版权声明:本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。
数据结构学习笔记:实现链表
1、结点结构
结点结构是由数据域和指针域组成,数据域是存放数据的,而指针域存放下一结点的地址。
2、链表结构
通过数据域访问到我们要的数据,而通过指针域访问到当前结点以后的结点,那么将这些结点串起来,就是一个链表。
3、案例演示——JavaScript实现链表
(1)创建WebStorm项目,添加LinkedList.html与LinkedList.js
(2)编写LinkeList.html
扫描二维码关注公众号,回复:
7585302 查看本文章
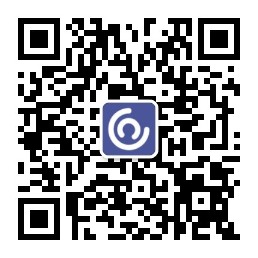
(3)编写LinkedList.js
// 定义结点
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
// 定义链表
class LinkedList {
constructor() {
// 初始化头结点
this.head = new Node('head');
}
/**
* 根据值查找结点
* @param value
* @returns {*}
*/
findByValue = (value) => {
// 从头结点开始查找
let currentNode = this.head;
// 如果当前结点非空且数据不等于查找值,就移向下一个结点
while (currentNode != null && currentNode.data != value) {
currentNode = currentNode.next;
}
// 判断按值是否找到结点,找到就返回当前结点,否则返回-1
return currentNode == null ? -1 : currentNode;
}
/**
* 根据索引查找结点
* @param index
* @returns {*}
*/
findByIndex = (index) => {
// 当前位置
let pos = 0;
let currentNode = this.head;
// 如果当前结点非空且当前位置不等于查找索引,就移向下一个结点,当前位置递增1
while (currentNode != null && pos != index) {
currentNode = currentNode.next;
pos++;
}
// 判断按索引是否找到结点,找到就返回当前结点,否则返回-1
return currentNode == null ? -1 : currentNode;
}
/**
* 在指定元素后插入新元素
* @param value
* @param element
*/
insert = (value, element) => {
// 查找元素
let currentNode = this.findByValue(element);
// 判断是否找到
if (currentNode == -1) {
console.log("未找到插入位置!");
} else {
// 创建新结点
let newNode = new Node(value);
// 让新结点指向当前结点指向的下一个结点
newNode.next = currentNode.next;
// 让当前结点指向新结点
currentNode.next = newNode;
}
}
/**
* 更新指定元素的值
* @param value
* @param element
*/
update = (value, element) => {
// 查找元素
let currentNode = this.findByValue(element);
// 判断是否找到
if (currentNode == -1) {
console.log("未找到指定元素[" + element.data + "]!");
} else {
currentNode.data = value;
}
}
/**
* 根据值删除结点
* @param value
* @returns {number}
*/
delete = (value) => {
let currentNode = this.head;
let previousNode = null;
while (currentNode != null && currentNode.data != value) {
previousNode = currentNode;
currentNode = currentNode.next;
}
if (currentNode == null) {
return -1;
} else {
previousNode.next = currentNode.next;
}
}
/**
* 遍历全部结点并打印其值
*/
printAll = () => {
let currentNode = this.head;
while (currentNode != null) {
console.log(currentNode.data);
currentNode = currentNode.next;
}
}
}
// 测试链表操作
const list = new LinkedList();
list.printAll();
console.log('插入三个结点:mike, howard, alice');
list.insert('mike', 'head');
list.insert('alice', 'mike');
list.insert('howard', 'mike');
list.printAll();
console.log("按值查找alice结点");
console.log(list.findByValue('alice').data);
console.log('按索引查找2结点');
console.log(list.findByIndex(2).data);
console.log('将alice修改为jenny');
list.update('jenny', 'alice');
list.printAll();
console.log('删除howard结点')
list.delete('howard');
list.printAll();
(4)浏览LinkedList.html,打开调试窗口,查看链表操作结果
4、案例演示——Python实现
# 实现链表
# 定义结点
class Node:
def __init__(self, data):
self.data = data;
self.next = None;
# 定义链表
class LinkedList:
def __init__(self):
# 初始化头结点
self.head = Node('head');
# 根据值查找结点
def findByValue(self, value):
currentNode = self.head
while currentNode != None and currentNode.data != value:
currentNode = currentNode.next
return -1 if currentNode == None else currentNode
# 根据索引查找结点
def findByIndex(self, index):
pos = 0
currentNode = self.head
while currentNode != None and pos != index:
currentNode = currentNode.next
pos = pos + 1
return -1 if currentNode == None else currentNode
# 在指定元素后插入新元素
def insert(self, value, element):
currentNode = self.findByValue(element)
if currentNode == -1:
print("未找到插入位置!")
else:
newNode = Node(value)
newNode.next = currentNode.next
currentNode.next = newNode
# 更新指定元素的值
def update(self, value, element):
currentNode = self.findByValue(element)
if currentNode == -1:
print("未找到指定元素[" + element.data + "]!")
else:
currentNode.data = value
# 根据值删除结点
def delete(self, value):
currentNode = self.head
previousNode = None
while currentNode != None and currentNode.data != value:
previousNode = currentNode
currentNode = currentNode.next
if currentNode == None:
return -1
else:
previousNode.next = currentNode.next
# 遍历全部结点并打印其值
def printAll(self):
currentNode = self.head
while currentNode != None:
print(currentNode.data)
currentNode = currentNode.next
# 测试链表操作
list = LinkedList()
list.printAll()
print('插入三个结点:mike, howard, alice');
list.insert('mike', 'head');
list.insert('alice', 'mike');
list.insert('howard', 'mike');
list.printAll();
print("按值查找alice结点");
print(list.findByValue('alice').data);
print('按索引查找2结点');
print(list.findByIndex(2).data);
print('将alice修改为jenny');
list.update('jenny', 'alice');
list.printAll();
print('删除howard结点')
list.delete('howard');
list.printAll();
运行结果如下: