import org.eclipse.swt.SWT; import org.eclipse.swt.layout.GridData; import org.eclipse.swt.layout.GridLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Shell; public class GridData_GridLayoutTest2 { protected Shell shell; /** * Launch the application. * @param args */ public static void main(String[] args) { try { GridData_GridLayoutTest2 window = new GridData_GridLayoutTest2(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); GridLayout layout = new GridLayout(); layout.numColumns = 3; shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); Button button1 = new Button(shell, SWT.PUSH); button1.setText("button1"); GridData buttonGrid = new GridData(SWT.FILL, SWT.FILL, false, false, 1, 1); buttonGrid.heightHint = 100; buttonGrid.widthHint = 100; button1.setLayoutData(buttonGrid); Button button2 = new Button(shell, SWT.PUSH); button2.setText("button2"); GridData button2data = new GridData(SWT.FILL,SWT.FILL,false,false,1,2); buttonGrid.heightHint = 100; buttonGrid.widthHint = 100; button2.setLayoutData(button2data); Button button3 = new Button(shell, SWT.PUSH); button3.setText("button3"); GridData button3data = new GridData(GridData.FILL_BOTH); button3.setLayoutData(button3data); Button button4 = new Button(shell, SWT.PUSH); button4.setText("button4"); GridData button4Grid = new GridData(SWT.FILL, SWT.FILL, false, false, 1, 1); button4Grid.heightHint = 100; button4Grid.widthHint = 100; button4.setLayoutData(button4Grid); } }
import org.eclipse.swt.SWT; import org.eclipse.swt.layout.GridData; import org.eclipse.swt.layout.GridLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Label; import org.eclipse.swt.widgets.Shell; import org.eclipse.swt.widgets.Text; public class GridData_GridLayoutTest { protected Shell shell; /** * Launch the application. * @param args */ public static void main(String[] args) { try { GridData_GridLayoutTest window = new GridData_GridLayoutTest(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); GridLayout layout = new GridLayout(); layout.numColumns = 4; shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); Label label0 = new Label(shell, SWT.NONE); label0.setText("label0"); Text text1 = new Text(shell, SWT.BORDER); text1.setText("text1"); GridData data = new GridData(); data.horizontalAlignment = GridData.FILL; data.horizontalSpan = 2; data.grabExcessHorizontalSpace = true; text1.setLayoutData(data); Button button2 = new Button(shell, SWT.PUSH); button2.setText("button2"); Text text3 = new Text(shell, SWT.BORDER); text3.setText("text3"); data = new GridData(); data.horizontalAlignment = GridData.FILL; data.verticalAlignment = GridData.FILL; data.horizontalSpan = 4; data.grabExcessHorizontalSpace = true; text3.setLayoutData(data); } }
import org.eclipse.swt.SWT; import org.eclipse.swt.graphics.Color; import org.eclipse.swt.layout.FormAttachment; import org.eclipse.swt.layout.FormData; import org.eclipse.swt.layout.FormLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Shell; import org.eclipse.swt.widgets.Text; public class FormLayoutTest { protected Shell shell; private Color color; /** * Launch the application. * @param args */ public static void main(String[] args) { try { FormLayoutTest window = new FormLayoutTest(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); FormLayout layout = new FormLayout(); shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } display.dispose(); color.dispose(); } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); Button cancelButton = new Button(shell,SWT.NONE); cancelButton.setText("取消"); FormData dataCancel = new FormData(); dataCancel.right = new FormAttachment(100,-5);//100代表到100%的宽度位置,-5代表减去5,如果第一个参数为控件,那就是相对控件的位置 