查了些资料,感觉很多码友,尤其是在Core那块写上传文件的代码,个人感觉写复杂了!
所以决定自己来个究竟!
还是先看测试中的效果 如下截图:
1:========MVC (这个就当自己再复习一遍)=========
2:========Core3.0的一些截图========
扫描二维码关注公众号,回复:
7910849 查看本文章
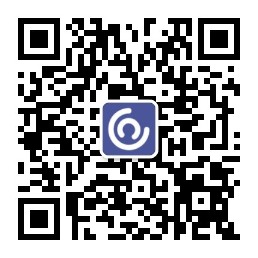
======================
1:Asp.net MVC 简单的文件上传
======================
index.cshtml (3种方法,1:原生html;2:HtmlHelper; 3:ajax非侵入式)

1 @{ 2 Layout = null; 3 } 4 5 <!DOCTYPE html> 6 7 <html> 8 <head> 9 <meta name="viewport" content="width=device-width" /> 10 <title>Index</title> 11 <link href="~/Content/bootstrap.css" rel="stylesheet" /> 12 <script src="~/Scripts/jquery-3.3.1.min.js"></script> 13 <script src="~/Scripts/jquery.unobtrusive-ajax.js"></script> 14 <script type="text/javascript"> 15 function upFileok(data) { 16 //var json = JSON.parse(data); //这里不需要再JSON.parse(),后台就是 jsonresult数据 17 console.log(data); 18 console.log("code=" + data.code + ", msg=" + data.msg) 19 } 20 </script> 21 </head> 22 <body> 23 <div> 24 @*方法一:*@ 25 @*<form method="post" enctype="multipart/form-data" action="/FileUpload/UploadFile"> 26 <input type="file" name="fileup" id="fileup" /><input type="submit" value="点击上传" id="submit" /> 27 </form>*@ 28 29 @*方法二:Microsoft封装的Html帮助类 *@ 30 @*@using (Html.BeginForm("UploadFile", "FileUpload", FormMethod.Post, new { enctype = "multipart/form-data" })) 31 { 32 <table class="table"> 33 <tr><td> @Html.Label("选择要上传的文件")</td><td><input type="file" name="fileup" /></td></tr> 34 <tr><td colspan="2"><input type="submit" value="点击上传" /></td></tr> 35 </table> 36 }*@ 37 38 @*方法三:Microsoft封装的Html帮助类+Ajax异步上传 ,需要Nuget jquery.unobtrusive-ajax.js文件 *@ 39 @using (Ajax.BeginForm("UploadFile", "FileUpload", null, new AjaxOptions() { HttpMethod = "post", OnSuccess = "upFileok" }, new { enctype = "multipart/form-data" })) 40 { 41 <table class="table"> 42 <tr><td> @Html.Label("选择要上传的文件")</td><td><input type="file" name="fileup" /></td></tr> 43 <tr><td colspan="2"><input type="submit" value="点击上传" /></td></tr> 44 </table> 45 } 46 </div> 47 </body> 48 </html>
FileUploadController.cs

1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Web; 5 using System.Web.Mvc; 6 7 namespace WebApplication1.Controllers 8 { 9 public class FileUploadController : Controller 10 { 11 // GET: FileUpload 12 public ActionResult Index() 13 { 14 return View(); 15 } 16 17 public ActionResult UploadFile() 18 { 19 var file = Request.Files; 20 if (file != null&&file.Count>0) 21 { 22 // string basePath = AppDomain.CurrentDomain.BaseDirectory; 23 for (int i = 0; i < file.Count; i++) 24 { 25 bool isimg = file[i].ContentType.Contains("image/"); 26 //---这个可以将原文件名称写入到数据库 27 string filename = System.IO.Path.GetFileName(file[i].FileName);//-- fengge001.jpg 28 29 string fileExstr = System.IO.Path.GetExtension(file[i].FileName);//--.jpg 30 string path = "~/imgs/" + Guid.NewGuid().ToString() + fileExstr; 31 file[i].SaveAs(Server.MapPath(path)); 32 } 33 34 return Json(new { code = 200, msg = "上传成功!" }, JsonRequestBehavior.AllowGet); 35 } 36 else 37 { 38 return Json(new { code = 500, msg = "上传失败!" }, JsonRequestBehavior.AllowGet); 39 } 40 } 41 } 42 }
======================
2: Asp.NetCore 3.0 MVC 简单的文件上传
======================
index.cshtml

1 @{ 2 Layout = null; 3 } 4 5 <!DOCTYPE html> 6 7 <html> 8 <head> 9 <meta name="viewport" content="width=device-width" /> 10 <title>Index</title> 11 12 </head> 13 <body> 14 <form action="/FileUpload/UploadF" method="post" enctype="multipart/form-data"> 15 <input type="file" name="file" /><input type="submit" value="点击上传" /> 16 </form> 17 </body> 18 </html>
FileUploadController.cs

1 using Microsoft.AspNetCore.Mvc; 2 using System; 3 namespace WebApplication.Controllers 4 { 5 using Microsoft.AspNetCore.Hosting; 6 using System.IO; 7 public class FileUploadController : Controller 8 { 9 private IWebHostEnvironment en; 10 public FileUploadController(IWebHostEnvironment en) { this.en = en;} 11 public IActionResult Index() {return View();} 12 public IActionResult UploadF() 13 { 14 var files = Request.Form.Files; 15 string filename = files[0].FileName;//--"360截图20191119113847612.jpg" 16 string fileExtention = System.IO.Path.GetExtension(files[0].FileName);//--.jpg 17 string path = Guid.NewGuid().ToString() + fileExtention; string basepath = en.WebRootPath; 18 //en.WebRootPath-》wwwroot的目录; .ContentRootPath到达WebApplication的项目目录 19 string path_server = basepath + "\\upfile\\" + path; 20 using (FileStream fstream = new FileStream(path_server, FileMode.OpenOrCreate, FileAccess.ReadWrite)) 21 // using (FileStream fstream = System.IO.File.Create(newFile)) //两种都可以使用 22 { 23 files[0].CopyTo(fstream); 24 } 25 return Ok(new { code = 200, msg = "上传成功!" }); 26 //files[0].CopyTo(,); Copies the contents of the uploaded file to the target stream 27 //将上载文件的内容复制到目标流 28 } 29 } 30 }