对于python而言,一切事物都是对象,对象是基于类创建的,对象继承了类的属性,方法等特性
一.int
首先我们来查看一下int包含了哪些函数
# python3.x dir(int) # ['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes'] # python 2.x dir(int) # ['__abs__', '__add__', '__and__', '__class__', '__cmp__', '__coerce__', '__delattr__', '__div__', '__divmod__', '__doc__', '__float__', '__floordiv__', '__format__', '__getattribute__', '__getnewargs__', '__hash__', '__hex__', '__index__', '__init__', '__int__', '__invert__', '__long__', '__lshift__', '__mod__', '__mul__', '__neg__', '__new__', '__nonzero__', '__oct__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdiv__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'bit_length', 'conjugate', 'denominator', 'imag', 'numerator', 'real']


# __abs__() 绝对值输出 num = 1 result = num.__abs__() print(result) num = -1 result = num.__abs__() print(result)


1 num = -1 2 result = num.__add__(2) 3 4 print(result) 5 6 # 打印结果将输出 1


1 num = 5 2 result = num.__and__(2) 3 print(result) 4 5 #打印输出为0 6 # 0 0 0 0 0 1 0 1 5 7 # 0 0 0 0 0 0 1 0 2 8 #相同位为1则为1,由于没有相同位,所以5 & 2结果为0


1 # 以下结果输出都是True 2 num = 11 3 print(num.__bool__()) # True 4 5 num = -11 6 print(num.__bool__()) #True 7 8 # 以下结果输出都是 False 9 num = 0 10 print(num.__bool__()) #False 11 12 num = None 13 num = False 14 print (num.__bool__()) # False


#通过divmod函数可以实现将一个int类型对象除以另一个int对象得到一个两个元素的列表, #列表左边为除尽取整的值,第二个元素为取模的余数 num = 9 result = num.__divmod__(2) print(result) #输出(4,1)


1 num = 2 2 result = num.__eq__(3) 3 print(result) 4 5 #打印结果为False 6 #2 == 3 结果为假 7 8 result = num.__eq__(2) 9 print(result) 10 #打印结果为True 11 # 2 == 2 结果为真


1 num = 9 2 print(num.__float__()) 3 4 #打印结果为 9.0


1 num = int(181) 2 result = num.__floordiv__(9) 3 print(result) 4 5 #打印输出20 6 #地板除 //取整


num = int(181) result = num.__getattribute__("bit_length") print(result) #打印输出 <built-in method bit_length of int object at 0x100275020> #说明该数据类型num存在bit_length这个属性,可以用于判断对象是否拥有某种属性


1 num = int(181)
2 print(num.__ge__(111))
3 #打印输出结果为True
4 #因为181大于111,所以结果为真,该属性用于判断大于等于该属性自身的方法,结果将返回真,否则为假


1 num = 181 2 print(int.__invert__(num)) 3 #打印输出-182 4 5 num = -180 6 print(int.__invert__(num)) 7 #打印输出179 8 9 num = -181 10 print(int.__invert__(num)) 11 #打印输出180


1 num = -181 2 result = num.__le__(111) 3 print(result) 4 #打印输出结果为True 5 #当传人参数与对象本身相比较,只要对象小于或者等于传人的参数,则结果为真,否则为假


1 num = -181 2 3 result = num.__lshift__(1) 4 print(result) 5 #打印输出结果为-362 ,即-181 *( 2**1) 6 7 result = num.__lshift__(2) 8 print(result) 9 #打印输出结果为-724 ,即-181*(2**2) 10 11 12 #当传入参数大于等于0时且对象本身不能为0,首先参数本身为2的指数幂运算,然后再与对象本身相乘结果则为左移最终结果


1 num = -181 2 print(num.__lt__(11)) 3 4 #打印输出结果为True 5 6 #凡是对象比传入的参数小,则结果为真,否则结果为假