dataCancel.bottom = new FormAttachment(100,-5); cancelButton.setLayoutData(dataCancel); Button okButton = new Button(shell,SWT.NONE); okButton.setText("确定"); FormData dataOk = new FormData(); dataOk.right = new FormAttachment(100,-60); dataOk.bottom = new FormAttachment(100,-5); okButton.setLayoutData(dataOk); Text text = new Text(shell,SWT.BORDER|SWT.MULTI|SWT.V_SCROLL|SWT.H_SCROLL); FormData dataText = new FormData(); dataText.top = new FormAttachment(0,5); dataText.bottom = new FormAttachment(cancelButton,-5); dataText.left = new FormAttachment(0,5); dataText.right = new FormAttachment(100,-5); text.setLayoutData(dataText); color = new Color(null,255,0,0,0); text.setForeground(color);//设置颜色,最后要记得调用color.dispose()释放资源 } }
import org.eclipse.swt.SWT; import org.eclipse.swt.layout.GridLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Shell; public class GridLayoutTest { protected Shell shell; /** * Launch the application. * @param args */ public static void main(String[] args) { try { GridLayoutTest window = new GridLayoutTest(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); GridLayout layout = new GridLayout(); layout.numColumns = 3; shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } display.dispose(); } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); for(int i = 0;i<10;i++){ Button button = new Button(shell, SWT.PUSH); button.setText("Button"+i); } } }
import org.eclipse.swt.SWT; import org.eclipse.swt.layout.RowLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Shell; public class RowLayoutTest { protected Shell shell; /** * Launch the application. * @param args */ public static void main(String[] args) { try { RowLayoutTest window = new RowLayoutTest(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); RowLayout layout = new RowLayout(); shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } display.dispose(); } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); for(int i = 0;i<10;i++){ Button button = new Button(shell, SWT.PUSH); button.setText("Button"+i); } } }
import org.eclipse.swt.SWT; import org.eclipse.swt.layout.FillLayout; import org.eclipse.swt.widgets.Button; import org.eclipse.swt.widgets.Display; import org.eclipse.swt.widgets.Shell; public class FillLayoutTest { protected Shell shell; /** * Launch the application. * @param args */ public static void main(String[] args) { try { FillLayoutTest window = new FillLayoutTest(); window.open(); } catch (Exception e) { e.printStackTrace(); } } /** * Open the window. */ public void open() { Display display = Display.getDefault(); createContents(); shell.open(); FillLayout layout = new FillLayout(); shell.setLayout(layout); shell.layout(); while (!shell.isDisposed()) { if (!display.readAndDispatch()) { display.sleep(); } } display.dispose(); } /** * Create contents of the window. */ protected void createContents() { shell = new Shell(); shell.setSize(450, 300); shell.setText("SWT Application"); for(int i = 0;i<10;i++){ Button button = new Button(shell, SWT.PUSH); button.setText("Button"+i); } } }
Eclipse的布局机制,提供了两个对象概念,Layout(描述内部布局方式,也就是子控件)以及GridData(描述本身布局方式)。FillLayout,GridLayout,FormLayout,RowLayout
一 (1)Fillloyout
填充布局
拉伸
(2)RowLayout
行布局,内部子元素以行布局,遇到边界时,自动换行
压缩后
扫描二维码关注公众号,回复:
694799 查看本文章
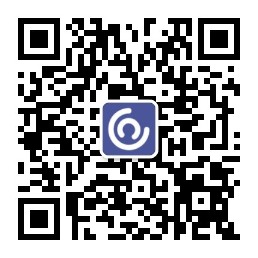
(3)GridLayout
网格布局,使内部子元素按指定的每行几个来布局
压缩
(4)FormLayout
表单布局 通过内部元素setLayoutData指定的FormData进行布局
拉升后,下面2个控件的相对位置是不会改变的 ,适合做类似表单及登录窗口这种
二、GridData
描述控件本身的摆放方式,一般与GridLayout配合使用。它最多的一个构造方法是6个参数:
GridData buttonGrid = new GridData(SWT.FILL, SWT.FILL, false, false, 1, 1);
第一个参数:水平方向如何对齐
第二个参数:竖直方向如何对齐
第三个参数:是否占用水平的剩余空间
第四个参数:是否占用竖直的剩余空间
第五个参数:水平的列数
第六个参数:竖直的行数
下面是2个例子的截图 配合着代码自己看看 都是很简单的