<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>历史记录</title>
<style>
*{
margin: 0;
padding: 0;
}
body{
margin-left: 300px;
}
ul{
list-style: none;
}
ul li,div{
width: 250px;
padding: 10px 0;
margin-left: 10px;
border-bottom: 1px dashed #ccc;
height: 20px;
}
a{
float: right;
}
input{
padding: 5px;
margin: 10px;
}
</style>
</head>
<body>
<input id="text" type="search" placeholder="输入搜索关键字"/>
<input id="btn"type="button" value="搜索"/>
<div><a class="link" href="javascript:;">清空搜索记录</a></div>
<ul></ul>
<script src="js/jquery.min.js"></script>
<script>
//先获取存入数据的值
var historyList = localStorage.getItem("list") || "[]";
//转化成json数组格式
var listArr = JSON.parse(historyList);
function render() {
var html = "";
//遍历获取里面值创建添加
$(listArr).each(function (index,item) {
html += "<li><span>"+item+"</span><a href=\"javascript:;\" data-index='"+index+"'>删除</a></li>" //把值表示出来
})
html = html || "<li>没有搜索记录</li>"//判断之前有没有存入这个值
$("ul").html(html)//放入ul中
}
render();
$("#btn").click(function () {
var val = $('#text').val();
if(!val) return;
console.log(listArr.length);
if(listArr.length > 9){
listArr.pop();
}
if(listArr.indexOf(val,0) != -1)return;
listArr.unshift(val);
localStorage.setItem("list",JSON.stringify(listArr));
$("#text").val("");
render();
})
//点击操作 记得使用on事件 因为要让新添加的a也有点击事件
$("ul").on("click","a",function () {
var index = $(this).data('index');
listArr.splice(index,1);
localStorage.setItem("list",JSON.stringify(listArr));
render();
})
//全部删除
$(".link").click(function () {
listArr = [];
localStorage.removeItem("list");
render();
})
</script>
</body>
</html>
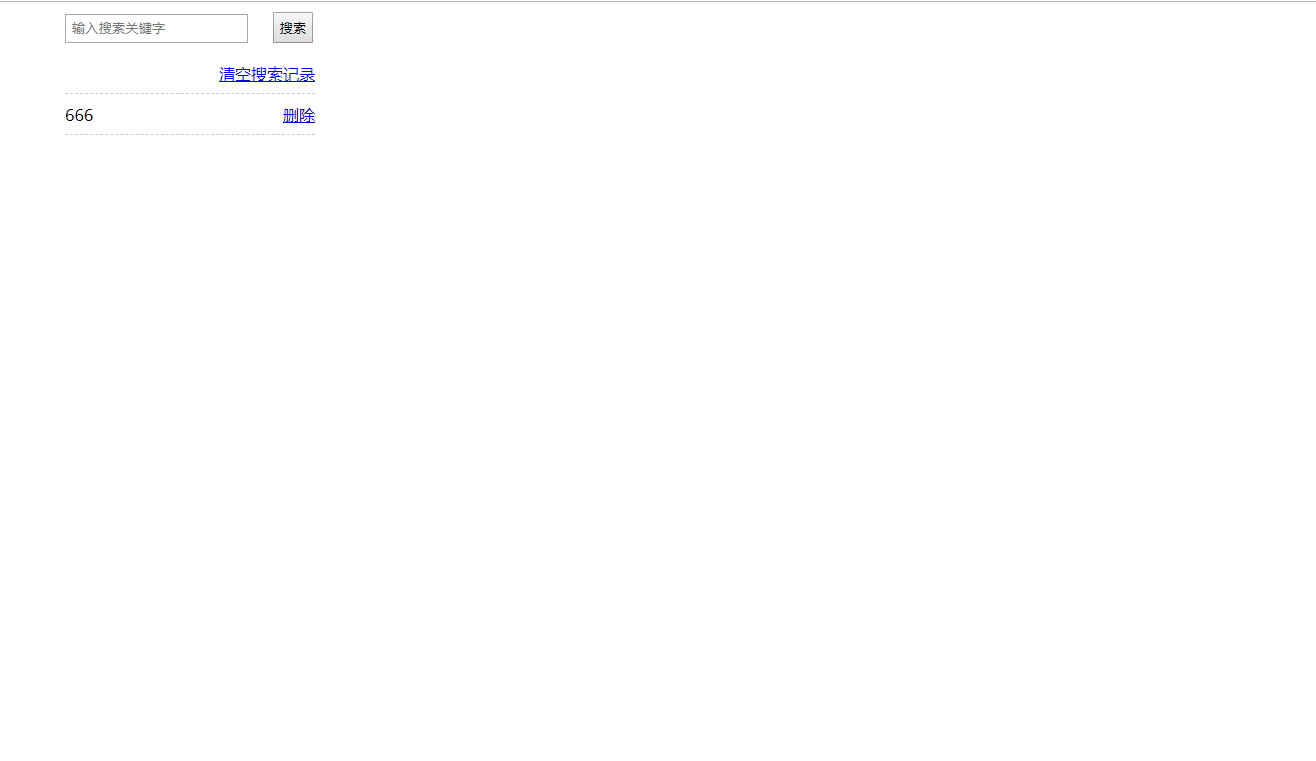
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>历史记录</title>
<style>
*{
margin: 0;
padding: 0;
}
body{
margin-left: 300px;
}
ul{
list-style: none;
}
ul li,div{
width: 250px;
padding: 10px 0;
margin-left: 10px;
border-bottom: 1px dashed #ccc;
height: 20px;
}
a{
float: right;
}
input{
padding: 5px;
margin: 10px;
}
</style>
</head>
<body>
<input id="text" type="search" placeholder="输入搜索关键字"/>
<input id="btn"type="button" value="搜索"/>
<div><a class="link" href="javascript:;">清空搜索记录</a></div>
<ul></ul>
<script src="js/jquery.min.js"></script>
<script>
//先获取存入数据的值
var historyList = localStorage.getItem("list") || "[]";
//转化成json数组格式
var listArr = JSON.parse(historyList);
function render() {
var html = "";
//遍历获取里面值创建添加
$(listArr).each(function (index,item) {
html += "<li><span>"+item+"</span><a href=\"javascript:;\" data-index='"+index+"'>删除</a></li>" //把值表示出来
})
html = html || "<li>没有搜索记录</li>"//判断之前有没有存入这个值
$("ul").html(html)//放入ul中
}
render();
$("#btn").click(function () {
var val = $('#text').val();
if(!val) return;
console.log(listArr.length);
if(listArr.length > 9){
listArr.pop();
}
if(listArr.indexOf(val,0) != -1)return;
listArr.unshift(val);
localStorage.setItem("list",JSON.stringify(listArr));
$("#text").val("");
render();
})
//点击操作 记得使用on事件 因为要让新添加的a也有点击事件
$("ul").on("click","a",function () {
var index = $(this).data('index');
listArr.splice(index,1);
localStorage.setItem("list",JSON.stringify(listArr));
render();
})
//全部删除
$(".link").click(function () {
listArr = [];
localStorage.removeItem("list");
render();
})
</script>
</body>
</html>