原题链接在这里:https://leetcode.com/problems/lowest-common-ancestor-of-deepest-leaves/
题目:
Given a rooted binary tree, return the lowest common ancestor of its deepest leaves.
Recall that:
- The node of a binary tree is a leaf if and only if it has no children
- The depth of the root of the tree is 0, and if the depth of a node is
d
, the depth of each of its children isd+1
. - The lowest common ancestor of a set
S
of nodes is the nodeA
with the largest depth such that every node in S is in the subtree with rootA
.
Example 1:
Input: root = [1,2,3] Output: [1,2,3] Explanation: The deepest leaves are the nodes with values 2 and 3. The lowest common ancestor of these leaves is the node with value 1. The answer returned is a TreeNode object (not an array) with serialization "[1,2,3]".
Example 2:
Input: root = [1,2,3,4] Output: [4]
Example 3:
Input: root = [1,2,3,4,5] Output: [2,4,5]
Constraints:
- The given tree will have between 1 and 1000 nodes.
- Each node of the tree will have a distinct value between 1 and 1000.
题解:
For dfs, state needs current TreeNode root only. return value needs both depth and lca of deepest leaves node.
扫描二维码关注公众号,回复:
8194936 查看本文章
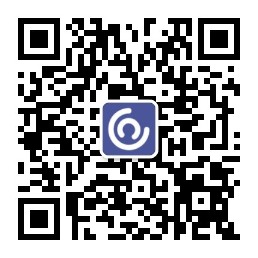
Divide and conquer, have left result and right result first. Then compare the depth, pick the bigger side, if both sides have same depth, return root with depth + 1.
Time Complexity: O(n). n is nodes count of the whole tree.
Space: O(logn).
AC Java:
1 /** 2 * Definition for a binary tree node. 3 * public class TreeNode { 4 * int val; 5 * TreeNode left; 6 * TreeNode right; 7 * TreeNode(int x) { val = x; } 8 * } 9 */ 10 class Solution { 11 public TreeNode lcaDeepestLeaves(TreeNode root) { 12 if(root == null){ 13 return root; 14 } 15 16 return lcaDfs(root).node; 17 } 18 19 private Pair lcaDfs(TreeNode root){ 20 if(root == null){ 21 return new Pair(root, 0); 22 } 23 24 Pair l = lcaDfs(root.left); 25 Pair r = lcaDfs(root.right); 26 if(l.depth > r.depth){ 27 return new Pair(l.node, l.depth + 1); 28 }else if(l.depth < r.depth){ 29 return new Pair(r.node, r.depth + 1); 30 }else{ 31 return new Pair(root, l.depth + 1); 32 } 33 } 34 } 35 36 class Pair{ 37 TreeNode node; 38 int depth; 39 public Pair(TreeNode node, int depth){ 40 this.node = node; 41 this.depth = depth; 42 } 43 }