
from collections import namedtuple point = namedtuple('坐标',['x','y','z']) # 第二个参数既可以传可迭代对象如(列表 ,元组) # point = namedtuple('坐标','x y z') # 也可以传字符串 但是字符串之间以空格隔开 p = point(1,2,5) # 注意元素的个数必须跟namedtuple第二个参数里面的值数量一致 print(p) # 坐标(x=1, y=2, z=5) print(p.x) # 1 print(p.y) # 2 print(p.z) # 5
2.deque双端队列(可以快速的从另外一侧追加和推出对象)
# 队列:现进先出(FIFO first in first out)

import queue q = queue.Queue() # 生成队列对象 q.put('first') # 往队列中添加值 q.put('second') q.put('third') print(q.get()) # 朝队列要值 first print(q.get()) # second print(q.get()) # third print(q.get()) # 如果队列中的值取完了再取的话 程序会在原地等待 直到从队列中拿到值才停止

from collections import deque q = deque(['a','b','c']) """ append() # 尾增 appendleft() # 左头增 insert(索引,值) 索引增值 pop() 末尾取值 popleft() 左头取值 """ q.append(1) # 尾增1 q.appendleft(2) # 左头增2 # [2,'a','b','c',1] print(q.pop()) # 1 print(q.pop()) # c print(q.popleft()) # 2 print(q.popleft()) # a

from collections import deque q = deque(['a','b','c']) """ append() # 尾增 appendleft() # 左头增 insert(索引,值) 索引增值 pop() 末尾取值 popleft() 左头取值 """ q.append(1) # 尾增1 q.appendleft(2) # 左头增2 # """ # 队列不应该支持任意位置插值 # 只能在首尾插值(不能插队) # """ # ['哈哈哈',2,'a','b','c',1] q.insert(0,'哈哈哈') # 特殊点:双端队列可以根据索引在任意位置插值 print(q.pop()) # 1 print(q.popleft()) # 哈哈哈 print(q.popleft()) # 2
3..OrderedDict: 有序字典
使用dict时,Key是无序的。在对dict做迭代时,我们无法确定Key的顺序。如果要保持Key的顺序,可以用OrderedDict

d = dict([('a', 1), ('b', 2), ('c', 3)]) print(d) # dict的Key是无序的 {'a': 1, 'c': 3, 'b': 2} from collections import OrderedDict order_d = OrderedDict([('a',1),('b',2),('c',3)]) print(order_d) # OrderedDict([('a', 1), ('b', 2), ('c', 3)]) order_d1 = OrderedDict() order_d1['x'] = 1 order_d1['y'] = 2 order_d1['z'] = 3 print(order_d1) # OrderedDict([('x', 1), ('y', 2), ('z', 3)]) for i in order_d1: print(i) # x y z
4.defaultdict: 带有默认值的字典

from collections import defaultdict values = [11, 22, 33,44,55,66,77,88,99,90] my_dict = defaultdict(list) # 后续该字典中新建的key对应的value默认就是列表,并且各个value之间互不影响 print(my_dict['aa']) # 添加key:'aa' value:默认为[] for value in values: if value>66: my_dict['k1'].append(value) else: my_dict['k2'].append(value) print(my_dict) # defaultdict(<class 'list'>, {'aa': [], 'k2': [11, 22, 33, 44, 55, 66], 'k1': [77, 88, 99, 90]})
5..Counter: 计数器,主要用来计数

rom collections import Counter s = 'abcdeabcdabcaba' # 也可为列表 res = Counter(s) print(res) # Counter({'a': 5, 'b': 4, 'c': 3, 'd': 2, 'e': 1})

