using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace CoordinateSystem
{
public partial class Form1 : Form
{
int orgX = 20;
int orgY = 20;//原点距离边框左,下距离
int move = 20;//格子间隔
int timerI = 0;
public Form1()
{
InitializeComponent();
timer1.Interval = 1000;
timer1.Enabled = true;
}
private void panel1_Paint(object sender, PaintEventArgs e)
{
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace CoordinateSystem
{
public partial class Form1 : Form
{
int orgX = 20;
int orgY = 20;//原点距离边框左,下距离
int move = 20;//格子间隔
int timerI = 0;
public Form1()
{
InitializeComponent();
timer1.Interval = 1000;
timer1.Enabled = true;
}
private void panel1_Paint(object sender, PaintEventArgs e)
{
Draw draw = new Draw();
draw.DrawX(move,panel1,orgX,orgY);
draw.DrawY(move, panel1, orgX, orgY);
}
draw.DrawX(move,panel1,orgX,orgY);
draw.DrawY(move, panel1, orgX, orgY);
}
private void button1_Click(object sender, EventArgs e)
{
panel1.Refresh();
Graphics g = panel1.CreateGraphics();
Pen pen = new Pen(Color.Red, 1);
double x1 = panel1.Width/2;
double y1 = 0;
for (double x = 1; x < panel1.Width; x++)//这一块就是画正弦波的位置 panel1.width/2是偏移波到坐标系0点起始的作用。
{
//SIN最大值为1,而我图上用了100像素,所以有
//100/1=100 故而y要乘100;
// x/180*Math.PI 这个表达式把角度换成弧度值
double y = Math.Sin(x / 180 * Math.PI) * 60;
//SIN值一个循环为360度,而我图上用了200像素表示,所以有:
//360/200=1.8 故而x值要除1.8
{
panel1.Refresh();
Graphics g = panel1.CreateGraphics();
Pen pen = new Pen(Color.Red, 1);
double x1 = panel1.Width/2;
double y1 = 0;
for (double x = 1; x < panel1.Width; x++)//这一块就是画正弦波的位置 panel1.width/2是偏移波到坐标系0点起始的作用。
{
//SIN最大值为1,而我图上用了100像素,所以有
//100/1=100 故而y要乘100;
// x/180*Math.PI 这个表达式把角度换成弧度值
double y = Math.Sin(x / 180 * Math.PI) * 60;
//SIN值一个循环为360度,而我图上用了200像素表示,所以有:
//360/200=1.8 故而x值要除1.8
//Matrix myMatrix = new Matrix(1, 0, 0, -1, 0, 0);
//g.Transform = myMatrix;
//g.TranslateTransform(0, (float)panel1.Height / 2);//画图前发这个,移动到X,Y位置
g.DrawLine(pen, (float)x1, (float)y1 + 60, (float)(x / 1.8) + panel1.Width / 2, (float)y + 60);
x1 = x / 1.8 + panel1.Width / 2;
y1 = y;
}
//pictureBoxFrequencyDomain.Image = bitmap;
//g.Transform = myMatrix;
//g.TranslateTransform(0, (float)panel1.Height / 2);//画图前发这个,移动到X,Y位置
g.DrawLine(pen, (float)x1, (float)y1 + 60, (float)(x / 1.8) + panel1.Width / 2, (float)y + 60);
x1 = x / 1.8 + panel1.Width / 2;
y1 = y;
}
//pictureBoxFrequencyDomain.Image = bitmap;
// Graphics g = panel1.CreateGraphics();
// g.DrawEllipse(new Pen(Color.Red, 2), panel1.Width / 2 - 50, panel1.Height / 2 - 50, 100, 100);//红色不填充圆
// g.FillEllipse(new SolidBrush(Color.Red), panel1.Width / 2 - 50, panel1.Height / 2 - 50, 100, 100);//红色填充圆
}
}
public class Draw
{
//X轴方法
public void DrawX(int move,Panel panel,int orgX,int orgY)//画X轴上分格
{
int a = (panel.Width/2) / move;//X轴有多少格
Graphics g = panel.CreateGraphics();
g.DrawLine(new Pen(Color.Black, 3), new Point(panel.Width/2, panel.Height),//画X轴长线
new Point(panel.Width/2, 0));
// g.DrawEllipse(new Pen(Color.Red, 2), panel1.Width / 2 - 50, panel1.Height / 2 - 50, 100, 100);//红色不填充圆
// g.FillEllipse(new SolidBrush(Color.Red), panel1.Width / 2 - 50, panel1.Height / 2 - 50, 100, 100);//红色填充圆
}
}
public class Draw
{
//X轴方法
public void DrawX(int move,Panel panel,int orgX,int orgY)//画X轴上分格
{
int a = (panel.Width/2) / move;//X轴有多少格
