Flutter 中的路由通俗的讲就是页面跳转。在 Flutter 中通过 Navigator 组件管理路由导航。并提供了管理堆栈的方法。如:
Navigator.push 和
Navigator.pop
Flutter 中给我们提供了两种配置路由跳转的方式:1、基本路由 2、命名路由
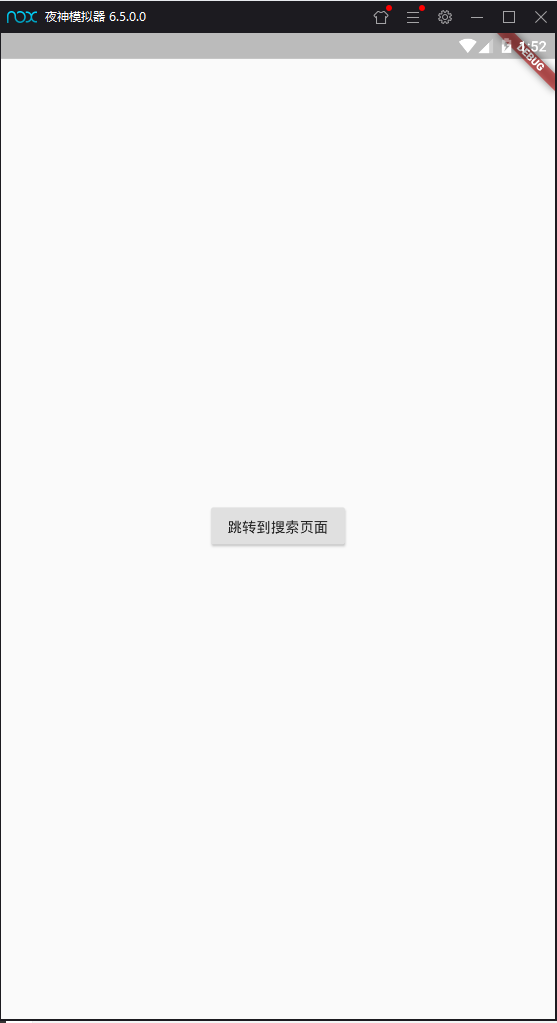
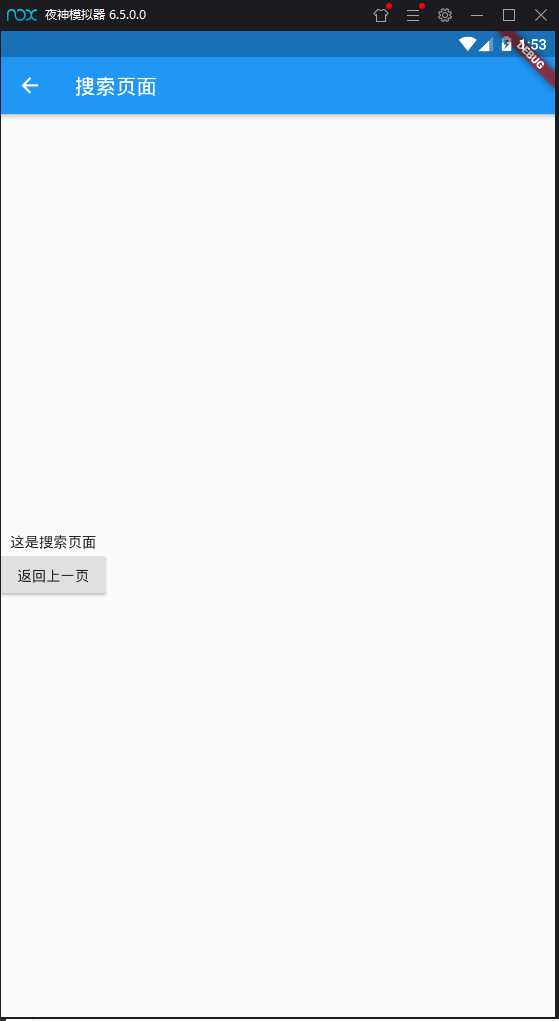
-
基本路由
比如我们现在想从 HomePage 组件跳转到 SearchPage 组件。
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( title: "NavigatorWidget", home: HomePage(), )); } class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: RaisedButton( child: Text("跳转到搜索页面"), onPressed: (){ Navigator.of(context).push( MaterialPageRoute( builder: (context) => SearchPage() ) ); }, ), ) ); } } class SearchPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("搜索页面")), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text("这是搜索页面"), RaisedButton( child: Text("返回上一页"), onPressed: () { Navigator.of(context).pop(); }, ) ], ), ); } }
-
基本路由+传参
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( title: "NavigatorWidget", home: HomePage(), )); } class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: RaisedButton( child: Text("跳转到搜索页面"), onPressed: (){ Navigator.of(context).push( MaterialPageRoute( builder: (context) => SearchPage("我是传参") ) ); }, ), ) ); } } class SearchPage extends StatelessWidget { String param; SearchPage(this.param); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("搜索页面")), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text("这是传参值:$param"), RaisedButton( child: Text("返回上一页"), onPressed: () { Navigator.of(context).pop(); }, ) ], ), ); } }
-
命名路由
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( title: "NavigatorWidget", initialRoute: "/", routes: { "/":(context) => HomePage(), "/search":(context) => SearchPage(), }, )); } class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: RaisedButton( child: Text("跳转到搜索页面"), onPressed: (){ Navigator.of(context).pushNamed("/search"); }, ), ) ); } } class SearchPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("搜索页面")), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text("这是搜索页面"), RaisedButton( child: Text("返回上一页"), onPressed: () { Navigator.of(context).pop(); }, ) ], ), ); } }
-
命名路由+传参(最常用的)