# 1.时间戳(timestamp):表示的是从1970年1月1日00:00:00开始按秒计算的偏移量单位秒 import time print(time.time()) # 2.延迟程序的运行:线程推迟指定的时间运行,secs`为秒, time.sleep(secs) # 3.格式化时间:time.strftime(fmt[,tupletime]) print(time.strftime("%Y-%m-%d %H:%M:%S"))# # 2019-05-07 19:16:21 # print(time.strftime('%Y-%m-%d %X')) # %X等价于%H:%M:%S res = time.strftime("%Y-%m-%d %H:%M:%S", (2008, 8, 8, 8, 8, 8, 0, 0, 0)) print(res) # 2008-08-08 08:08:08 可以格式化打印当前时间和传入时间的格式(传入的时间要为9位 4.结构化时间(当前时区时间):time.localtime() print(time.localtime()) # time.struct_time(tm_year=2019, tm_mon=7, tm_mday=18, tm_hour=18, tm_min=39, tm_sec=12, tm_wday=3, tm_yday=199, tm_isdst=0) print(time.localtime(time.time())) # # time.struct_time(tm_year=2019, tm_mon=7, tm_mday=18, tm_hour=18, tm_min=39, tm_sec=12, tm_wday=3, tm_yday=199, tm_isdst=0) print(time.localtime(1473525444.037215)) #time.struct_time(tm_year=2016, tm_mon=9, tm_mday=11, tm_hour=0, tm_min=37, tm_sec=24, tm_wday=6, tm_yday=255, tm_isdst=0) # 注:传入参数转换成相应的时间格式未传参则以当前时间为准 5.结构化时间,格式换时间,时间戳相互转换 print(time.localtime(time.time())) # 时间戳 -》结构化时间 time.struct_time(tm_year=2019, tm_mon=7, tm_mday=18, tm_hour=19, tm_min=0, tm_sec=37, tm_wday=3, tm_yday=199, tm_isdst=0) res = time.localtime(time.time()) # print(time.mktime(res)) # 结构化时间 -》时间戳 1563447665.0 print(time.strftime('%Y-%m',time.localtime())) # 结构换时间—》格式化时间 2019-07 print(time.strptime(time.strftime('%Y-%m',time.localtime()),'%Y-%m')) # 格式换时间—》结构换时间 time.struct_time(tm_year=2019, tm_mon=7, tm_mday=1, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=0, tm_yday=182, tm_isdst=-1)

''' %y 两位数的年份表示(00-99) %Y 四位数的年份表示(000-9999) %m 月份(01-12) %d 月内中的一天(0-31) %H 24小时制小时数(0-23) %I 12小时制小时数(01-12) %M 分钟数(00=59) %S 秒(00-59) %a 本地简化星期名称 %A 本地完整星期名称 %b 本地简化的月份名称 %B 本地完整的月份名称 %c 本地相应的日期表示和时间表示 %j 年内的一天(001-366) %p 本地A.M.或P.M.的等价符 %U 一年中的星期数(00-53)星期天为星期的开始 %w 星期(0-6),星期天为星期的开始 %W 一年中的星期数(00-53)星期一为星期的开始 %x 本地相应的日期表示 %X 本地相应的时间表示 %Z 当前时区的名称 %% %号本身 '''

import datetime print(datetime.date.today()) # date>>>:年月日 2019-07-18 print(datetime.datetime.today()) # datetime>>>:年月日 时分秒 2019-07-18 20:09:32.516456 res = datetime.date.today() res1 = datetime.datetime.today() print(res.year) print(res.month) print(res.day) print(res.weekday()) # 0-6表示星期 0表示周一 print(res.isoweekday()) # 1-7表示星期 7就是周日 """ (******) 日期对象 = 日期对象 +/- timedelta对象 timedelta对象 = 日期对象 +/- 日期对象 """ current_time = datetime.date.today() # 日期对象 print(current_time) # 2019-07-18 timetel_t = datetime.timedelta(days=7) # timedelta对象 print(timetel_t) # 7 days, 0:00:00 res1 = current_time+timetel_t # 日期对象 print(res1) # 2019-07-25 # print(current_time - timetel_t) # 2019-07-11 print(res1-current_time) # 7 days, 0:00:00
扫描二维码关注公众号,回复:
6820753 查看本文章
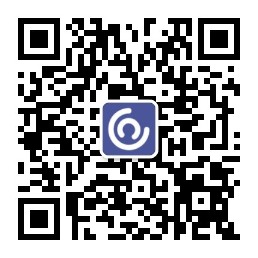