1 num = -181 2 print(num.__mod__(3)) 3 4 #打印输出结果为2,因为-181除以3等于60,余数为2,所以结果为2


1 num = 181
2 print(num.__mul__(2))
3
4 #打印输出结果为362,即181*2的结果


1 num = -181 2 print(int.__neg__(num)) 3 4 #打印结果为181,即-(-181),结果为181


1 num = 181 2 print(num.__ne__(181)) 3 #打印结果为False 4 5 print(num.__ne__(11)) 6 #打印结果为True 7 8 #凡是传入参数与对象本身不相等,则结果为真,否则为假


1 num = 18 2 print(num.__or__(7)) 3 4 #打印输出结果为23 5 # 0 0 0 1 0 0 1 0 18 6 # 0 0 0 0 0 1 1 1 7 7 # 0 0 0 1 0 1 1 1 23 8 位的或运算,凡是相同位有一位为真,即为1,则结果为真,即1,然后所以最终结果为23


1 num = 9 2 print(num.__pow__(2)) 3 #打印输出结果为81,即9**2


1 num = 6 2 print(num.__rdivmod__(3)) 3 #返回结果(0,3) 左边为余数,右边为整除的结果


1 #python 2.7 2 num = 1 3 print(num.__sizeof__()) 4 #打印输出结果为24个字节,说明一个int类型默认就在内存中占用了24个字节大小 5 6 #python3.5 7 num = 1 8 print(num.__sizeof__()) 9 #打印输出结果为28个字节,说明一个int类型数据默认在内存中占用了24个字节大小


1 num = int(1111) 2 result = num.__str__() 3 print(type(result)) 4 5 #打印输出结果为<class 'str'> 6 #将int类型转换为str数据类型


1 num = int(9) 2 print(num.__sub__(2)) 3 4 #打印输出结果为7 5 #对象本身减去传入参数,得到最终的返回值


1 num = 11 2 print(num.__truediv__(3)) 3 4 #打印输出结果为3.6666666666666665 5 #返回的数据类型为float,浮点型


1 num = 10 2 print(num.__xor__(6)) 3 4 # 0 0 0 0 1 0 1 0 10 5 # 0 0 0 0 0 1 1 0 6 6 # 0 0 0 0 1 1 0 0 12 7 8 #同位比较,都是0则为假,都是1则为假,一真一假为真


1 num = 5 2 print(num.bit_length()) 3 #打印输出结果为3 4 5 # 0 0 0 0 0 1 0 1 #长度为3位


1 num = 2.3 - 2.5j 2 result = num.real #复数的实部 3 print(result) #打印输出2.3 4 result = num.imag #复数的虚部 5 print(result) #打印输出2.5j 6 7 result = num.conjugate() #返回该复数的共轭复数 8 print(result) #打印输出(2.3+2.5j)


1 num = 5 2 print(num.__format__("20")) 3 #表示5前面讲话有20个空格


1 print(int.from_bytes(bytes=b'1', byteorder='little') 2 3 4 #打印输出 49 ,即将字符1转换为十进制


1 num = 2 2 result = num.to_bytes(5,byteorder='little') 3 print(result) 4 #打印输出b'\x02\x00\x00\x00\x00' 5 for i in result: 6 print(i) 7 8 9 #打印输出2\n0\n0\n0\n0 10 #\n表示回车
二.str
1 #python3.5 2 dir(str) 3 #['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__',
'__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__iter__',
'__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__',
'__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__',
'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find', 'format',
'format_map', 'index', 'isalnum', 'isalpha', 'isdecimal', 'isdigit', 'isidentifier', 'islower',
'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip',
'maketrans', 'partition', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip',
'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill'] 4 5 #python2.7 6 dir(str) 7 #['__add__', '__class__', '__contains__', '__delattr__', '__doc__', '__eq__', '__format__',
'__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__getslice__', '__gt__',
'__hash__', '__init__', '__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__',
'__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmod__', '__rmul__', '__setattr__',
'__sizeof__', '__str__', '__subclasshook__', '_formatter_field_name_split', '_formatter_parser',
'capitalize', 'center', 'count', 'decode', 'encode', 'endswith', 'expandtabs', 'find', 'format',
'index', 'isalnum', 'isalpha', 'isdigit', 'islower', 'isspace', 'istitle', 'isupper', 'join',
'ljust', 'lower', 'lstrip', 'partition', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition',
'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate',
'upper', 'zfill']


