一、父窗口(容器窗口)
1.概念:创建控件时可以指定停靠在父窗口上,如果没有指定,则飘在外面形成独立的窗体,父窗口本质也是图形控件,常用于表示父窗口类主要包括如下三个:
QWidget
QDialog(对话框)
QMainWindow(主窗口)
注:QWidget和它的所有子类都可以作为父窗口,但是常用的父窗口类只有上面三个
参考代码:
#include <QApplication>
#include <QPushButton>
#include <QDialog>
int main(int argc,char** argv)
{
QApplication app(argc,argv);
//创建父窗口对象(本质也是图形控件)
QDialog parent;
parent.resize(400,400);
parent.move(100,100);
//创建按钮,并停靠在父窗口上面
QPushButton button("Button",&parent);
button.resize(120,120);
button.move(50,50);
//显示父窗口,上面停靠的控件也会一起显示
parent.show();
return app.exec();
}
二、信号和槽机制
1.概念:信号和槽是QT自行定义的一种通信机制,实现对象之间的数据交互
2.定义
class XX:public QObject{
Q_OBJECT //moc(元对象编译器)
signals:
void sig_func(); //信号函数
public slots:
void slot_func(){} //槽函数
};
注:信号函数只需声明,不能定义
注:槽函数可以被连接到信号上,通过信号触发槽函数执行,也可以把槽函数当做一个普通的成员函数去调用
注:能够定义信号或槽的类,必须是QObject的子类
扫描二维码关注公众号,回复:
6042823 查看本文章
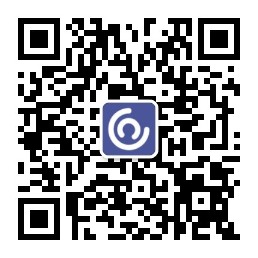
3.信号和槽的连接
QObject::connect{
const QObject* sender;
const char* signal;
const QObject* receiver;
const char* method;
}
@sender:信号的发送对象
@signal:信号函数
@receiver:信号的接收对象
@method:槽函数
//将信号函数转换为const char*
//SIGNAL(信号函数(参数表));
//eg:SIGNAL(clicked(void));
//将槽函数转换为const char*
//SLOT(槽函数(参数表));
//eg:SLOT(close(void));
参考代码:
#include <QApplication>
#include <QPushButton>
#include <QLabel>
#include <QDialog>
int main(int argc,char** argv)
{
QApplication app(argc,argv);
QDialog parent;
parent.resize(480,320);
QPushButton button("关闭标签",&parent);
QLabel label("请关闭我!!",&parent);
button.move(30,50);
label.move(180,50);
button.resize(100,100);
QPushButton button2("退出",&parent);
button2.move(30,200);
button2.resize(100,100);
parent.show();
//点击按钮,实现关闭标签功能
QObject::connect(
&button,SIGNAL(clicked(void)),
&label,SLOT(close(void)));
//练习:添加一个按钮,显示"退出",实现点
//击该按钮,退出应用程序.
QObject::connect(
&button2,SIGNAL(clicked(void)),
//&app,SLOT(closeAllWindows(void)));
&app,SLOT(quit(void)));
//&parent,SLOT(close(void)));
return app.exec();
}
4)信号和槽连接的语法要求
1)信号和槽参数要一致
QObject::connect(A,SIGNAL(sigfunc(int)),
B,SLOT(slotfunc(int)));//ok
QObject::connect(A,SIGNAL(sigfunc(void)),
B,SLOT(slotfunc(int)));//error
2)可以带有缺省参数
QObject::connect(A,SIGNAL(sigfunc(void)),
B,SLOT(slotfunc(int=0)));
3)信号函数参数可以多于槽函数,多余参数将被忽略
QObject::connect(A,SIGNAL(sigfunc(int)),
B,SLOT(slotfunc(void)));
4)一个信号可以连接多个槽函数(一对多)
QObject::connect(A,SIGNAL(sigfunc(void)),
B1,SLOT(slotfunc1(void)));
5)多个信号连接到同一个槽函数(多对一)
QObject::connect(A1,SIGNAL(sigfunc1(void)),
B,SLOT(slotfunc(void)));
QObject::connect(A2,SIGNAL(sigfunc2(void)),
B,SLOT(slotfunc(void)));
注:无论A1还是A2发送信号时,B的槽函数都会被执行
6)两个信号可以直接连接//了解
QObject::connect(A1,SIGNAL(sigfunc1(void)),
A2,SIGNAL(sigfunc2(void)));
案例:事件同步,滑块控件和选值框控件同步运行
参考代码:
#include <QApplication>
#include <QSlider>
#include <QSpinBox>
#include <QDialog>
int main(int argc,char** argv)
{
QApplication app(argc,argv);
QDialog parent;
parent.resize(320,240);
//滑块
QSlider slider(Qt::Horizontal,&parent);
slider.move(30,50);
slider.setRange(0,100);
//选值框
QSpinBox spin(&parent);
spin.move(130,50);
spin.setRange(0,100);
//显示
parent.show();
//滑块滑动时,选值框数值随之变化
QObject::connect(
&slider,SIGNAL(valueChanged(int)),
&spin,SLOT(setValue(int)));
//选值框数值改变,滑块随之滑动
QObject::connect(
&spin,SIGNAL(valueChanged(int)),
&slider,SLOT(setValue(int)));
return app.exec();
}