8.表名和类名不同,对表名进行配置
8.1在使用注解的实体类
//表名和类名不同,对表名进行配置;使用Table注解
@Table(name = "_teacher") //name后的为数据库中对应的表名
public class Teacher {}
执行TestCase时,console的语句
insert
into
_teacher
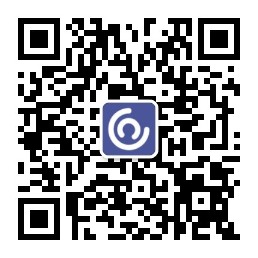
(name, title, id)
values
(?, ?, ?)
数据库中会出现_teacher,进行对表的操作。
8.2在使用配置文件时,/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Student.hbm.xml
只要加上table属性对应的数据库中表的名称
<class name="Student" table="_student">
代码案例:
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<!-- 找不到entity,是因为这个类没改包名 -->
<hibernate-mapping package="com.zhuhw.hibernate.model">
<class name="Student" table="_student">
<!-- id主键;name=id对应的是Student中的getid() -->
<id name="id" ></id>
<property name="name" />
<property name="age" />
<!-- hibernater知道了怎么将class与表中的字段对应到一起了 -->
</class>
</hibernate-mapping>
出现的问题:
An AnnotationConfiguration instance is required to use <mapping class="com.zhuhw.hibernate.model.Teacher"/>
使用Configuration cf = new AnnotationConfiguration();
Configuration类只支持xml配置方式,AnnotationConfiguration扩展了Configuration类,同时支持xml配置和注解方式。
老师的价值就在项目上,在综合运用上。
从老美的认为:
1.查的能力
2.综合运用的能力
查询E:\zhuhw\hibernate\jar\hibernate-annotations-3.4.0.GA\doc\reference\zh_cn\html_single文档
用JPA的标准的
9.字段名和属性相同
a)默认为@Basic
b)xml中不用写column
例如:
@Basic //对数据库中,字段名和属性相同
public void setId(int id) {
this.id = id;
}
10.字段名属性不同
a)Annotation:@Column (直接写在get方法上)
b)xml:自己查询
注解方式代码案例:
/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Teacher.java
//字段名属性不同a)Annotation:@Column
@Column(name = "_name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
执行结果:
console:
insert
into
_teacher
(_name, title, id)
values
(?, ?, ?)
数据库:增加_name与setName()对应。
xml方式,/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Student.hbm.xml
<property name="name" column="_name" />
需要Hibernate API 中文版啊
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<!-- 找不到entity,是因为这个类没改包名 -->
<hibernate-mapping package="com.zhuhw.hibernate.model">
<class name="Student" table="_student">
<!-- id主键;name=id对应的是Student中的getid() -->
<id name="id" ></id>
<property name="name" column="_name" />
<property name="age" />
<!-- hibernater知道了怎么将class与表中的字段对应到一起了 -->
</class>
</hibernate-mapping>
11.不需要persistence的字段
a)Annotation:@Transient
b)xml:不写就好了(/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Student.hbm.xml)
<property name="age" />不写
不要存到数据库中,
@Transient存储的时候把我当透明人就行了,不需要加到数据库中(就存到内存里就行了)
代码案例:
/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Teacher.java
private String yourWifeName;
//不要存到数据库中
@Transient
public String getYourWifeName() {
return yourWifeName;
}
public void setYourWifeName(String yourWifeName) {
this.yourWifeName = yourWifeName;
}
12.映射日期与时间类型,指定时间精度
a)Annotation:@Temporal
b)xml:指定type
代码案例:
//映射日期与时间类型,指定时间精度
//通过@Temporal可以指定时间的精度
@Temporal(value=TemporalType.DATE)
public Date getBirthdate() {
return birthdate;
}
public void setBirthdate(Date birthdate) {
this.birthdate = birthdate;
}
正常的运行结果:
insert
into
_teacher
(birthdate, _name, title, yourWifeName, id)
values
(?, ?, ?, ?, ?)
insert
into
_teacher
(date, _name, title, yourWifeName, id)
values
(?, ?, ?, ?, ?)
把日期和时间都存起来了
@Temporal //通过@Temporal可以指定时间的精度
这里存在问题....
-----是没有按比如只有日期显示/时间显示
求解:
我错了
....写成Date date;关键字重复了... 所以木有生效呢
换下变量名称就ok了
2016-01-01
13.映射枚举类型
a)@Enumerared
b)xml:麻烦
数据库:
1.数据库存储String
/*@Enumerated(EnumType.STRING)
`zhicheng` varchar(255) DEFAULT NULL,*/
@Enumerated(EnumType.STRING)
A
2.数据库存储下标值
/*@Enumerated(EnumType.ORDINAL)
* 数据库存储下标值
`zhicheng` varchar(255) DEFAULT NULL,*/
@Enumerated(EnumType.ORDINAL)
生成一个enum类,
package com.zhuhw.hibernate.model;
public enum ZhiCheng {
A,B,C;
}
在/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Teacher.java,
使用
//ZhiCheng enum
private ZhiCheng zhicheng;
public ZhiCheng getZhicheng() {
return zhicheng;
}
public void setZhicheng(ZhiCheng zhicheng) {
this.zhicheng = zhicheng;
}
14.字段映射的位置(field或者get方法)
a)best practice:保持gfield和 get set方法一致
15.@Lob
16.课外:CLOB BLOB类型的数据存储
17.课外:hibernate自定义数据类型
18.hibernate类型
在95%以上的没必要指定类型
由hibernate自动默认帮我们实现就好了。
本章节完整代码:
package com.zhuhw.hibernate.model; import java.util.Date; import javax.persistence.Basic; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.EnumType; import javax.persistence.Enumerated; import javax.persistence.Id; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; import javax.persistence.Transient; /*1.实体类(使用annotation进行实现,告诉hibernate是实体类, * 不需要再建立Teacher.hbm.xml文件进行映射) * 2.hibernate.cfg.xml进行配置即可 * 3.建立一个TeacherTest */ @Entity //表名和类名不同,对表名进行配置 @Table(name = "_teacher") public class Teacher { private int id; private String name; private String title; private Date birthdate; //ZhiCheng enum private ZhiCheng zhicheng; /*@Enumerated(EnumType.STRING) * 数据库存储String `zhicheng` varchar(255) DEFAULT NULL, */ /*@Enumerated(EnumType.ORDINAL) * 数据库存储下标值 `zhicheng` varchar(255) DEFAULT NULL,*/ @Enumerated(EnumType.ORDINAL) public ZhiCheng getZhicheng() { return zhicheng; } public void setZhicheng(ZhiCheng zhicheng) { this.zhicheng = zhicheng; } //映射日期与时间类型,指定时间精度 //通过@Temporal可以指定时间的精度 @Temporal(value=TemporalType.DATE) public Date getBirthdate() { return birthdate; } public void setBirthdate(Date birthdate) { this.birthdate = birthdate; } private String yourWifeName; //不要存到数据库中 /*@Transient*/ public String getYourWifeName() { return yourWifeName; } public void setYourWifeName(String yourWifeName) { this.yourWifeName = yourWifeName; } //主键 @Id public int getId() { return id; } @Basic//对数据库中,字段名和属性相同 public void setId(int id) { this.id = id; } //字段名属性不同a)Annotation:@Column @Column(name = "_name") public String getName() { return name; } public void setName(String name) { this.name = name; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
/hibernate_0100_HelloWorld/test/com/zhuhw/hibernate/model/TeacherTest.java
Junit Test Case
package com.zhuhw.hibernate.model; import java.util.Date; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.cfg.AnnotationConfiguration; import org.junit.AfterClass; import org.junit.BeforeClass; import org.junit.Test; public class TeacherTest { /*这个类TeacherTest一进来就被初始化了,在测试方法执行用已经初始化好的SessionFactory; * 只初始化一次*/ public static SessionFactory sf = null; @BeforeClass public static void beforeClass(){ /*关于junit会出现吞掉bug,1.可以在下面的语句上加上try catch * 2.或者加个main()方法,在main里面进行调用 beforeClass()*/ sf = new AnnotationConfiguration().configure().buildSessionFactory(); } @Test public void TestTeacherSave(){ Teacher t = new Teacher(); t.setId(14); t.setName("zhuhw14"); t.setTitle("ccc14"); t.setBirthdate(new Date()); t.setYourWifeName("yourWifeName14"); t.setZhicheng(ZhiCheng.A); //因为使用的annotation,所以Configuration要使用AnnotationConfiguration /*Configuration cf = new AnnotationConfiguration(); SessionFactory sf = cf.configure().buildSessionFactory();*/ Session session = sf.openSession(); //在hibernate中执行操作要在一个事务里面 session.beginTransaction(); session.save(t); session.getTransaction().commit(); session.close(); } /*关于junit会出现吞掉bug,1.可以在下面的语句上加上try catch * 2.或者加个main()方法,在main里面进行调用 beforeClass()*/ public void main() { beforeClass(); } @AfterClass public static void afterClass(){ sf.close(); } }
/hibernate_0100_HelloWorld/src/hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost/hibernate</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- JDBC connection pool (use the built-in) --> <!--<property name="connection.pool_size">1</property>--> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Enable Hibernate's automatic session context management --> <!--<property name="current_session_context_class">thread</property>--> <!-- Disable the second-level cache --> <property name="cache.provider_class">org.hibernate.cache.NoCacheProvider</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="format_sql">true</property> <!-- Drop and re-create the database schema on startup --> <property name="hbm2ddl.auto">update</property> <mapping resource="com/zhuhw/hibernate/model/Student.hbm.xml"/> <mapping class="com.zhuhw.hibernate.model.Teacher"/> </session-factory> </hibernate-configuration>
/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Student.java
package com.zhuhw.hibernate.model; public class Student { private int id; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } private String name; private int age; }
/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/StudentTest.java
package com.zhuhw.hibernate.model;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.AnnotationConfiguration;
import org.hibernate.cfg.Configuration;
public class StudentTest {
public static void main(String[] args){
Student s = new Student();
s.setId(9);
s.setName("yuzhou9");
s.setAge(9);
Configuration cf = new AnnotationConfiguration();
SessionFactory sf = cf.configure().buildSessionFactory();
Session session = sf.openSession();
//在hibernate中执行操作要在一个事务里面
session.beginTransaction();
session.save(s);
session.getTransaction().commit();
session.close();
sf.close();
}
}
/hibernate_0100_HelloWorld/src/com/zhuhw/hibernate/model/Student.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- 找不到entity,是因为这个类没改包名 --> <hibernate-mapping package="com.zhuhw.hibernate.model"> <class name="Student" table="_student"> <!-- id主键;name=id对应的是Student中的getid() --> <id name="id" ></id> <property name="name" column="_name" /> <property name="age" /> <!-- hibernater知道了怎么将class与表中的字段对应到一起了 --> </class> </hibernate-mapping>
package com.zhuhw.hibernate.model; public enum ZhiCheng { A,B,C; } /hibernate_0100_HelloWorld/src/log4j.properties ### direct log messages to stdout ### log4j.appender.stdout=org.apache.log4j.ConsoleAppender log4j.appender.stdout.Target=System.out log4j.appender.stdout.layout=org.apache.log4j.PatternLayout log4j.appender.stdout.layout.ConversionPattern=%d{ABSOLUTE} %5p %c{1}:%L - %m%n ### direct messages to file hibernate.log ### #log4j.appender.file=org.apache.log4j.FileAppender #log4j.appender.file.File=hibernate.log #log4j.appender.file.layout=org.apache.log4j.PatternLayout #log4j.appender.file.layout.ConversionPattern=%d{ABSOLUTE} %5p %c{1}:%L - %m%n ### set log levels - for more verbose logging change 'info' to 'debug' ### log4j.rootLogger=warn, stdout #log4j.logger.org.hibernate=info log4j.logger.org.hibernate=debug ### log HQL query parser activity #log4j.logger.org.hibernate.hql.ast.AST=debug ### log just the SQL #log4j.logger.org.hibernate.SQL=debug ### log JDBC bind parameters ### log4j.logger.org.hibernate.type=info #log4j.logger.org.hibernate.type=debug ### log schema export/update ### log4j.logger.org.hibernate.tool.hbm2ddl=debug ### log HQL parse trees #log4j.logger.org.hibernate.hql=debug ### log cache activity ### #log4j.logger.org.hibernate.cache=debug ### log transaction activity #log4j.logger.org.hibernate.transaction=debug ### log JDBC resource acquisition #log4j.logger.org.hibernate.jdbc=debug ### enable the following line if you want to track down connection ### ### leakages when using DriverManagerConnectionProvider ### #log4j.logger.org.hibernate.connection.DriverManagerConnectionProvider=trace