1 strA = "hello" 2 print(strA.__add__(" world")) 3 #输出hello world


1 strA = "hello" 2 print(strA.__contains__("h")) 3 #True 4 5 print(strA.__contains__('hex')) 6 #False


1 strA = "hello" 2 print(strA.__eq__('hello')) 3 #True 4 5 print(strA.__eq__('hellq')) 6 #False


1 strA = "hello" 2 print(strA.__getattribute__('__add__')) 3 #<method-wrapper '__add__' of str object at 0x101d78730> 4 5 #判断对象是否包含传入参数的属性


1 strA = "hello" 2 print(strA.__getitem__(0)) 3 #输出下标为0的字符 h 4 #超出下标会报错的


1 strA = "hello" 2 print(strA.__getnewargs__()) 3 #打印输出 ('hello',) 4 #将字符类型转换为元组方式输出


1 strA = "hello" 2 print(strA.__ge__('HELLO')) 3 print(strA.__ge__('Hello')) 4 print(strA.__ge__('hello')) 5 #以上结果都为True, 6 print(strA.__ge__('hellq')) 7 #以上结果为假


1 strA = 'Hello' 2 print(strA.__gt__('HellO')) 3 #打印输出True 4 5 #字符串比较的是传入的参数每个字符首先得包含对象,其次如果字符串之间比较,大写比小写大,,如果传入参数都为大写,且对象也都为大写,那么结果为假,字符串比较首先比较的是字符串是否相同,不相同则为假,再次每个字符进行比较,只要前面有一位大于对方,则不继续比较了 6 7 8 #比如 HelLo与HEllo,首先两个字符串都是一样的,然后再比较第一位,第一位也一样,再比较第二位,大写比小写大,所以第二个字符串大,就不会继续比较下去了


1 strA = "hello" 2 print(strA.__hash__()) 3 #-7842000111924627789


1 strA = "hello" 2 result = strA.__iter__() 3 for i in result: 4 print(i) 5 6 #打印输出 7 #h 8 #e 9 #l 10 #l 11 #o


1 strA = 'hello' 2 print(strA.__len__()) 3 4 #打印输出结果为5


1 strA = 'Hello' 2 print(strA.__le__('ello')) 3 #True 4 5 6 #字符串小于运算比较,先比较对象是否包含传入参数,当包含则再比较相同位的字母,大小字母比小写字母大,当前面有一位比较出谁大谁小了,则不再继续比下去了


1 strA = 'hello' 2 print(strA.__lt__('ello')) 3 #True 4 5 #字符串小于比较与小于等于比较类似,唯一一点是小于比较时,对象与传入的参数大小写不能完全一样


1 strA = 'hello' 2 print(strA.__mul__(3)) 3 #hellohellohello 4 5 6 #打印结果将输出三个hello


1 strA = "hello" 2 3 print(strA.__ne__('HEllo')) 4 #True 5 6 7 #字符串不等于运算比较,凡是对象与传入参数只要有一个字母大小写不一样则为真,否则为假


1 strA = "HELLO" 2 print(strA.zfill(6)) 3 #0HELLO 4 5 6 #当传入的参数长度比对象长度大时,多余的长度则以0进行填充


1 strA = "hELlo1112123"
2 print(strA.upper())
3 #HELLO
4
5 #将所有的字母转换为大写


1 print("hello world".title())
2
3 #Hello World
4
5 #每个单词首字母大写输出,且单词的第二位后面都会变成小写,如helLO,最终会格式化为Hello


1 print("hEllO".swapcase())
2 #HeLLo
3
4 #将原来的大小字母转换成小写字母,小写转换成大小字母


1 print(" hello world ".strip())
2 #hello world
3 #将字符串两边的空格去掉


1 print("hello".startswith('h'))
2 #True
3
4 print("hello".startswith('h',1))
5 #False
6
7 #startswith这个函数可以指定起始位置进行判断字符是否存在


