发送get请求
private void requestDataByGet() {
try {
URL url = new URL("http://www.imooc.com/api/teacher?type=2&page=1");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(30*1000);
connection.setRequestMethod("GET");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Charset", "UTF-8");
connection.setRequestProperty("Accept-Charset", "UTF-8");
connection.connect(); // 发起连接
int responseCode = connection.getResponseCode();
String reponseMessage = connection.getResponseMessage();
if(responseCode == HttpURLConnection.HTTP_OK){
InputStream inputStream = connection.getInputStream();
mResult = streamToString(inputStream);
//跳回主线程
runOnUiThread(new Runnable() {
@Override
public void run() {
mResult = decode(mResult);
mTextView.setText(mResult);
}
});
} else {
// TODO: error fail
Log.e(TAG, "run: error code:" +responseCode +", message:" + reponseMessage);
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
发送post请求:
private void requestDataByPost() {
try {
// ?type=2&page=1
URL url = new URL("http://www.imooc.com/api/teacher");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(30*1000);
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Charset", "UTF-8");
connection.setRequestProperty("Accept-Charset", "UTF-8");
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setUseCaches(false);
connection.connect(); // 发起连接
String data = "username=" + getEncodeValue("imooc") + "&number=" + getEncodeValue("150088886666");
OutputStream outputStream = connection.getOutputStream();
outputStream.write(data.getBytes());
outputStream.flush();
outputStream.close();
int responseCode = connection.getResponseCode();
String reponseMessage = connection.getResponseMessage();
if(responseCode == HttpURLConnection.HTTP_OK){
InputStream inputStream = connection.getInputStream();
mResult = streamToString(inputStream);
runOnUiThread(new Runnable() {
@Override
public void run() {
mResult = decode(mResult);
mTextView.setText(mResult);
}
});
} else {
// TODO: error fail
Log.e(TAG, "run: error code:" +responseCode +", message:" + reponseMessage);
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
//对发送的信息封装
private String getEncodeValue(String imooc) {
String encode = null;
try {
encode = URLEncoder.encode(imooc, "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return encode;
}
将输入流解析成字符串
/**
* 将输入流转换成字符串
*
* @param is 从网络获取的输入流
* @return 字符串
*/
public String streamToString(InputStream is) {
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
baos.write(buffer, 0, len);
}
baos.close();
is.close();
byte[] byteArray = baos.toByteArray();
return new String(byteArray);
} catch (Exception e) {
Log.e(TAG, e.toString());
return null;
}
}
解析JSON数据:
JSONOBJECT指的是用大括号括起来的,JSONArray指的是用中括号括起来的【】.
映射json:
public class LessonResult {
private int mStatus;
private List<Lesson> mLessons;
public int getStatus() {
return mStatus;
}
public void setStatus(int status) {
mStatus = status;
}
public List<Lesson> getLessons() {
return mLessons;
}
public void setLessons(List<Lesson> lessons) {
mLessons = lessons;
}
@Override
public String toString() {
return "LessonResult{" +
"mStatus=" + mStatus +
", mLessons=" + mLessons +
'}';
}
public static class Lesson{
private int mID;
private int mLearnerNumber;
private String mName;
private String mSmallPictureUrl;
private String mBigPictureUrl;
private String mDescription;
public int getID() {
return mID;
}
public void setID(int ID) {
mID = ID;
}
public int getLearnerNumber() {
return mLearnerNumber;
}
public void setLearnerNumber(int learnerNumber) {
mLearnerNumber = learnerNumber;
}
public String getName() {
return mName;
}
public void setName(String name) {
mName = name;
}
public String getSmallPictureUrl() {
return mSmallPictureUrl;
}
public void setSmallPictureUrl(String smallPictureUrl) {
mSmallPictureUrl = smallPictureUrl;
}
public String getBigPictureUrl() {
return mBigPictureUrl;
}
public void setBigPictureUrl(String bigPictureUrl) {
mBigPictureUrl = bigPictureUrl;
}
public String getDescription() {
return mDescription;
}
public void setDescription(String description) {
mDescription = description;
}
@Override
public String toString() {
return "Lesson{" +
"mID=" + mID +
", mLearnerNumber=" + mLearnerNumber +
", mName='" + mName + '\'' +
", mSmallPictureUrl='" + mSmallPictureUrl + '\'' +
", mBigPictureUrl='" + mBigPictureUrl + '\'' +
", mDescription='" + mDescription + '\'' +
'}';
}
}
}
处理json数据:
private void handleJSONData(String result) {
try {
LessonResult lessonResult = new LessonResult();
JSONObject jsonObject = new JSONObject(result);
List<LessonResult.Lesson> lessonList = new ArrayList<>();
int status = jsonObject.getInt("status");
JSONArray lessons = jsonObject.getJSONArray("data");//读data数组
lessonResult.setStatus(status);
if(lessons != null && lessons.length() > 0){
for (int index = 0; index < lessons.length(); index++) {
JSONObject lesson = (JSONObject) lessons.get(index);//一个大括号item
int id = lesson.getInt("id");
int learner = lesson.getInt("learner");
String name = lesson.getString("name");
String smallPic = lesson.getString("picSmall");
String bigPic = lesson.getString("picBig");
String description = lesson.getString("description");
//赋值
LessonResult.Lesson lessonItem = new LessonResult.Lesson();
lessonItem.setID(id);
lessonItem.setName(name);
lessonItem.setSmallPictureUrl(smallPic);
lessonItem.setBigPictureUrl(bigPic);
lessonItem.setDescription(description);
lessonItem.setLearnerNumber(learner);
lessonList.add(lessonItem);
}
lessonResult.setLessons(lessonList);
}
mTextView.setText(lessonResult.toString());
} catch (JSONException e) {
e.printStackTrace();
}
}
现在有gson等第三方库来解析json。
——来自慕课网
扫描二维码关注公众号,回复:
6085928 查看本文章
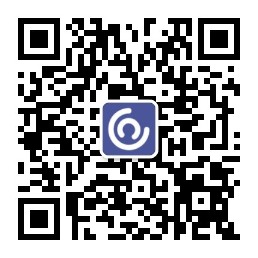