linux下大多数命令都需要根据情况加不同的参数,而我们自己编写的python脚本也不例外,通过参数来改变代码的功能,比起去修改代码方便多了。
1. 基础方式-sys.argv
import sys
所有的脚本后面所跟的参数都会保存在sys.argv中,然后自行判断参数个数,第几个参数是什么以及它的值。
2. 提高方式-optparse
#!/usr/bin/python #*-*coding=utf-8 from optparse import OptionParser def main(): usage = ''' test optparse ''' parser = OptionParser(usage) parser.add_option('-a', '--first',dest='arg1', help='this is first arg') parser.add_option('-b', '--second',dest='arg2', help='this is second arg') parser.add_option('-c', '--thrid',dest='arg3',help='this is third arg') #options是内部Values格式,访问数据跟dict一样 #args是解析完options后还剩下的参数,多余的参数 (options, args) = parser.parse_args() print(options) print(args) if __name__ == '__main__': main()
执行如下:
$ ./optparsetest.py --help Usage: test optparse Options: -h, --help show this help message and exit -a ARG1, --first=ARG1 this is first arg -b ARG2, --second=ARG2 this is second arg -c ARG3, --thrid=ARG3 this is third arg
$ ./optparsetest.py -a 1 -b 2 -c 3 4 {'arg1': '1', 'arg2': '2', 'arg3': '3'} ['4']
3. option详解
add_option的参数如下:
action : string type : string dest : string default : any nargs : int const : any choices : [string] callback : function callback_args : (any*) callback_kwargs : { string : any } help : string metavar : string
(1)dest 为保存参数的名称,通过options.destname来访问
(2)type 为参数的数据类型,根据数据类型可以自动做参数检查,并自动转化,方便使用
type支持的类型为 TYPES = ("string", "int", "long", "float", "complex", "choice")
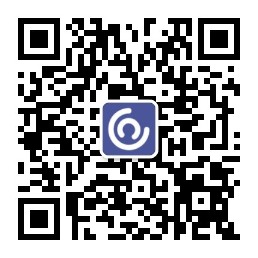
(3)const 为任意值,保存在参数类中(Options),使用const时action必须为store_const或append_const一起
parser.add_option('-c', '--thrid',dest='arg3',action='store_const', const='i am const', help='this is third arg')
$ ./optparsetest.py -a 1 -b 2 -c 3 {'arg1': '1', 'arg2': '2', 'arg3': 'i am const'} ['3']
store_const的优先级比命令行的高,此时-c 3中的3成了多余参数了,但是如果命令行不加-c,也不会有store_const这个动作了,arg3为None
(4)default 默认值,即使输入参数没有给出,也会有值,输入参数有值,则以输入值为准。
(5)nargs 为该参数接收的值个数,解析到该选项后会连续取两个值
(6)action 动作,分为
ACTIONS = ("store", "store_const","store_true","store_false","append","append_const","count","callback","help","version")
append为把解析好的dest的值加入defualt或者[]中,其中defualt为list
callback
def func(inst, opt, value, parser, *args, **kwargs): print(inst) print(opt) print(value) print(parser) print(args) print(kwargs) #这里进行想要的操作 parser.add_option('-f', '--function',type='int', action='callback', callback=func, callback_args=(1,2) , help='callback')