re模块
用来从字符串(文本)中查找特定的东西
1.元字符:有特殊意义的字符
- ^ 从开头匹配
import re
a = re.findall('^abc', 'abcsds')
b = re.findall('^abc', 'aabcsds') # 不是以abc开头,所以返回空
print(a,b)
['abc'] []
- $ 从末尾开始匹配
a = re.findall('abc$', 'sdfabcsdsabc')
b = re.findall('abc$', 'aabcsdsbc') # 不是以abc结尾,所以返回空
print(a,b)
['abc'] []
- | 相当于或者or
a = re.findall('a|bc', 'sdfabbcsdsabc') # 将匹配到的对象用列表的形式返回
print(a)
['a', 'bc', 'a', 'bc']
- [] 找到[]内的元素
a = re.findall('[bac]', 'sdfabcsdsabc')
print(a)
['a', 'b', 'c', 'a', 'b', 'c']
- [^] 取反,匹配出除[^]里面的字符,^元字符如果写到字符集里就是反取
a = re.findall('[^bac]', 'sdfabcsdsabc')
print(a)
['s', 'd', 'f', 's', 'd', 's']
- () 找到匹配的结果后,只取()内的,分组匹配
a = re.findall('a(bc)s', 'sdfabcsdsabc')
print(a)
['bc']
- . 表示任意一个字符
a = re.findall('b.', 'sdb,sdb sdkjfbasd sdb') # 可表示任意字符,包括空格及其他字符
print(a)
['b,', 'b ', 'ba']
- {n} 将大括号前面最近的第一个字符匹配n次
a = re.findall('ab{3}','abbbbsfsabbs dfbbb')
print(a)
['abbb']
- * 前面字符匹配0到无穷个
a = re.findall('sa*','fsa dsaasdf')
print(a)
['sa', 'saa', 's']
- + 前面的字符匹配1到无穷个
a = re.findall('a+','fsa dsaasdf') # 至少要匹配到一个a
print(a)
['a', 'aa']
- ? 前面的字符匹配0个或1个
a = re.findall('sa?','fsa dsaasdf') # 匹配0或一个a
print(a)
['sa', 'sa', 's']
2.预定义字符:反斜杠后边跟普通字符实现特殊功能
- \d 匹配数字(0-9)
a = re.findall('\d', 'sda123jf 342 4sdf4')
print(a)
['1', '2', '3', '3', '4', '2', '4', '4']
- \D 匹配非数字的字符
a = re.findall('\D', 'sda123jf 342 4sdf4')
print(a)
['s', 'd', 'a', 'j', 'f', ' ', ' ', 's', 'd', 'f']
- \s 匹配空字符
a = re.findall('\s', 'sda123jf 342 4sd,f4')
print(a)
[' ', ' ']
- \S 匹配非空字符
a = re.findall('\S', 'sda123jf 342 4sd,f4')
print(a)
['s', 'd', 'a', '1', '2', '3', 'j', 'f', '3', '4', '2', '4', 's', 'd', ',', 'f', '4']
- \w 匹配字母、数字、下划线
a = re.findall('\w', 'sd_f 34?2 4sd,f4')
print(a)
['s', 'd', '_', 'f', '3', '4', '2', '4', 's', 'd', 'f', '4']
- \W 匹配非字母、非数字、非下划线的字符
a = re.findall('\W', 'sd_f 34?2 4sd,f4')
print(a)
[' ', '?', ' ', ',']
3.贪婪匹配:一直找,直到不满足
a = re.findall('a.*', 'asda123456asa')
print(a)
['asda123456asa']
4.非贪婪匹配,找到一个就停止,?相当于停止符
a = re.findall('a.*?', 'asda123456asa')
print(a)
['a', 'a', 'a', 'a']
5.常用的功能函数
- re.complie 相当于写一个通用的规则模板
phone_compile = re.compile('1\d{10}')
email_compile = re.compile('\w+@\w+.\w+')
test_s = '12345678900 [email protected] [email protected]'
res = phone_compile.findall(test_s)
print(res)
res = email_compile.findall(test_s)
print(res)
['12345678900']
['[email protected]', '[email protected]']
- re.match 从开头匹配,取一个匹配到的
a = re.match('\d','sdf123sdd456')
b = re.match('\d','123sdfa 212d')
print(a)
print(b)
None
<_sre.SRE_Match object; span=(0, 1), match='1'>
- re.search 搜索匹配到的第一个字符,并返回其索引
a = re.search('\d','sdfs1213hfjsf 2323')
print(a)
<_sre.SRE_Match object; span=(4, 5), match='1'>
match与search的区别:mathch从开头开始匹配找一个,search搜索所有找第一个
- re,split 根据正则匹配分割字符串,返回分割后的一个列表
s = 'asb sfsl sfjwo212 12312,dsfsf'
print(s.split(' '))
res = re.split('\d+',s)
print(res)
['asb', 'sfsl', 'sfjwo212', '12312,dsfsf']
['asb sfsl sfjwo', ' ', ',dsfsf']
- re,sub和re.subn 他们两都是替换内容,但是subn会计算替换了多少次,类似于字符串的replace内置方法
import re
s = 'asfhf12fdgds 743wiuw22'
print(re.sub('\d',',',s))
print(re.subn('\d',',',s)) # 除了会修改内容,还会返回修改了多少次
asfhf,,fdgds ,,,wiuw,,
('asfhf,,fdgds ,,,wiuw,,', 7)
typing模块
1.类型检查,防止运行时出现参数和返回值类型不符合。
2.作为开发文档附加说明,方便使用者调用时传入和返回参数类型。
3.该模块加入后并不会影响程序的运行,不会报正式的错误,只有提醒。
扫描二维码关注公众号,回复:
6458071 查看本文章
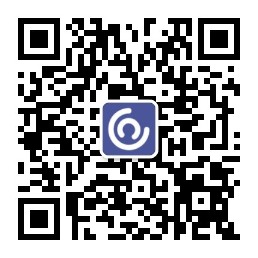
- 注意:typing模块只有在python3.5以上的版本中才可以使用,pycharm目前支持typing检查
from typing import List, Tuple, Dict
def add(a: int, string: str, f: float,
b: bool) -> Tuple[List, Tuple, Dict, bool]:
list1 = list(range(a))
tup = (string, string, string)
d = {"a": f}
bl = b
return list1, tup, d, bl
print(add(5, "hhhh", 2.3, False))
爬取音频
import re
import requests
response = requests.get('http://www.gov.cn/premier/index.htm')
data = response.text
res = re.findall('href="(/\w+/\w+_yp.htm)"', data) # ()只取括号内的
yp_res = 'http://www.gov.cn' + res[0]
yp_response = requests.get(yp_res)
yp_data = yp_response.text
res = re.findall('<a href="(.*?)"', yp_data)
count = 0
for url in res:
if url == 'javascript:;':
continue
mp3_url = 'http://www.gov.cn' + url
mp3_response = requests.get(mp3_url)
mp3_response.encoding = 'utf8' # 改变网址的utf8
mp3_data = mp3_response.text
# print(mp3_data)
res = re.findall('<title>(.*?)</title>|data-src="(.*?)"',mp3_data)
title = res[0][0]
mp3_url = res[1][1]
if res[1][1].startswith('/home'):
continue
res_response = requests.get(mp3_url)
mp3_data = res_response.content # MP3的二进制形式
with open(f'{title}.mp3','wb') as fw:
fw.write(mp3_data)
fw.flush()
count += 1
print(f'{count}')