springmvc实现文件上传
多数文件上传都是通过表单形式提交给后台服务器的,因此,要实现文件上传功能,就需要提供一个文件上传的表单,而该表单就要满足以下3个条件
(1)form表彰的method属性必须设置为post提交
(2)form表单的enctype属性设置为multipart/form-data
(3) 提供<input type="file" name ="filename" />的文件上传输入框
文件上传表单的示例代码如下:
<form action="/fileUpload.do" method="post" enctype="multipart/form-data"> 上传人:<input id="name" type="text" name="name" /> <br /> 请选择文件:<inputid="file" type="file" name="filename" multiple="multiple" /> <br /> <input type="submit" value="上传" /> </form>
其中的 multiple="multiple" 可以实现多文件上传
还需要要springmvc.xml文件中配置文件上传解析器
<!--配置文件上传解析器 --> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- 设置请求编码格式,必须与JSP中的pageEncoding属性一致,默认为iso-8859-1 --> <property name="defaultEncoding" value="UTF-8" /> <!-- 设置允许上传文件的最大值(2MB) ,单位为字节 --> <property name="maxUploadSize" value="2097152"></property> </bean>
下面通过一个例子来说明
一个web.xml文件
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
id="WebApp_ID" version="3.1"> <display-name>testupload</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <!--配置前端控制器--> <servlet> <servlet-name>upload</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:springmvc.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>upload</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
扫描二维码关注公众号,回复:
68063 查看本文章
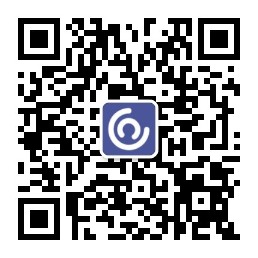
一个springmvc.xml文件
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- 扫描的Controller包 --> <context:component-scan base-package="cn.lzc.upload.controller" /> <!--配置注解驱动 --> <mvc:annotation-driven /> <!--配置视图解析器 --> <bean id="internalResourceViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp/" /> <property name="suffix" value=".jsp" /> </bean> <!--配置文件上传解析器 --> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- 设置请求编码格式,必须与JSP中的pageEncoding属性一致,默认为iso-8859-1 --> <property name="defaultEncoding" value="UTF-8" /> <!-- 设置允许上传文件的最大值(2MB) ,单位为字节 --> <property name="maxUploadSize" value="2097152"></property> </bean> </beans>
页面fileUpload.jsp文件
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>文件上传</title> <script> // 判断是否填写上传人并已选择上传文件 function check(){ var name = document.getElementById("name").value; var file = document.getElementById("file").value; if(name==""){ alert("填写上传人"); return false; } if(file.length==0||file==""){ alert("请选择上传文件"); return false; } return true; } </script> </head> <body> <form action="${pageContext.request.contextPath }/fileUpload.do" method="post" enctype="multipart/form-data" onsubmit="return check()"> 上传人:<input id="name" type="text" name="name" /><br /> 请选择文件:<input id="file" type="file" name="uploadfile" multiple="multiple" /><br /> <input type="submit" value="上传" /> </form> </body> </html>
一个FileUploadController.class文件
package cn.lzc.upload.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.multipart.MultipartFile; import javax.servlet.http.HttpServletRequest; import java.io.File; import java.util.List; import java.util.UUID; /** * 文件上传Controller */ @Controller public class FileUploadController { /** * 文件上传的方法 */ @RequestMapping("/fileUpload.do") public String handleFormUpload(@RequestParam("name")String name, @RequestParam("uploadfile") List<MultipartFile> uploadfile, HttpServletRequest request) { //判断所上传文件是否存在 if (!uploadfile.isEmpty() && uploadfile.size() > 0) { //循环输出上传的文件 for (MultipartFile file : uploadfile) { //获取上传文件原始名称 String originalFilename = file.getOriginalFilename(); //设置上传文件的保存地址目录 String savePath = request.getServletContext().getRealPath("/upload/"); File filePath = new File(savePath); //如果文件的地址不存在,就创建一个目录 if (!filePath.exists()) { filePath.mkdirs(); } //使用UUID重样命名上传的文件名称 String newFileName = name + "_" + UUID.randomUUID() + "_" + originalFilename; try { //使用MultipartFile接口的方法寒碜文件上传到指定位置 file.transferTo(new File(savePath + newFileName)); } catch (Exception e) { e.printStackTrace(); return "error"; } } //跳转到成功页面 return "success"; } else { return "error"; } } }
正确提交页面 succeess.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 文件上传成功! </body> </html>
错误页面error.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 文件上传失败,请重新上传! </body> </html>
按过程来就可以了