1 print("hello\nworld".splitlines()) 2 #['hello','world'] 3 4 #splitlines默认以\n换行符进行分割字符,最终返回一个列表
分割,只能根据,true,false;是否保留换行


1 print("hello world".split())
2 #['hello','world']
3
4 print("hello world".split('\n'))
5 #['hello world',]
6
7 #默认以空格分割字符串,可以指定分隔符


1 print(" hello world ".rstrip()) 2 3 # hello world 4 #打印将会把world后面的空格去除


1 print("hello world".rpartition('he'))
2 #('', 'he', 'llo world ')
3 #只返回传入参数且存在字符串里的字符然后组合成一个新的元组


1 print("hello world".rjust(20)) 2 # hello world 3 #默认以空格填充,从左到最后一个单词d结尾一个长度为20,也就是说h前面有9个空格 4 5 print("hello world".rjust(20,'+')) 6 #+++++++++hello world 7 #这里以‘+’填充,对比上面,可以看的更具体,前面有9个+被用来填充


1 print("hello world".rindex('wo')) 2 #6 3 #通过查找字符串'wo'获取该字符串在hello world 里面的下标位置,这里从左往右数,第七个位置,字符串的下标默认从0开始,所以返回6 4 #当找不到时则抛出异常

1 print("hello world".rindex('wo')) 2 #6 3 #通过查找字符串'wo'获取该字符串在hello world 里面的下标位置,这里从左往右数,第七个位置,字符串的下标默认从0开始,所以返回6 4 #当找不到时则抛出

1 print("hello world".rindex('wo')) 2 #6 3 #通过查找字符串'wo'获取该字符串在hello world 里面的下标位置,这里从左往右数,第七个位置,字符串的下标默认从0开始,所以返回6 4 #当找不到时则抛

1 print("hello world".rindex('wo')) 2 #6 3 #通过查找字符串'wo'获取该字符串在hello world 里面的下标位置,这里从左往右数,第七个位置,字符串的下标默认从0开始,所以返回6 4 #当找不到时则抛

1 print("hello world".rindex('wo')) 2 #6 3 #通过查找字符串'wo'获取该字符串在hello world 里面的下标位置,这里从左往右数,第七个位置,字符串的下标默认从0开始,所以返回6 4 #当找不到时则抛出


1 strA = 'hello 123' 2 table1 = str.maketrans('123','我很好') 3 print(strA.translate(table1)) 4 #hello 我很好 5 6 #将字符串里面的123通过table进行翻译成对应的值,table1的123长度必须和‘我很好长度对应’ 7 8 9 strA = 'hello 12' 10 table1 = str.maketrans('123','我很好') 11 print(strA.translate(table1)) 12 #hello 我很


1 print("hello".rfind('e')) 2 #1 3 4 print("hello".rfind('ee')) 5 #-1 6 7 如果找到,则结果为对应的下标,否则返回-1


1 print('hello world'.replace('e','o')) 2 #hollo world 3 #将字符串里面所有的e替换成o,区分大小写


1 print('hello world'.rpartition('el'))
2 #('h', 'el', 'lo world')
3 #效果与rpartition相似


1 table1 = str.maketrans('123','我很好') 2 print(table1) 3 #{49: 25105, 50: 24456, 51: 22909} 4 #首先传入的必须是两个参数,且长度相等 5 #返回结果将是一个字典类型,每一个字符串将会映射到第二个参数的相同位置的字符串上, 6 #当这里存在三个参数时,第三个参数必须是一个字符串类型,且整个字符串将被映射成None 7 8 strA = 'hello 1233飒飒' 9 table1 = str.maketrans('123','我很好','飒飒') 10 print(strA.translate(table1)) 11 print(table1) 12 13 #以下为输出结果 14 #hello 我很好好 15 #{49: 25105, 50: 24456, 51: 22909, 39122: None} 16 17 #这个字典的值将被映射成unicode值,如49表示unicode的1