Graphics g = panel.CreateGraphics();
g.DrawLine(new Pen(Color.Black, 3), new Point(panel.Width/2, panel.Height),//画X轴长线
new Point(panel.Width/2, 0));
for (int i = 0; i < a; i++)
{
g.DrawLine(new Pen(Color.Black, 3), new Point(panel.Width/2+ move * i, panel.Height/2),
new Point(panel.Width / 2 + move * i, panel.Height / 2 - 4));//划分+格子
g.DrawLine(new Pen(Color.Black, 3),new Point(panel.Width / 2 - move * i, panel.Height / 2),
new Point(panel.Width / 2 - move * i, panel.Height / 2 - 4));//划分-格子
g.DrawString(i.ToString(), new Font("宋体", 8f), Brushes.Black, panel.Width / 2 + move * i,
panel.Height / 2 + 4 );//写X轴+的数字
g.DrawLine(new Pen(Color.Black, 3), new Point(panel.Width/2+ move * i, panel.Height/2),
new Point(panel.Width / 2 + move * i, panel.Height / 2 - 4));//划分+格子
g.DrawLine(new Pen(Color.Black, 3),new Point(panel.Width / 2 - move * i, panel.Height / 2),
new Point(panel.Width / 2 - move * i, panel.Height / 2 - 4));//划分-格子
g.DrawString(i.ToString(), new Font("宋体", 8f), Brushes.Black, panel.Width / 2 + move * i,
panel.Height / 2 + 4 );//写X轴+的数字
if (i!=0)
{
g.DrawString("-" + i.ToString(), new Font("宋体", 8f), Brushes.Black, panel.Width / 2 - move * i,
panel.Height / 2 + 4);//写X轴-的数字
}
}
g.DrawString("X轴", new Font("宋体", 10f), Brushes.Black, panel.Width-30, panel.Height/2+20);
{
g.DrawString("-" + i.ToString(), new Font("宋体", 8f), Brushes.Black, panel.Width / 2 - move * i,
panel.Height / 2 + 4);//写X轴-的数字
}
}
g.DrawString("X轴", new Font("宋体", 10f), Brushes.Black, panel.Width-30, panel.Height/2+20);
}
public void DrawY(int move,Panel pan,int orgX,int orgY)
{
Graphics g = pan.CreateGraphics();
g.DrawLine(new Pen(Color.Black, 3), new Point(0, pan.Height/2),
new Point(pan.Width, pan.Height / 2));//画Y轴长线
{
Graphics g = pan.CreateGraphics();
g.DrawLine(new Pen(Color.Black, 3), new Point(0, pan.Height/2),
new Point(pan.Width, pan.Height / 2));//画Y轴长线
int a = (pan.Height/2) / move;
for (int i = 0; i < a; i++)
{
g.DrawLine(new Pen(Color.Black, 3), new Point(pan.Width/2, pan.Height/2 - move * i),
new Point(pan.Width / 2 + 4, pan.Height / 2 - move * i));
g.DrawLine(new Pen(Color.Black, 3), new Point(pan.Width / 2, pan.Height / 2 + move * i),
new Point(pan.Width / 2 + 4, pan.Height / 2 + move * i));
if (i!=0)
{
g.DrawString(i.ToString(), new Font("宋体", 8f), Brushes.Black, pan.Width / 2 - 10,
pan.Height / 2 - move * i);
g.DrawString("-"+i.ToString(), new Font("宋体", 8f), Brushes.Black, pan.Width / 2 - 15,
pan.Height / 2 + move * i);
}
}
g.DrawString("Y轴", new Font("宋体", 10f),Brushes.Black, orgX, orgY - 20);
}
}
}
for (int i = 0; i < a; i++)
{
g.DrawLine(new Pen(Color.Black, 3), new Point(pan.Width/2, pan.Height/2 - move * i),
new Point(pan.Width / 2 + 4, pan.Height / 2 - move * i));
g.DrawLine(new Pen(Color.Black, 3), new Point(pan.Width / 2, pan.Height / 2 + move * i),
new Point(pan.Width / 2 + 4, pan.Height / 2 + move * i));
if (i!=0)
{
g.DrawString(i.ToString(), new Font("宋体", 8f), Brushes.Black, pan.Width / 2 - 10,
pan.Height / 2 - move * i);
g.DrawString("-"+i.ToString(), new Font("宋体", 8f), Brushes.Black, pan.Width / 2 - 15,
pan.Height / 2 + move * i);
}
}
g.DrawString("Y轴", new Font("宋体", 10f),Brushes.Black, orgX, orgY - 20);
}
}
}
以下是运行后的界面
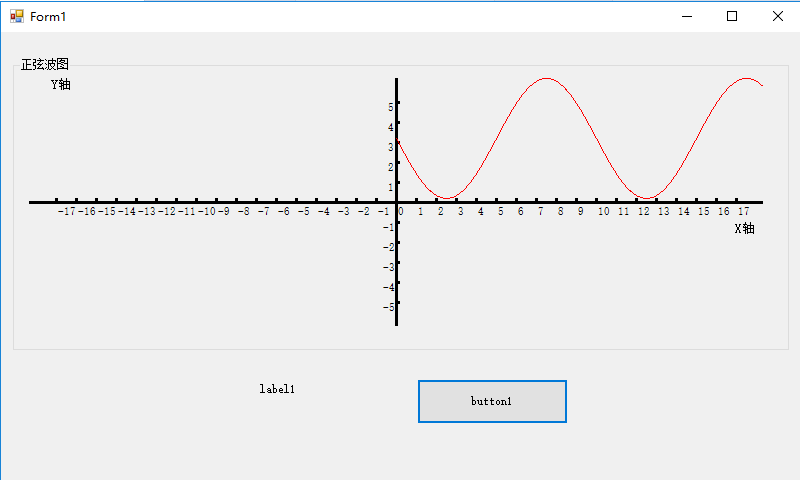