// main.cpp
// stack_quhao
// Created by duanqibo on 2019/6/29.
// Copyright © 2019年 duanqibo. All rights reserved.
// 顺序栈的操作,整数进栈,取栈顶元素,栈内剩余元素
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
const int maxsize=6;
/*
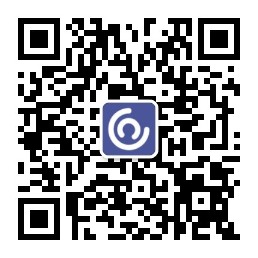
typedef struct student
{
int No;
char name[20];
char sex[2];
int age;
}DataType;
*/
typedef struct seqstack
{
int data[maxsize];
int top;
}SeqStk;
//栈初始化
int InitStack(SeqStk *stk)
{
stk->top=0;
return 1;
}
//判断是否显空栈
int EmptyStack(SeqStk *stk)
{
if(stk->top==0)
return 1;
else
return 0;
}
//入栈
int Push(SeqStk *stk,int x)
{
if(stk->top==maxsize-1)
{
printf("栈已满!");
return 0;
}
else
{
stk->top++;
stk->data[stk->top]=x;
return 1;
}
}
//出栈
int Pop(SeqStk *stk)
{
if(EmptyStack(stk))
{
printf("栈已空!");
return 0;
}
else
{
stk->top--;
return 1;
}
}
//取栈顶元素
int GetTop(SeqStk *stk)
{
if(EmptyStack(stk))
return 0;
else
return stk->data[stk->top];
}
void menu()
{
printf("*************************\n");
printf(" 1.进栈(-1结束)\n");
printf(" 2.输出栈顶元素\n");
printf(" 3.剩余栈元素\n");
printf(" 0.退出系统\n");
printf("*************************\n");
}
int main(int argc, const char * argv[])
{
SeqStk SK;
int n;
int ch;
InitStack(&SK);
menu();
while(1)
{
printf("请输入:");
scanf("%d",&ch);
switch(ch)
{
case 1:
//printf("\n---客户取号---\n");
scanf("%d",&n);
while(n!=-1)
//for(i=0;i<6;i++)
{
Push(&SK,n);
scanf("%d",&n);
}
break;
case 2:
if(!EmptyStack(&SK))
{
n=GetTop(&SK);
Pop(&SK);
printf("\n从栈中出来的数是:%d\n",n);
break;
}
else
printf("\n无人等待服务!\n");
break;
case 3:
printf("\n栈中剩余元素...\n");
while(!EmptyStack(&SK))
{
n=GetTop(&SK);
Pop(&SK);
printf("\n栈中剩余的元素为 %d!\n",n);
}
break;
case 0:
exit(1);
break;
}
}
}