1 table1 = str.maketrans('123','我很好')
2 print(table1)
3 #{49: 25105, 50: 24456, 51: 22909}
4 #首先传入的必须是两个参数,且长度相等
5 #返回结果将是一个字典类型,每一个字符串将会映射到第二个参数的相同位置的字符串上,
6 #当这里存在三个参数时,第三个参数必须是一个字符串类型,且整个字符串将被映射成None
7
8 strA = 'hello 1233飒飒'
9 table1 = str.maketrans('123','我很好','飒飒')
10 print(strA.translate(table1))
11 print(table1)
12
13 #以下为输出结果
14 #hello 我很好好
15 #{49: 25105, 50: 24456, 51: 22909, 39122: None}
16
17 #这个字典的值将被映射成unicode值,如49表示unicode的1


1 table1 = str.maketrans('123','我很好')
2 print(table1)
3 #{49: 25105, 50: 24456, 51: 22909}
4 #首先传入的必须是两个参数,且长度相等
5 #返回结果将是一个字典类型,每一个字符串将会映射到第二个参数的相同位置的字符串上,
6 #当这里存在三个参数时,第三个参数必须是一个字符串类型,且整个字符串将被映射成None
7
8 strA = 'hello 1233飒飒'
9 table1 = str.maketrans('123','我很好','飒飒')
10 print(strA.translate(table1))
11 print(table1)
12
13 #以下为输出结果
14 #hello 我很好好
15 #{49: 25105, 50: 24456, 51: 22909, 39122: None}
16
17 #这个字典的值将被映射成unicode值,如49表示unicode的1


1 table1 = str.maketrans('123','我很好')
2 print(table1)
3 #{49: 25105, 50: 24456, 51: 22909}
4 #首先传入的必须是两个参数,且长度相等
5 #返回结果将是一个字典类型,每一个字符串将会映射到第二个参数的相同位置的字符串上,
6 #当这里存在三个参数时,第三个参数必须是一个字符串类型,且整个字符串将被映射成None
7
8 strA = 'hello 1233飒飒'
9 table1 = str.maketrans('123','我很好','飒飒')
10 print(strA.translate(table1))
11 print(table1)
12
13 #以下为输出结果
14 #hello 我很好好
15 #{49: 25105, 50: 24456, 51: 22909, 39122: None}
16
17 #这个字典的值将被映射成unicode值,如49表示unicode的1


1 print(" hello world ".lstrip())
2 #hello world
3 将hello左边空格去除


1 print("HELLo22".lower())
2 #hello22
3 #将所有字母转换为小写


1 print("hello world".ljust(20,'+')) 2 #hello world+++++++++ 3 #从右向左开始进行填充,总长度为20


print('+'.join(('hello','world'))) #hello+world #通过一个字符串去与join里面的一个迭代器里的字符串进行联结生存一个新的字符串


1 test = "无所谓,谁会爱上谁!"
2 t=' '
3 v=t.join(test)
4 print(v)
将字符串中的每一个元素按照指定分隔符进行拼接


print('Hello'.isupper())
print('HELLO1'.isupper())
#False
#True
#判断所有的字母是否都是大小,是则返回真,否则假


1 print('Hello'.istitle())
2 print('Hello world'.istitle())
3 #True
4 #False
5 #判断每个单词首字母是否大写,是则为真,否则为假


1 print(' hello'.isspace())
2 print(' '.isspace())
3 #False
4 #True
5 #判断内容是否为空格


1 print('hello world'.isprintable()) 2 print('\n'.isprintable()) 3 #True 4 #False 5 #由于换行符是特殊字符,不可见,所以不能被打印,结果为假
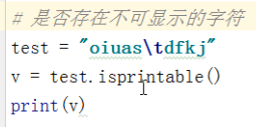


1 print('111'.isnumeric()) 2 print('壹'.isnumeric()) 3 print('1q'.isnumeric()) 4 #True 5 #True 6 #False 7 #True包含unicode数字,全角数字(双字节),罗马数字,汉字数字


