一、SystemServer
Zygote如何启动SystemServer就不分析了,主要分析下java层:
先看下主函数
- public static void main(String[] args) {
- new SystemServer().run();
- }
下面我们看run函数里面的java service的启动:
- mSystemServiceManager = new SystemServiceManager(mSystemContext);
- LocalServices.addService(SystemServiceManager.class, mSystemServiceManager);
- // Start services.
- try {
- startBootstrapServices();
- startCoreServices();
- startOtherServices();
- } catch (Throwable ex) {
- Slog.e("System", "******************************************");
- Slog.e("System", "************ Failure starting system services", ex);
- throw ex;
- }
SystemServiceManager这个类只是管理各个service,用SystemServiceManager启动service的时候,会把service加入自己的链表。并且调用service的onStart函数。
- public <T extends SystemService> T startService(Class<T> serviceClass) {
- final String name = serviceClass.getName();
- Slog.i(TAG, "Starting " + name);
- // Create the service.
- if (!SystemService.class.isAssignableFrom(serviceClass)) {
- throw new RuntimeException("Failed to create " + name
- + ": service must extend " + SystemService.class.getName());
- }
- final T service;
- try {
- Constructor<T> constructor = serviceClass.getConstructor(Context.class);
- service = constructor.newInstance(mContext);
- } catch (InstantiationException ex) {
- throw new RuntimeException("Failed to create service " + name
- + ": service could not be instantiated", ex);
- } catch (IllegalAccessException ex) {
- throw new RuntimeException("Failed to create service " + name
- + ": service must have a public constructor with a Context argument", ex);
- } catch (NoSuchMethodException ex) {
- throw new RuntimeException("Failed to create service " + name
- + ": service must have a public constructor with a Context argument", ex);
- } catch (InvocationTargetException ex) {
- throw new RuntimeException("Failed to create service " + name
- + ": service constructor threw an exception", ex);
- }
- // Register it.
- mServices.add(service);//加入链表
- // Start it.
- try {
- service.onStart();//调用service的onStart函数
- } catch (RuntimeException ex) {
- throw new RuntimeException("Failed to start service " + name
- + ": onStart threw an exception", ex);
- }
- return service;
- }
SystemServer每执行到一个阶段都会调用SystemServiceManager的startBootPhase函数
- mActivityManagerService = mSystemServiceManager.startService(
- ActivityManagerService.Lifecycle.class).getService();
- mActivityManagerService.setSystemServiceManager(mSystemServiceManager);
- mActivityManagerService.setInstaller(installer);
- // Power manager needs to be started early because other services need it.
- // Native daemons may be watching for it to be registered so it must be ready
- // to handle incoming binder calls immediately (including being able to verify
- // the permissions for those calls).
- mPowerManagerService = mSystemServiceManager.startService(PowerManagerService.class);
- // Now that the power manager has been started, let the activity manager
- // initialize power management features.
- mActivityManagerService.initPowerManagement();
- // Display manager is needed to provide display metrics before package manager
- // starts up.
- mDisplayManagerService = mSystemServiceManager.startService(DisplayManagerService.class);
- // We need the default display before we can initialize the package manager.
- mSystemServiceManager.startBootPhase(SystemService.PHASE_WAIT_FOR_DEFAULT_DISPLAY);
我们下面看下SystemServiceManager的startBootPhase函数,它会把mServices中的service取出来,调用onBootPhase函数
- public void startBootPhase(final int phase) {
- if (phase <= mCurrentPhase) {
- throw new IllegalArgumentException("Next phase must be larger than previous");
- }
- mCurrentPhase = phase;
- Slog.i(TAG, "Starting phase " + mCurrentPhase);
- final int serviceLen = mServices.size();
- for (int i = 0; i < serviceLen; i++) {
- final SystemService service = mServices.get(i);
- try {
- service.onBootPhase(mCurrentPhase);
- } catch (Exception ex) {
- throw new RuntimeException("Failed to boot service "
- + service.getClass().getName()
- + ": onBootPhase threw an exception during phase "
- + mCurrentPhase, ex);
- }
- }
- }
二、SystemService
我们再来SystemService这个类,这个类一般是一些系统service来继承这个类
先看看PowerManagerService
- public final class PowerManagerService extends SystemService
- implements Watchdog.Monitor {
其中实现了onStart和onBootPhase函数
扫描二维码关注公众号,回复:
592827 查看本文章
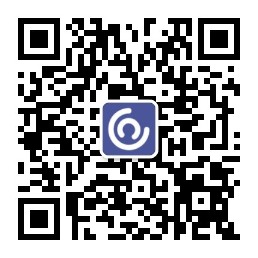
- @Override
- public void onStart() {
- publishBinderService(Context.POWER_SERVICE, new BinderService());
- publishLocalService(PowerManagerInternal.class, new LocalService());
- Watchdog.getInstance().addMonitor(this);
- Watchdog.getInstance().addThread(mHandler);
- }
- @Override
- public void onBootPhase(int phase) {
- synchronized (mLock) {
- if (phase == PHASE_BOOT_COMPLETED) {
- final long now = SystemClock.uptimeMillis();
- mBootCompleted = true;
- mDirty |= DIRTY_BOOT_COMPLETED;
- userActivityNoUpdateLocked(
- now, PowerManager.USER_ACTIVITY_EVENT_OTHER, 0, Process.SYSTEM_UID);
- updatePowerStateLocked();
- }
- }
- }
其中publishBinderService函数是SystemService中的函数,最后是调用了ServiceManager.addService。
- protected final void publishBinderService(String name, IBinder service) {
- publishBinderService(name, service, false);
- }
- /**
- * Publish the service so it is accessible to other services and apps.
