一、数值的整数次方
题目描述
给定一个double类型的浮点数base和int类型的整数exponent。求base的exponent次方。
public class Solution {
public double Power(double base, int exponent) {
double result=1;
for(int i=1;i <= Math.abs(exponent);i++){
result*=base;
}
if(exponent<0){
result=1/result;
}
return result;
}
}
int类型的整数exponent :这个整数需要考虑为负的情况。否则for循环时会矛盾。
二、调整数组顺序使奇数位于偶数前面
题目描述
输入一个整数数组,实现一个函数来调整该数组中数字的顺序,使得所有的奇数位于数组的前半部分,所有的偶数位于数组的后半部分,并保证奇数和奇数,偶数和偶数之间的相对位置不变。
public class Solution {
public void reOrderArray(int [] array) {
int arraylength=array.length;
for(int i=1;i<arraylength;i++){
int temp=array[i];
int j=i-1;
if(array[i]%2!=0){
while(j>=0){
if(array[j]%2!=0){
break;
}
if(array[j]%2==0){
int t=array[j+1];
array[j+1]=array[j];
array[j]=t;
j--;
}
}
}
array[j+1]=temp;
}
}
}
从索引值为1的数开始,判断是否为奇数。
若是,则判断前一项;前一项为你奇数则停止,为偶数则与后一项换位置
三、链表中倒数第k个结点
题目描述
输入一个链表,输出该链表中倒数第k个结点。
思路:由于单项链表不能回头,我思考了两种方法
方法一:先遍历链表,算出链表节点数count,第二次直接遍历到第count-k个节点。但是要注意,可能链表结点数cout
小于k,此时要返回NULL。这一条件要先判断。
class Solution {
public:
ListNode* FindKthToTail(ListNode* pListHead, unsigned int k) {
// ListNode temp(0);
int count=0;//count用于统计结点数量
ListNode *temp = pListHead;
while (temp != NULL) //统计节点个数
{
++count;
temp = temp->next;
}
count -= k;
// pListHead = temp;
temp = pListHead;
//注意:要判断要找的节点是否在范围内,如果在范围内,返回节点;如果不在,返回NULL
if(count>=0)
{
while (count--) //从前往后再次搜索
{
temp = temp->next;
}
return temp;
}
else
return NULL;
}
};
方法二
可以用两个指针,一个指针遍历到第k个结点的时候,第二个指针再走到第一个节点,然后两个指针的距离始终保持k-1,这样,当第一个指针的next==NULL,也就是走到最后一个节点的时候,第二个指针对应的位置,就是倒数第k个结点。
这样的好处是能够节省一个循环,时间复杂度会相应降低。从Q(2N) 到Q(N)
注意,但是需要一个小循环让第一个指针先走到第k个指针。同时也存在结点总数小于k的问题,如果循环还没有进行到k次,而第一个指针的已经是NULL,即走到头了,那么,函数返回NULL。
扫描二维码关注公众号,回复:
6437243 查看本文章
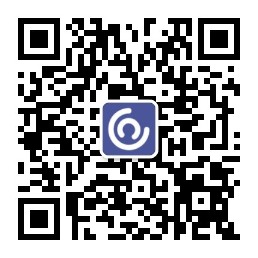
代码如下:可以直接拷贝到IDE上编译运行
//#include <stdio.h>
#include <stdlib.h>
#include <vector>
#include <stack>
#include <math.h>
#include <iostream>
using namespace std;
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :
val(x), next(NULL) {
}
};
class Solution {
public:
ListNode* FindKthToTail(ListNode* pListHead, unsigned int k) {
ListNode *first = pListHead; //第一个指针first
ListNode *second = pListHead; //第二个指针second
int i;
if(first == NULL)
return NULL;
for(i = 1; i <= k; i++){
if(first->next == NULL)
break;如果已经走到头了,就跳出,否则继续走
first = first->next;
}
while(first->next != NULL){//如果第一个指针没有走到头,两个指针想跟着一起走,保持k-1的距离
first = first->next;
second = second->next;
}
return second;
}
};
int main()
{
struct ListNode* p = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* r = (struct ListNode*)malloc(sizeof(struct ListNode));
p->val = 0;
p->next = NULL;
for(int i = 1000; i >= 1; i--){
struct ListNode* t = (struct ListNode*)malloc(sizeof(struct ListNode));//注意,这个空间申请一定要放到for循环内部。
t->val = i;//这种方法是插在头节点p前面,当然也可以插在后面,也可以插在链表的尾部,但是为了方便,需要用一个指针始终指向链表的尾节点
t->next = p->next;
p->next=t;
}
Solution s;
r = s.FindKthToTail(p,1000);
cout << r->val << endl;
system("pause");
return 0;
}