1.随机取一个你提供的整数范围内的数字;包含首尾 # print(random.randint(1,6)) 2.随机取一个你提供的整数范围内的数字;顾头不顾尾 # print(random.randrange(1, 10)) # 注意与random.randint的区别不包括结束值 3. 随机取一个0-1之间小数 # print(random.random()) 4. 摇号 随机从列表中取一个元素random.choice(item) # print(random.choice([1,2,3,4,5,6])) # print(random.choice((1, 4, 3,7,2,7,10))) 5.洗牌单列集合:random.shuffle() res = [1,2,3,4,5,6] random.shuffle(res) # 洗牌,元组不可以 print(res) 6.例:# 生成随机验证码 """ 大写字母 小写字母 数字 5位数的随机验证码 chr random.choice 封装成一个函数,用户想生成几位就生成几位 """ def get_code(num): info = '' for i in range(num): d = random.randint(65,90) # 大写字母 x = random.randint(97,122) # 小写字母 n = random.randint(0,9) # 数字 info += random.choice([chr(d),chr(x),str(n)]) # 随机选取一个字符串拼接 return info res = get_code(4) print(res)

os模块和sys模块的区别:
# os模块:跟操作系统打交道的模块
# sys模块:跟python解释器打交道模块

# 1.生成单级目录:os.mkdir('dirname') import os os.mkdir('abc') # 在当前所执行文件所在文件夹下创建abc文件夹 os.mkdir('D:\\abc') # 就是在指定的绝对路径下创建abc文件夹 os.mkdir('a/b/c') # a,b必须提前存在,c不能存在 # 2.生成多层目录:os.makedirs('dirname1/.../dirnamen2') os.makedirs(r'a\b\c') # 在所执行文件的文件夹下 a,b存在与否都可以,c不能存在 os.makedirs(r'D:\a\b\c') # 也可以指定到某个盘符下创建 # 3.重命名:os.rename("oldname","newname") os.rename("a", "a1")#将执行文件同目录下的a文件命名为a1文件 4.工作目录:os.getcwd() print(os.getcwd()) # 获取当前所执行文件所在的文件夹路径 5.删除单层空目录:os.rmdir('dirname') os.rmdir('aa/b/c') #删除文件夹c; 删除单级空目录,若目录不为空则无法删除,报错 6.移除多层空目录:os.removedirs('dirname1/.../dirnamen') os.removedirs('a1/b') # 删除a1和a1下的空文件;若目录为空,则删除,并递归到上一级目录,如若也为空,则删除,依此类推 7.列举目录下所有资源:os.listdir('dirname')列出指定目录下的所有文件夹和子目录,包括隐藏文件,并以列表方式打印 print(os.listdir(r'E:\Python脱产8期课堂内容\day18\代码\part4')) #['aa', 'abc', 'os模块.py'] 8.路径分隔符:os.sep 9.行终止符:os.linesep 10.文件分隔符:os.pathsep 11.操作系统名:os.name 12.操作系统环境变量:os.environ 13.执行shell脚本:os.system() 14.os.chdir(r'D:\Python项目\day16\老师们的作品') # 切换当前所在的目录