- */
- protected final void publishBinderService(String name, IBinder service,
- boolean allowIsolated) {
- ServiceManager.addService(name, service, allowIsolated);
- }
三、ServiceManager
而ServiceManager 这个类,是java层用来和serviceManager进程通信的。
- public final class ServiceManager {
- private static final String TAG = "ServiceManager";
- private static IServiceManager sServiceManager;
- private static HashMap<String, IBinder> sCache = new HashMap<String, IBinder>();
- private static IServiceManager getIServiceManager() {
- if (sServiceManager != null) {
- return sServiceManager;
- }
- // Find the service manager
- sServiceManager = ServiceManagerNative.asInterface(BinderInternal.getContextObject());
- return sServiceManager;
- }
- /**
- * Returns a reference to a service with the given name.
- *
- * @param name the name of the service to get
- * @return a reference to the service, or <code>null</code> if the service doesn't exist
- */
- public static IBinder getService(String name) {
- try {
- IBinder service = sCache.get(name);
- if (service != null) {
- return service;
- } else {
- return getIServiceManager().getService(name);
- }
- } catch (RemoteException e) {
- Log.e(TAG, "error in getService", e);
- }
- return null;
- }
- /**
- * Place a new @a service called @a name into the service
- * manager.
- *
- * @param name the name of the new service
- * @param service the service object
- */
- public static void addService(String name, IBinder service) {
- try {
- getIServiceManager().addService(name, service, false);
- } catch (RemoteException e) {
- Log.e(TAG, "error in addService", e);
- }
- }
四、系统的各个Manager
再来看看ContextImpl里面对各个Manager的注册,下面是PMS的注册
- registerService(POWER_SERVICE, new ServiceFetcher() {
- public Object createService(ContextImpl ctx) {
- IBinder b = ServiceManager.getService(POWER_SERVICE);
- IPowerManager service = IPowerManager.Stub.asInterface(b);
- if (service == null) {
- Log.wtf(TAG, "Failed to get power manager service.");
- }
- return new PowerManager(ctx.getOuterContext(),
- service, ctx.mMainThread.getHandler());
- }});
- @Override
- public Object getSystemService(String name) {
- ServiceFetcher fetcher = SYSTEM_SERVICE_MAP.get(name);
- return fetcher == null ? null : fetcher.getService(this);
- }
- /*package*/ static class ServiceFetcher {
- int mContextCacheIndex = -1;
- /**
- * Main entrypoint; only override if you don't need caching.
- */
- public Object getService(ContextImpl ctx) {
- ArrayList<Object> cache = ctx.mServiceCache;
- Object service;
- synchronized (cache) {
- if (cache.size() == 0) {
- // Initialize the cache vector on first access.
- // At this point sNextPerContextServiceCacheIndex
- // is the number of potential services that are
- // cached per-Context.
- for (int i = 0; i < sNextPerContextServiceCacheIndex; i++) {
- cache.add(null);
- }
- } else {
- service = cache.get(mContextCacheIndex);
- if (service != null) {
- return service;
- }
- }
- service = createService(ctx);
- cache.set(mContextCacheIndex, service);
- return service;
- }
- }
- /**
- * Override this to create a new per-Context instance of the
- * service. getService() will handle locking and caching.
- */
- public Object createService(ContextImpl ctx) {
- throw new RuntimeException("Not implemented");
- }
- }