本文主要介绍在Java工程中如何生成ETH钱包的助记词、私钥、地址。
一、在之前创建的spring boot 项目中的 pom.xml文件中加入需要的依赖
<dependency> <groupId>org.bitcoinj</groupId> <artifactId>bitcoinj-core</artifactId> <version>0.14.7</version> </dependency> <dependency> <groupId>org.web3j</groupId> <artifactId>core</artifactId> <version>3.6.0</version> </dependency>
二、创建一个Wallet.java类
1、定义一个path路径常量
/** * path路径 */ private final static ImmutableList<ChildNumber> BIP44_ETH_ACCOUNT_ZERO_PATH = ImmutableList.of(new ChildNumber(44, true), new ChildNumber(60, true), ChildNumber.ZERO_HARDENED, ChildNumber.ZERO);
2、创建一个 createWallet 方法体
/** * 创建钱包 * @throws MnemonicException.MnemonicLengthException */ private static void createWallet() throws MnemonicException.MnemonicLengthException { SecureRandom secureRandom = new SecureRandom(); byte[] entropy = new byte[DeterministicSeed.DEFAULT_SEED_ENTROPY_BITS / 8]; secureRandom.nextBytes(entropy); //生成12位助记词 List<String> str = MnemonicCode.INSTANCE.toMnemonic(entropy); //使用助记词生成钱包种子 byte[] seed = MnemonicCode.toSeed(str, ""); DeterministicKey masterPrivateKey = HDKeyDerivation.createMasterPrivateKey(seed); DeterministicHierarchy deterministicHierarchy = new DeterministicHierarchy(masterPrivateKey); DeterministicKey deterministicKey = deterministicHierarchy .deriveChild(BIP44_ETH_ACCOUNT_ZERO_PATH, false, true, new ChildNumber(0)); byte[] bytes = deterministicKey.getPrivKeyBytes(); ECKeyPair keyPair = ECKeyPair.create(bytes); //通过公钥生成钱包地址 String address = Keys.getAddress(keyPair.getPublicKey()); System.out.println(); System.out.println("助记词:"); System.out.println(str); System.out.println(); System.out.println("地址:"); System.out.println("0x"+address); System.out.println(); System.out.println("私钥:"); System.out.println("0x"+keyPair.getPrivateKey().toString(16)); System.out.println(); System.out.println("公钥:"); System.out.println(keyPair.getPublicKey().toString(16)); }
3、写一个main方法调用 createWallet 方法。
public static void main(String[] args) throws Exception { //创建钱包 createWallet(); }
4、执行main方法 查看输出的钱包信息
助记词:
[usage, universe, dress, client, gold, wealth, short, diet, tourist, leave, roof, conduct]
地址:
0x1f3220b4651503bfe53bb96997fa547c4bc0fdaa
私钥:
0xa91f672a0c2094d20f3beeb4f47e2ce6c356e9081c74d7e3cd261f1bdc279a0b
扫描二维码关注公众号,回复:
7649407 查看本文章
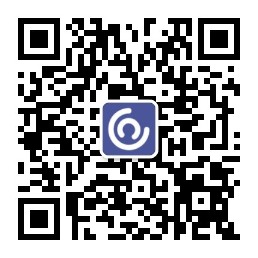
公钥:
462aa97d90c49eaa4ec98c44a64c44606049be5d168c5461c8ed49762fa130d7b3201b336bac26e871c04f5aa84a0d0c868ffd0e04ad2b11d01561d6bb99eb1
5、验证
将上述生成的私钥或助记词在任意支持ETH的钱包中导入,可以看到地址与我们生成的一致,至此说明成功!
附:
完整代码
package com.bizzan.test; import java.security.SecureRandom; import java.util.List; import org.bitcoinj.crypto.ChildNumber; import org.bitcoinj.crypto.DeterministicHierarchy; import org.bitcoinj.crypto.DeterministicKey; import org.bitcoinj.crypto.HDKeyDerivation; import org.bitcoinj.crypto.MnemonicCode; import org.bitcoinj.crypto.MnemonicException; import org.bitcoinj.wallet.DeterministicSeed; import org.web3j.crypto.ECKeyPair; import org.web3j.crypto.Keys; import com.google.common.collect.ImmutableList; public class Wallet { /** * path路径 */ private final static ImmutableList<ChildNumber> BIP44_ETH_ACCOUNT_ZERO_PATH = ImmutableList.of(new ChildNumber(44, true), new ChildNumber(60, true), ChildNumber.ZERO_HARDENED, ChildNumber.ZERO); public static void main(String[] args) throws Exception { //创建钱包 createWallet(); } /** * 创建钱包 * @throws MnemonicException.MnemonicLengthException */ private static void createWallet() throws MnemonicException.MnemonicLengthException { SecureRandom secureRandom = new SecureRandom(); byte[] entropy = new byte[DeterministicSeed.DEFAULT_SEED_ENTROPY_BITS / 8]; secureRandom.nextBytes(entropy); //生成12位助记词 List<String> str = MnemonicCode.INSTANCE.toMnemonic(entropy); //使用助记词生成钱包种子 byte[] seed = MnemonicCode.toSeed(str, ""); DeterministicKey masterPrivateKey = HDKeyDerivation.createMasterPrivateKey(seed); DeterministicHierarchy deterministicHierarchy = new DeterministicHierarchy(masterPrivateKey); DeterministicKey deterministicKey = deterministicHierarchy .deriveChild(BIP44_ETH_ACCOUNT_ZERO_PATH, false, true, new ChildNumber(0)); byte[] bytes = deterministicKey.getPrivKeyBytes(); ECKeyPair keyPair = ECKeyPair.create(bytes); //通过公钥生成钱包地址 String address = Keys.getAddress(keyPair.getPublicKey()); System.out.println(); System.out.println("助记词:"); System.out.println(str); System.out.println(); System.out.println("地址:"); System.out.println("0x"+address); System.out.println(); System.out.println("私钥:"); System.out.println("0x"+keyPair.getPrivateKey().toString(16)); System.out.println(); System.out.println("公钥:"); System.out.println(keyPair.getPublicKey().toString(16)); } }
币严(BIZZAN.COM)数字货币交易平台官方网址:
https://www.bizzan.com