观察者模式
其定义了对象之间的一种一对多的依赖关系,当一个对象的状态发生改变时,所有依赖它的对象都会得到通知,并响应对应的行为。
需求描述:场景中有一个小球做自由落体运动,场景中存在三个Cube,这三个Cube会监视这个小球的运动状态,并保持自身的运动状态和小球的运动状态一致;同时,小球的坐标位置也会实时的显示到UI界面中。
演示例子:
定义被观察者的接口
/// <summary>
/// 角色接口
/// </summary>
/// <typeparam name="T">要发布的消息的数据类型</typeparam>
public interface ISubject<T> {
Action<T> PublicAction { get; set; }
}
定义观察者接口
/// <summary>
/// 观察者接口
/// </summary>
/// <typeparam name="T">接受消息的数据类型</typeparam>
public interface IObserver<T> {
ISubject<T> Publisher { get; set; }
void Subscriber (T data);
}
定义需要传递消息的数据结构
public struct DataType {
public Vector3 pos;
public string name;
}
定义并实现小球类(被观察者)(Ball)
小球做自由落体运动,在这个过程中,小球会不断的向外部发送自己当前的位置信息。
public class Ball : MonoBehaviour, ISubject<DataType> {
private DataType _dataType=new DataType{pos=Vector3.one,name="ball"};
public Action<DataType> PublicAction { get; set; }
private void PublishData () {
if (PublicAction != null) PublicAction (_dataType);
}
void Start () {
}
void Update () {
_dataType.pos=transform.position;
PublishData ();
}
}
定义具体观察者对象(Cube)
Cube会随时观察者小球的位置信息,并保持自己的位置和小球的位置同步。
public class Ball : MonoBehaviour, ISubject<DataType> {
private DataType _dataType=new DataType{pos=Vector3.one,name="ball"};
public Action<DataType> PublicAction { get; set; }
private void PublishData () {
if (PublicAction != null) PublicAction (_dataType);
}
void Start () {
}
void Update () {
_dataType.pos=transform.position;
PublishData ();
}
}
定义具体观察者对象(UIController)
UIController 接受来自Ball的数据,并将数据实时显示到界面上。
public class UIController : MonoBehaviour, IObserver<DataType> {
public Text _text;
public ISubject<DataType> Publisher { get; set; }
public void Subscriber (DataType data) {
_text.text = string.Format ("{0} : {1}", data.name, data.pos);
}
void Start () {
Publisher = FindObjectOfType<Ball> ();
Publisher.PublicAction += Subscriber;
}
}
演示Demo:
https://gitee.com/Sun_ME/ObserverPattern.git
更多内容,欢迎关注:
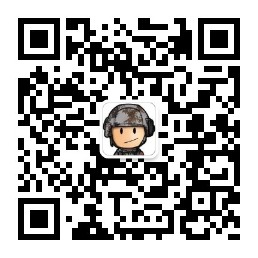