redis是一个nosql(非关系型数据库) , 可以用作数据库,缓存,消息中间件
连接redis需要注意的几点:
在redis.conf里设置 :
1.把daemonize 设置为yes, 这个属性不影响连接, 这只是把redis启动设置为后台启动
2.找到pretected-mode把他放开,并且设置为no ; 这是把安全模式关掉
3.把bind 127.0.0.1 : : 1这行注释掉 ,这样才能用别的ip地址还是什么去了
4. redis 版本在五点几之后,就需要给redis设置密码了 : requirepass xxx
创建spring-boot工程,在创建的时候选web里的web和nosql里的redis ,这里用的是spring data redis
创建好了工程后 , 再添加一pool2依赖
整体依赖如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.liy</groupId>
<artifactId>redis</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>redis</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
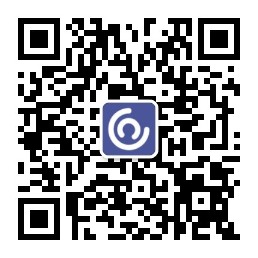
添加完依赖后就是配置文件了 application.properties
spring.redis.database=0
spring.redis.host=192.168.72.114
spring.redis.password=liy
spring.redis.port=6379
spring.redis.lettuce.pool.min-idle=5
spring.redis.lettuce.pool.max-idle=10
spring.redis.lettuce.pool.max-active=8
spring.redis.lettuce.pool.max-wait=1ms
spring.redis.lettuce.shutdown-timeout=100ms
默认的连接池是lettuce ,如果要用其他的连接池,需要在依赖里去排除lettuce的依赖 , 再导入你要用的连接池的依赖
整合redis非常的简单 , 主要是靠两个类 , 一个是RedisTemplate,一个是StringRedisTemplate
就写个service类测试下吧
@Service
public class HelloService {
@Autowired
RedisTemplate redisTemplate;
public void hello(){
redisTemplate.setKeySerializer(new StringRedisSerializer());
ValueOperations ops = redisTemplate.opsForValue();
ops.set("k1","v1");
Object k1 = ops.get("k1");
System.out.println(k1);
}
@Autowired
StringRedisTemplate stringRedisTemplate;
public void hello2(){
ValueOperations ops = stringRedisTemplate.opsForValue();
ops.set("k1","v1");
Object k1 = ops.get("k1");
System.out.println(k1);
}
}
用spring-boot的测试列测试
package com.liy;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.stereotype.Service;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
@SpringBootTest
public class RedisApplicationTests {
@Autowired
HelloService hs;
@Test
public void contextLoads() {
hs.hello();
// hs.hello2();
}
}
两个类的的区别是,传送的数据类型不同
RedisTemplate里传送的数据key和value都是object类型的
StringRedisTemplate里传送的数据都是String类型的
而如果用的是RedisTemplate,那么redis这个非关系型数据库就会以object的格式去序列化数据化
然后会如下图
而用如果用的是StringRedisTemplate传的数据,那么就会安装string类型来序列化 , string类型的数据序列话后 , 好像并没有变化
如果你只是value是object , 那么用RedisTemplate 的时候,可以用
redisTemplate.setKeySerializer(new StringRedisSerializer());
来设置key的序列化的方式 , 换成string类型的序列化
转载于:https://my.oschina.net/u/4116654/blog/3057911