1 print('Hello'.islower())
2 print('hello'.islower())
3 #False
4 #True
5 #判断字母是不是都是小写,是则为真,否则为假


1 print('def'.isidentifier())
2 print('hello'.isidentifier())
3 print('2a2'.isidentifier())
4 #True
5 #True
6 #False
7
8 #用来检测标识符是否可用,也就是说这个名字能不能用来作为变量名,是否符合命名规范,如果符合则为真
9 #通常会结合keyword.iskeyword()这个方法去在做判断是否是关键字,防止因命名不规范导致某些内置功能不可用


1 print('hello'.isdigit())
2 print('111e'.isdigit())
3 print('壹'.isdigit())
4 print('121'.isdigit())
5
6 #False
7 #False
8 #False
9 #True
10
11 #unicode数字,全角数字(双字节),byte数字,罗马数字都为真


1 print('11'.isdecimal())
2 print('壹'.isdecimal())
3 print('11d'.isdecimal())
4 #
5 #True
6 #False
7 #False
8 #只有全部为unicode数字,全角数字(双字节),结果才为真


1 print('hee'.isalpha()) 2 print('Hello'.isalpha()) 3 print('1212'.isalpha()) 4 print('hhee1'.isalpha()) 5 #True 6 #True 7 #False 8 #False 9 #当结果都是字母则为真,否则为假


1 print('hew11'.isalnum())
2 print('HHH'.isalnum())
3 print('112'.isalnum())
4 print(' q '.isalnum())
5 print('!!@~d'.isalnum())
6 #True
7 #True
8 #True
9 #False
10 #False
11
12 #当结果为任意数字或字母时,结果为真,其他字符为假


1 print('hello'.index('e'))
2 print('hello'.index('el'))
3 print('hello'.index('el',1))
4 #1
5 #1
6 #1
7 #通过查找制定的字符获取对应字符串的下标位置,可以指定起始位置,第3个事咧则表示从下标1开始查找,包括下标1的位置,如果指定end的结束位置,查找是不包括end的位置本身


1 print('hello'.find('h',0)) 2 print('hello'.find('h',1)) 3 #0 4 #-1 5 6 #find是从下标0位置开始找起,包含开始的位置0,如果有结束的位置,不包含结束位置,查找到则显示具体下标位置,否则显示-1


1 print('hello{0}'.format(' world')) 2 print('hello{0}{1}'.format(' world',' python')) 3 print('hello{name}'.format(name=' world')) 4 #hello world 5 #hello world python 6 #hello world


1 print('hello\tworld'.expandtabs(tabsize=8)) 2 #hello world 指定制表符长度为8

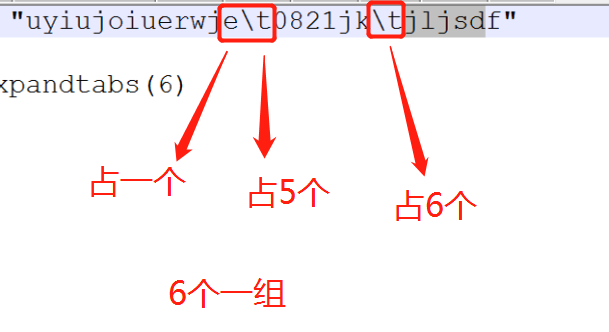


1 print('hello'.endswith('lo',3))
2 #True
3 #判断结束字符是否为lo,默认从下标0开始查找,包含下标0的位置


1 print('我好'.encode()) 2 print('hello'.encode()) 3 # print('hela!~@@~!\xe2lo'.encode('gbk',errors='strict')) 4 print(b'\xe6\x88\x91\xe5\xa5\xbd'.decode('utf-8')) 5 6 #b'\xe6\x88\x91\xe5\xa5\xbd' 7 #b'hello' 8 #我好 9 10 11 #将字符串进行编码,最终返回以b开头的编码格式


1 print('heelloe'.count('e',1,2)) 2 #1 3 #表示从开始下标1包括下标1位置查找字符e,结束位置为下标2,不包括结束位置 4 #统计结果为1


