setClientId("orderService");//在认证服务器配置的,订单服务的clientId tokenServices.setClientSecret("123456");//在认证服务器配置的,订单服务的ClientSecret tokenServices.setCheckTokenEndpointUrl("http://localhost:9090/oauth/check_token"); return tokenServices; } /** * 要认证跟用户相关的信息,一般用 AuthenticationManager * 覆盖这个方法,可以将AuthenticationManager暴露为一个Bean * * @return * @throws Exception */ @Bean @Override public AuthenticationManager authenticationManagerBean() throws Exception { OAuth2AuthenticationManager authenticationManager = new OAuth2AuthenticationManager(); authenticationManager.setTokenServices(tokenServices());//设置为自定义的TokenServices,去校验令牌 return authenticationManager; } }
认证服务器关于订单服务的配置:
启动认证服务器,
启动订单 资源服务器,
访问认证服务器获取token : localhost:9090/oauth/token
拿着 token 去资源服务器创建订单,注意,选择bearer类型的token,生成的请求头是这样的(注:Authorization的value值,postman生成的是以Bearer开头的,但是我看谷歌浏览器restclient插件生成的是bearer的B是小写):
在资源服务器里,可以通过该注解获取用户名:
错误的情况:
如果把资源服务器配置的resourceId改成了order-server222,请求创建订单,会受到如下的错误
如果资源服务器检验token的cilentID获取clientSecret写错了,后台会报错:
++++++++++++++++++++++分割线++++++++++++++++++++++
小结:
接上篇的认证服务器,本篇实现了资源服务器,以及与认证服务器的交互,怎么去验令牌。
遗留疑问:
认证服务器里面,配置资源服务器secret的时候,用passwordEncoder对123456进行了加密,而在资源服务器里,clientSecret确实明文的123456,这两者不需要一致么?
认证服务器里对资源服务器ClientId、 ClientSecret配置:
资源服务器验令牌携带的ClientId、 ClientSecret:
代码github :https://github.com/lhy1234/springcloud-security/tree/chapt-4-5-resource-server