# coding: utf-8
import matplotlib.pyplot as plt
import numpy as np
x1 = np.random.normal(30, 3, 100)
x2 = np.random.normal(20, 2, 100)
x3 = np.random.normal(10, 3, 100)
# 随机正态分布生成x1、x2、x3,并为每个plot指定一个字符串标签
plt.plot(x1, label="plot")
plt.plot(x2, label="plot2")
plt.plot(x3, label="plot3")
# 设置loc参数确定图例框的位置
# 设置列数为nloc=3,指定边框的(bbox_to_anchor)的起始位置为(0.0,1.02),设置宽度为1,高度为0.102,注意这些值都是基于归一化坐标系。参数mode可以设置为None或者expand,当为expand时,图例框会水平的扩展至整个坐标轴区域。参数borderaxespad指定了坐标轴和图例边界之间的间距。
plt.legend(bbox_to_anchor=(0., 1.02, 1., .102), loc=3,
ncol=3, mode="expand", borderaxespad=0.)
# 进行备注
plt.annotate("Important value", (55,20), xycoords='data',
xytext=(5, 38),
arrowprops=dict(arrowstyle='->'))
plt.show()
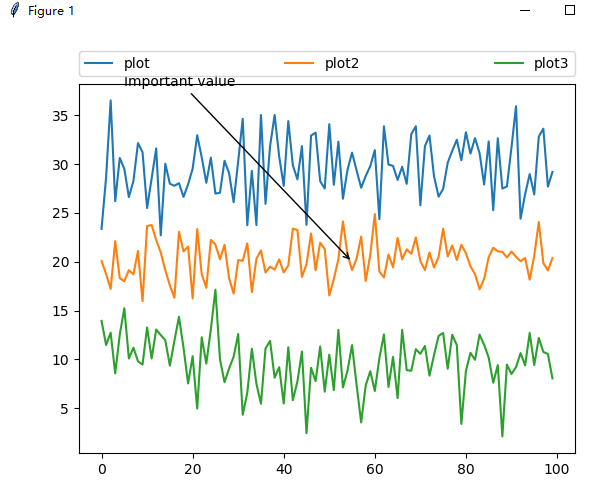
plt.annotate()函数解析:
# plt.annotate()函数用于标注文字
plt.annotate(s='str', xy=(x,y) , xytext=(l1,l2) , ... )
参数解释:
s 为注释文本内容
xy 为被注释的坐标点
xytext 为注释文字的坐标位置
xycoords 参数如下:
figure points:图左下角的点
figure pixels:图左下角的像素
figure fraction:图的左下部分
axes points:坐标轴左下角的点
axes pixels:坐标轴左下角的像素
axes fraction:左下轴的分数
data:使用被注释对象的坐标系统(默认)
polar(theta,r):if not native ‘data’ coordinates t
weight 设置字体线型
{‘ultralight’, ‘light’, ‘normal’, ‘regular’, ‘book’, ‘medium’, ‘roman’, ‘semibold’, ‘demibold’, ‘demi’, ‘bold’, ‘heavy’, ‘extra bold’, ‘black’}
color 设置字体颜色
{‘b’, ‘g’, ‘r’, ‘c’, ‘m’, ‘y’, ‘k’, ‘w’}
‘black’,'red’等
[0,1]之间的浮点型数据
RGB或者RGBA, 如: (0.1, 0.2, 0.5)、(0.1, 0.2, 0.5, 0.3)等
arrowprops #箭头参数,参数类型为字典dict
width:箭头的宽度(以点为单位)
headwidth:箭头底部以点为单位的宽度
headlength:箭头的长度(以点为单位)
shrink:总长度的一部分,从两端“收缩”
facecolor:箭头颜色
bbox给标题增加外框 ,常用参数如下:
boxstyle:方框外形
facecolor:(简写fc)背景颜色
edgecolor:(简写ec)边框线条颜色
edgewidth:边框线条大小
# coding: utf-8
import matplotlib.pyplot as plt
import numpy as np
def test1():
x = np.arange(0, 50, 5)
print(x)
y = x * x
plt.plot(x, y, marker="o")
for xy in zip(x,y):
plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(2, 4), textcoords='offset points')
plt.show()
# test1()
def test2():
x = np.arange(0,50, 5)
y = x * x
plt.plot(x, y, marker='o')
# weight:增加字体线型
for xy in zip(x, y):
plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(-20, 10), textcoords='offset points', weight='heavy')
plt.show()
# test2()
def test3():
x = np.arange(0,50,5)
y = x * x
plt.plot(x, y, marker='o')
# 增加字体的颜色
for xy in zip(x, y):
plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(-20, 10), textcoords='offset points', color='y', weight='heavy')
plt.show()
# test3()
def test4():
x = np.arange(0, 50, 10)
y = x * x
plt.plot(x, y, marker='o')
# 增加箭头
for xy in zip(x, y):
plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(-20, 10), textcoords='offset points', color='y', weight='heavy',
arrowprops=dict(facecolor="y", headlength=10, headwidth=10, width=20))
plt.show()
# test4()
def test5():
x = np.arange(0, 50, 10)
y = x * x
plt.plot(x, y, marker='o')
# 增加标题外框
for xy in zip(x, y):
plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(-20, 10), textcoords='offset points',color='y', weight='heavy',
arrowprops=dict(facecolor="y", headlength=10, headwidth=10, width=20),
bbox=dict(boxstyle='round,pad=0.5', fc='yellow', ec='k', lw=1, alpha=0.5))
plt.show()
# test5()
def test6():
x = np.arange(0, 50, 10)
y = x * x
plt.plot(x, y, marker='o')
# 增加箭头指示
for xy in zip(x, y):
# plt.annotate("(%s,%s)" % xy, xy=xy, xytext=(-20, 10), textcoords='offset points', color='y', weight='heavy',
# arrowprops=dict(facecolor="y", headlength=10, headwidth=10, width=20),
# bbox=dict(boxstyle='round,pad=0.5', fc='yellow', ec='k', lw=1, alpha=0.5))
plt.annotate('local max', xy=(5, 25), xytext=(20, 25), arrowprops=dict(facecolor='red', shrink=0.05))
plt.show()
# test6()