题目如下:
Given a rooted binary tree, return the lowest common ancestor of its deepest leaves.
Recall that:
- The node of a binary tree is a leaf if and only if it has no children
- The depth of the root of the tree is 0, and if the depth of a node is
d
, the depth of each of its children isd+1
.- The lowest common ancestor of a set
S
of nodes is the nodeA
with the largest depth such that every node in S is in the subtree with rootA
.Example 1:
Input: root = [1,2,3] Output: [1,2,3] Explanation: The deepest leaves are the nodes with values 2 and 3. The lowest common ancestor of these leaves is the node with value 1. The answer returned is a TreeNode object (not an array) with serialization "[1,2,3]".Example 2:
Input: root = [1,2,3,4] Output: [4]Example 3:
Input: root = [1,2,3,4,5] Output: [2,4,5]Constraints:
- The given tree will have between 1 and 1000 nodes.
- Each node of the tree will have a distinct value between 1 and 1000.
解题思路:首先求出最深的所有叶子节点的索引,存入列表中;然后把索引列表排序,取出最大的索引值记为val,显然其父节点的索引值是(val - val%2)/2,把父节点的索引值加入索引列表,并重复这个过程,知道索引列表中所有的值都相等为止,这个值就是所有最深叶子的节点的公共父节点。得到公共父节点索引值,可以反推出从根节点到该节点的遍历路径,从而可以求出具体的节点。
代码如下:
扫描二维码关注公众号,回复:
6847162 查看本文章
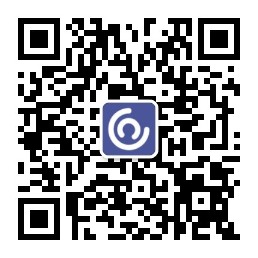
# Definition for a binary tree node. # class TreeNode(object): # def __init__(self, x): # self.val = x # self.left = None # self.right = None class Solution(object): dic = {} maxLevel = 0 def recursive(self,node,level,number): self.maxLevel = max(self.maxLevel,level) self.dic[level] = self.dic.setdefault(level,[]) + [number] if node.left != None: self.recursive(node.left,level+1,number*2) if node.right != None: self.recursive(node.right,level+1,number*2+1) def lcaDeepestLeaves(self, root): """ :type root: TreeNode :rtype: TreeNode """ self.dic = {} self.maxLevel = 0 self.recursive(root,1,1) node_list = sorted(self.dic[self.maxLevel]) def isEqual(node_list): v = node_list[0] for i in node_list: if v != i: return False return True while not isEqual(node_list): node_list.sort(reverse=True) val = node_list.pop(0) val = (val - val%2)/2 node_list.append(val) number = node_list[0] path = [] while number > 1: if number%2 == 0: path = ['L'] + path else: path = ['R'] + path number = (number - number % 2) / 2 node = root while len(path) > 0: p = path.pop(0) if p == 'L':node = node.left else:node = node.right return node