1 print('aaa'.center(22,'+')) 2 #+++++++++aaa++++++++++ 3 #表示将字符aaa的位置显示在长度为22的中间位置,默认是空格方式填充,这里以+号填充方便演示效果,注意,由于22-3(字符本身3个长度),剩余的并不能整除,所以先整除的整数部分作为公共的填充内容,剩余的填充到末尾


1 print('hDasdd23ellAo'.casefold())
2 #hdasdd23ellao
3
4 #将字符串里面所有的字母都转换为小写输出


1 print('hEello World'.capitalize())
2
3 #Heello world
4 #这个方法会将整个字符串的第一个字母大写,其余都是小写输出,如果第一个字符串不是字母,则只将其余字母转换成小写
三.dict
1 #python3.5 2 dir(dict) 3 #['__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'items', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values'] 4 5 #python2.x 6 dir(dict) 7 #['__class__', '__cmp__', '__contains__', '__delattr__', '__delitem__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'has_key', 'items', 'iteritems', 'iterkeys', 'itervalues', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values', 'viewitems', 'viewkeys', 'viewvalues']


1 food = {'1':'apple','2':'banana'} 2 print(food) 3 food.clear() 4 print(food) 5 6 #{'2': 'banana', '1': 'apple'} 正常打印结果 7 #{} 调用字典函数clear打印结果


1 food = {'1':'apple','2':'banana'} 2 newfood = food.copy() 3 print(newfood) 4 #{'1': 'apple', '2': 'banana'} 打印输出,


1 food = {'1':'apple','2':'banana'} 2 print(food.fromkeys(('w'),('2','5'))) 3 print(food) 4 5 #{'w': ('2', '5')} 6 #{'2': 'banana', '1': 'apple'} 7 8 #注意,这个操作并不会改变字典的数据值,仅仅是返回一个新字典


1 food = {'1':'apple','2':'banana'} 2 print(food.get('22')) 3 print(food.get('1')) 4 print(food.get('22','neworange')) 5 print(food.get('1','neworange')) 6 print(food) 7 8 #None 如果没有这个key将返回一个默认值none 9 #apple 如果能能查到key则显示对应的值 10 #neworange 如果查不到,则显示默认的值 11 #apple 如果能查到key,只显示key对应的值,否则使用默认的值 12 #{'1': 'apple', '2': 'banana'} get不会改变字典内容


1 food = {'1':'apple','2':'banana'} 2 print(food.items()) 3 4 #dict_items([('1', 'apple'), ('2', 'banana')]) 将字典的键存放在一个元组,对应的值也放在另一个元组里面,返回


1 food = {'1':'apple','2':'banana'} 2 print(food.keys()) 3 4 #dict_keys(['2', '1'])


1 food = {'1':'apple','2':'banana'} 2 result = food.pop('1') 3 print(result) 4 print(food) 5 6 #apple 将被删除的键对应的值返回 7 #{'2': 'banana'} 打印更新后的字典


1 food = {'1':'apple','2':'banana'} 2 print(food.popitem()) 3 print(food) 4 5 #('1', 'apple') 随机删除键值对 6 #{'2': 'banana'} 返回删除后的字典


1 food = {'1':'apple','2':'banana'} 2 print(food.setdefault('3','orange')) 3 print(food) 4 5 #orange 默认的值 6 #{'3': 'orange', '2': 'banana', '1': 'apple'} 打印字典


1 food = {'1':'apple','2':'banana'} 2 goods = {'1':'TV','22':'Computer'} 3 print(food.update(goods)) 4 print(food) 5 6 7 #None 8 #{'2': 'banana', '1': 'TV', '22': 'Computer'} 如果存在对应的key则更新value,否则新增键值对


1 food = {'1':'apple','2':'banana'} 2 print(food.values()) 3 4 5 #dict_values(['apple', 'banana'])
上下内容替换
转载于:https://www.cnblogs.com/dusihan/p/10130736.html