1、编写SpringBoot的引导类
package springboot_test.springboot_test;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
@SpringBootApplication
public class SpringbootTestApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootTestApplication.class, args);
}
}
2、编写普通实体Dog
package springboot_test.springboot_test.bean;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
//普通实体,会做为Person类中的属性使用,不需要加任何注解
//@ConfigurationProperties(prefix = "person.dog")
//@Component
public class Dog {
private String name;
private String color;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
", color='" + color + '\'' +
'}';
}
}
3、编写需要绑定yml文件中的值的配置类Person
package springboot_test.springboot_test.bean;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.boot.context.properties.NestedConfigurationProperty;
import org.springframework.stereotype.Component;
import java.util.Date;
import java.util.List;
import java.util.Map;
//prefix指定使用yml配置文件中的哪部分开头的数据
@ConfigurationProperties(prefix = "person")
//该类也做为组件才能使用容器中的其他组件,即才能使用@ConfigurationProperties
@Component
public class Person {
private String name;
private String age;
private Date birthDay;
private Map map;
private List list;
//类中含有其他类的对象,想让dog在yml编写的时候也给提示,需要加上该属性
@NestedConfigurationProperty
private Dog dog;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public Date getBirthDay() {
return birthDay;
}
public void setBirthDay(Date birthDay) {
this.birthDay = birthDay;
}
public Map getMap() {
return map;
}
public void setMap(Map map) {
this.map = map;
}
public List getList() {
return list;
}
public void setList(List list) {
this.list = list;
}
public Dog getDog() {
return dog;
}
public void setDog(Dog dog) {
this.dog = dog;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age='" + age + '\'' +
", birthDay=" + birthDay +
", map=" + map +
", list=" + list +
", dog=" + dog +
'}';
}
}
4、配置pom.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!--依赖的父项目,父项目中还会依赖其他父项目来决定使用的jar包的版本-->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>springboot_test</groupId>
<artifactId>springboot_test</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot_test</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<!--用于引进springmvc等相关jar包,可通过页面访问-->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--用于junit测试-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--用于热部署-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
<!--配置处理器,当类上加@ConfigurationProperties注解时,需要配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
<!--用于使用maven的package功能进行打包-->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
5、编写application.yml文件
person:
name: zhangsan
age: 18
birth-day: 2019/11/19
map: {k1: v1,k2: v2}
list:
- pig
- cat
dog:
color: black
name: 小狗
6、编写PersonTest.java测试类
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import springboot_test.springboot_test.SpringbootTestApplication;
import springboot_test.springboot_test.bean.Person;
@SpringBootTest(classes = SpringbootTestApplication.class)
@RunWith(SpringRunner.class)
public class PersonTest {
@Autowired
private Person person;
@Test
public void getPerson(){
System.out.println(person);
}
}
另外在测试的时候出现了报错java.lang.Exception: No runnable methods,原因为@Test注解引入的类不对,应该引入org.junit.Test;
还有一个可能的原因是没有在方法上写@Test注解
另外,如果绑定的值是在application.properties中配置的,而不是在yml中配置的,则会出现打印结果中文乱码的问题,application.properties配置如下:
person.name=张三
person.age=18
person.birth-day= 2019/11/19
person.list=a,b,c
person.map.k1=v1
person.map.k2=v2
person.dog.name=小狗
person.dog.color=black
可通过如下所示
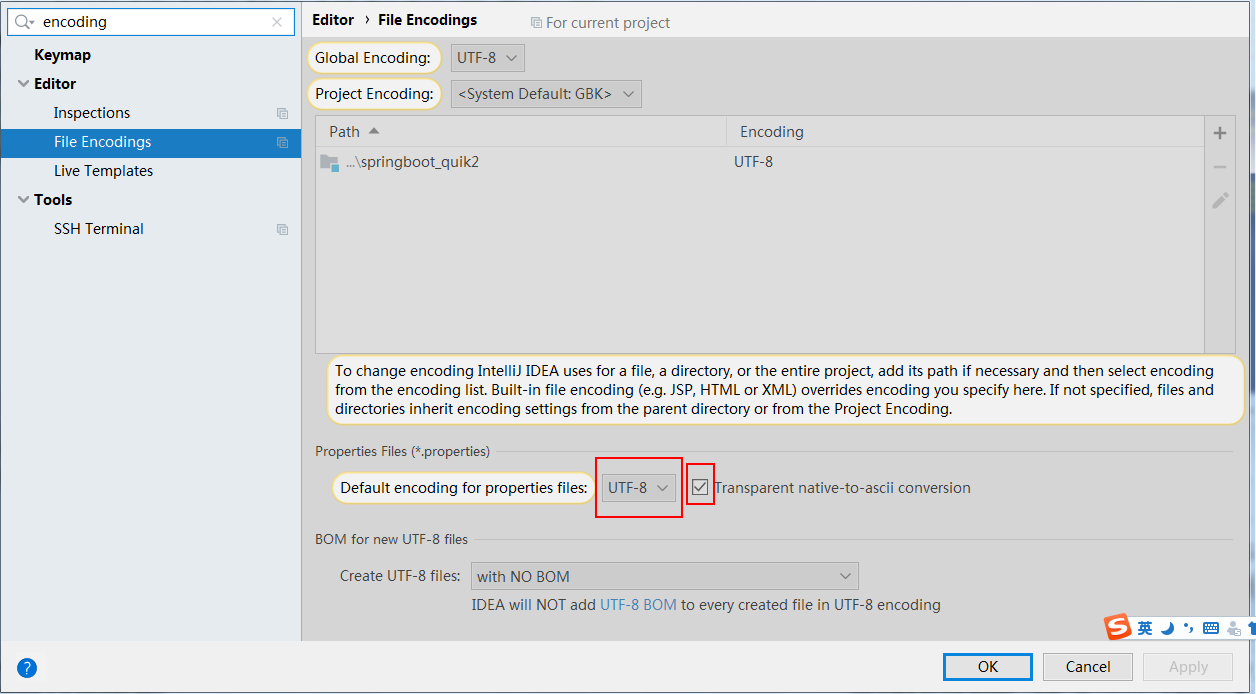
如有理解不到位的地方,望指教!