加载require
语法:
作用:
1、执行被加载模块中的代码
2、得到被加载模块中的exports导出接口对象
导出exports
Node中是模块作用域,默认文件中所有的成员只在当前文件模块有效。
对于希望可以被其它模块访问的成员,我们就需要把这些公开的成员都挂载到exports接口对象中就可以了。
1. 导出多个成员(必须在对象中)
2. 导出单个成员
错误写法1
错误写法2
如果一个模块需要直接导出某个成员,而非挂载的方式,那这个时候必须使用下面这种方式
扫描二维码关注公众号,回复:
6101349 查看本文章
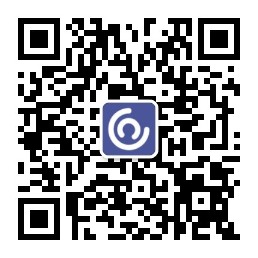
结果:
只有一个module.exports时,不管是在位置一还是位置二,都为 bar。
当有两个module.exports 时,比如一个在位置一,另一个在位置三,会导出位置三的对象(module.exports会被后者覆盖)。
上面的结果出现的原因:exports 和module.exports是有区别的。
在Node中,每个模块内部都有一个自己的module 对象,该 module 对象中,有一个成员叫exports也是一个对象,类似这样:
每次导出的对象是module.exports,也就是说如果你需要对外导出成员,只需要把导出的成员挂载到module.exports中,
node中有一句代码,来简化输入
exports相当于是 一个引用,指向module.exports对象,所以有
而当给 exports 赋值会断开和 module.exports 之间的引用。
同理,给 module.exports 重新赋值也会断开。
但是这里又重新建立两者的引用关系
最后,一定要记得return的是module.exports。
如果给exports赋值,断开了两个引用之间的联系,就不管用了。