2019-11-02-23:25:26
目录
1.泛型的概念:
2.泛型的定义和使用:
2.1定义和使用含有泛型的类:
2.2定义和使用含有泛型的方法:
2.3定义和使用含有泛型的接口:
泛型的概念:
泛型是一种未知的数据类型,当我门不知道使用什么数据类型的时候,可以使用泛型,泛型也可以看成是一个变量,用来接受数据类型
E e:Element 元素
T t:Type 类型
扫描二维码关注公众号,回复:
7726248 查看本文章
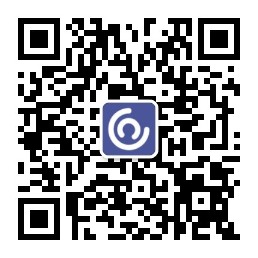
泛型的定义和使用:
我们在集合中会大量使用到泛型,用来灵活地将数据类型应用到不同的类、方法、接口当中,将数据类型作为参数进行传递
定义和使用含有泛型的类:
修饰符 class 类名<代表泛型的变量>{ }
1 package demosummary.generic;
2
3 public class GenericClass<E> {
4 private E name;
5
6 public GenericClass() {
7 }
8
9 public GenericClass(E name) {
10 this.name = name;
11 }
12
13 public E getName() {
14 return name;
15 }
16
17 public void setName(E name) {
18 this.name = name;
19 }
20
21 @Override
22 public String toString() {
23 return "GenericClass{" +
24 "name=" + name +
25 '}';
26 }
27 }
1 package demosummary.generic;
2
3 public class GenericClassTest {
4 public static void main(String[] args) {
5 //不写泛型则默认为Object类
6 GenericClass gc = new GenericClass();
7 gc.setName("默认为Object类");
8 Object obj = gc.getName();
9 System.out.println(obj);
10
11 //使用泛型为Integer类
12 GenericClass<Integer> gci = new GenericClass<>();
13 gci.setName(1);
14 Integer integer = gci.getName();
15 System.out.println(integer);
16 }
17 }
定义和使用含有泛型的方法:
格式:修饰符<泛型> 返回值类型 方法名(参数列表(使用泛型)){
语句体;
}
注意:含有泛型的方法,在调用方法的时候确定泛型的数据类型,传递什么类型的参数,泛型就是什么类型
1 package demosummary.generic;
2
3 public class GenericMethod {
4 //定义一个含有泛型的方法
5 public <E> void method(E e){
6 System.out.println(e);
7 }
8
9 //定义一个含有泛型的静态方法
10 public static <M> void method01(M m){
11 System.out.println(m);
12 }
13 }
1 package demosummary.generic;
2
3 public class GenericMethodTest {
4 public static void main(String[] args) {
5 //创建一个GenericMethod对象
6 GenericMethod gm = new GenericMethod();
7 gm.method(1);//传入Integer类型
8 gm.method("字符串");//传入String类型
9 gm.method(1.1);//传入Float类型
10 gm.method(true);//传入Boolean类型
11
12 //静态方法建议通过类名调用
13 GenericMethod.method01(2);//传入Integer类型
14 GenericMethod.method01("静态方法");//传入String类型
15 }
16 }
定义和使用含有泛型的接口:
格式:
修饰符 interface接口名<代表泛型的变量>{ }
第一种使用方式:
定义接口的实现类,实现接口,指定接口的泛型
第二种使用方式:
接口使用什么泛型,实现类就使用什么泛型,类跟着接口走
1 package demosummary.generic;
2 //定义一个抽象类
3 public interface GenericInterface<E> {
4 //创建一个抽象方法
5 public void method(E e);
6 }
1 package demosummary.generic;
2
3 public class GenericInterface01 implements GenericInterface<String>{
4 //重写抽象方法
5 @Override
6 public void method(String s) {
7 System.out.println(s);
8 }
9 }
1 package demosummary.generic;
2
3 public class GenericInterface02<E> implements GenericInterface<E>{
4 //重写抽象类中的抽象方法
5 @Override
6 public void method(E e) {
7 System.out.println(e);
8 }
9 }
1 package demosummary.generic;
2
3 public class GenericInterfaceTest {
4 public static void main(String[] args) {
5 //创建一个GenericInterface01对象,指定实现类泛型为String类型
6 GenericInterface01 gi01 = new GenericInterface01();
7 gi01.method("泛型指定为字符串");
8
9 //创建一个GenericInterface02对象,接口泛型为Integer类型,实现类也为Integer类型
10 GenericInterface02<Integer> gi02 = new GenericInterface02<>();
11 gi02.method(2);
12
13 //创建一个GenericInterface02对象,接口泛型为String类型,实现类也为String类型
14 GenericInterface02<String> gi021 = new GenericInterface02<>();
15 gi021.method("接口为什么泛型,实现类就是什么泛型");
16 }
17 }