1.执行文件的当前路径:__file__ print(__file__) # 打印查看当前执行文件的绝对路径(包括执行文件自身) 2.返回path规范化的绝对路径:os.path.abspath(path) res = os.path.abspath(r'F:/python8期\课堂内容/day18\\代码\part5\\os_path.py') print(res)#F:\python8期\课堂内容\day18\代码\part5\os_path.py 3.将path分割成目录和文件名二元组返回:os.path.split(path) res = os.path.split(r'F:\python8期\课堂内容\day18\代码\part5\os_path.py') print(res, res[1]) # ('F:\\python8期\\课堂内容\\day18\\代码\\part5', 'os_path.py') os_path.py print(r'F:\python8期\课堂内容\day18\代码\part5\os_path.py'.rsplit(os.sep, 1)) # ['F:\\python8期\\课堂内容\\day18\\代码\\part5', 'os_path.py'] 4.返回上一级目录:os.path.dirname(path) print(__file__) print(os.path.dirname(__file__)) print(os.path.dirname(os.path.dirname(__file__))) 结果: E:/Python脱产8期课堂内容/day18/代码/part5/os_path.py E:/Python脱产8期课堂内容/day18/代码/part5 E:/Python脱产8期课堂内容/day18/代码 注:可以嵌套使用 5.最后一级名称:os.path.basename(path) print(os.path.basename(r'F:\python8期\课堂内容\day18\代码\part5')) # part5 6.判断文件夹和文件是否存在:os.path.exists(path) print(os.path.exists(r'E:\python10期\day16 模块collection time random os sys json picke subpross\代码\day16\老师们的作品')) # 判断文件是否存在 print(os.path.exists(r'E:\python10期\day16 模块collection time random os sys json picke subpross\代码\day16\老师们的作品\tank老师.txt')) # 判断文件是否存在 # print(os.path.isfile(r'D:\Python项目\day16\tank老师精选')) # 只能判断文件 不能判断文件夹 7.目标大小:os.path.getsize(path) print(os.path.getsize(r'D:\Python项目\day16\老师们的作品\tank老师.txt')) # 字节大小 8.路径拼接:os.path.join(path1[, path2[, ...]])

import sys # sys.path.append() # 将某个路径添加到系统的环境变量中 # print(sys.platform) # print(sys.version) # python解释器的版本

# 序列化:将对象转换为字符串 # dumps:将对象直接序列化成字符串 # dump:将对象序列化成字符串存储到文件中 obj = {'name': 'Owen', "age": 18, 'height': 180, "gender": "男"} r1 = json.dumps(obj, ensure_ascii=False) # 取消默认ascii编码,同该文件的编码 utf-8 py3默认,py2规定文件头 print(r1) # {"name": "Owen", "age": 18, "height": 180, "gender": "男"} with open('1.txt', 'w', encoding='utf-8') as wf: json.dump(obj, wf, ensure_ascii=False) # 反序列化:将字符串转换为对象 # loads:将字符串直接序列化成对象 # load:将字符串从文件中读出直接序列化成对象 json_str = '{"name": "Owen", "age": 18, "height": 180, "gender": "男"}' r2 = json.loads(json_str, encoding='utf-8') # 默认跟当前文件被解释器执行的编码走 print(r2, type(r2)) # {'name': 'Owen', 'age': 18, 'height': 180, 'gender': '男'} <class 'dict'> with open('1.txt', 'r', encoding='utf-8') as rf: r3 = json.load(rf) print(r3, type(r3))

import pickle obj = {"name": 'Owen', "age": 18, "height": 180, "gender": "男"} # 序列化 r1 = pickle.dumps(obj) print(r1) # 将对象直接转成二进制 b'\x80\x03}q\x00(X\x04\x00\x00\x00nameq\x01X\x04\x00\x00\x00Owenq\x02X\x03\x00\x00\x00ageq\\x94\xb7q\x06u.' # 反序列化 res1 = pickle.loads(r1) print(res1,type(res1)) # {'name': 'Owen', 'age': 18, 'height': 180, 'gender': '男'} <class 'dict'> """ 用pickle操作文件的时候 文件的打开模式必须是b模式 """ # with open('userinfo_1','wb') as f: # pickle.dump(d,f) # with open('userinfo_1','rb') as f: # res = pickle.load(f) # print(res,type(res))

import subprocess order = subprocess.Popen('终端命令', shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE) suc_res = order.stdout.read().decode('系统默认编码') err_res = order.stderr.read().decode('系统默认编码') order = subprocess.run('终端命令', shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE) suc_res = order.stdout.decode('系统默认编码') err_res = order.stderr.decode('系统默认编码')