1. 递归编程
题目不明,没有编程
2. 整形转化为字符串
- 内置函数(string to_string(int val)) ,这是string内的函数
- 字符串流
string IntToStr(int n)
{
ostringstream stream;
stream << n;
return stream.str();
}
template <class out_type,class in_type)
out_type covert(const in_type &t)
{
stringstream stream;
stream << t;
out_type result;
stream >> result;
return result;
}
应用如下:
double d;
string s = "12.21";
d = cover<double>(s);
string salary;
salary = cover<string>(9000.0)
string变成int 使用int atoi(const char*); string函数有,cstring函数也有
c风格字符串转string,应用string构造函数即可
string转c风格字符串,应用string.c_str(),内置函数,返回的是const char*;
常规方法:
void IntToStr(int a, char s[])
{
int count = 0;
while(a){
s[count] = a%10 + '0';
a = a/10;
count ++;
}
s[count] = '\0';
int left = 0;
int right = count - 1;
char c;
while(left < right)
{
c = s[left];
s[left] = s[right];
s[right] = c;
left ++;
right --;
}
}
扩展:将数字反转
int reverseNumber(int number)
{
int reverse = 0;
while(number > 0)
{
reverse *= 10;
reverse += number%10;
number /= 10;
}
return reverse;
}
3. 编写程序,使数组的前m项和后n项交换位置
#include <iostream>
using namespace std;
void exchange(int [],int,int);
void swap(int [],int,int);
int main()
{
int a[] = {1,2,3,4,5,6,7,8,9,10};
for(int i = 0; i < 10; i++)
cout << a[i] << " ";
swap(a,6,4);
cout << endl;
for(int i = 0; i < 10; i++)
cout << a[i] << " ";
return 0;
}
void exchange(int a[],int left,int right)
{
int temp;
while(left < right)
{
temp = a[left];
a[left] = a[right];
a[right] = temp;
left ++;
right --;
}
}
void swap(int a[],int m, int n)
{
exchange(a, 0, m-1);
exchange(a, m, m + n-1);
exchange(a, 0, m + n-1);
}
void swap1(int a[],int m, int n)
{
int x = 0,y =m,z,t;
while(x<y)
{
t = y;
for(;x<t&&y < m+n;x++,y++)
{
z = a[x];
a[x] = a[y];
a[y] = z
}
if(y == m+n) y = t;
}
}
4. (1)日期类Dte
成员数据有年月日,成员函数有无参构造函数,设置年月日的函数setDate.
还有display打印函数
(2)第二个类是员工类Employee,
成员有工号、姓名、身份证号、出生日期、受聘日期、聘用年限、月薪
成员函数要有构造函数、改变出生日期函数、改变聘用年限函数、改变月薪函数、续聘函数(要求当续聘后的年龄大于60时给提示不能续聘)
还有展示函数display,需要有工号、姓名、身份证号、出生日期、聘用【到期】时间、聘用年限、【年】薪
注意第二个类会有Date类或其指针作为成员
Date.h
扫描二维码关注公众号,回复:
5806146 查看本文章
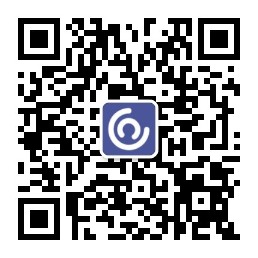
#ifndef DATE_H
#define DATE_H
class Date
{
public:
Date(Date &);
Date(int y = 1900,int m = 1, int n = 1);
void setDate(int ,int ,int );
void setYear(int);
void setMonth(int);
void setDay(int);
int getYear()const;
int getMonth()const;
int getDay()const;
void printDate()const;
int checkDay(int )const;
virtual ~Date();
private:
int year;
int month;
int day;
};
#endif // DATE_H
#include "Date.h"
#include <iostream>
#include <string>
using namespace std;
Date::Date(int y, int m, int d)
{
setDate(y,m,d);
}
Date::Date(Date &t)
{
setDate(t.getYear(),t.getMonth(),t.getDay());
}
void Date::setDate(int y, int m, int d)
{
setYear(y);
setMonth(m);
setDay(d);
}
void Date::setYear(int y)
{
year = (y >= 1900 && y <= 2100)? y : 1900;
}
void Date::setMonth(int m)
{
month = (m > 0 && m < 13)? m :1;
}
void Date::setDay(int d)
{
day = checkDay(d);
}
int Date::checkDay(int d)const
{
static const int daysPerMonth[13] =
{0,31,28,31,30,31,30,31,31,30,31,30,31};
if(d > 0 && d <= daysPerMonth[month])
return d;
if(month == 2 && day ==29 && (year % 400 == 0 || (year%4 == 0&& year % 100 != 0)))
return d;
cout << "Invalid day! set to 1" << endl;
return 1;
}
int Date::getYear()const
{
return year;
}
int Date::getMonth()const
{
return month;
}
int Date::getDay()const
{
return day;
}
void Date::printDate()const
{
string months[13] =
{" ", "January","February","March","April","May","June","July","August",
"September","October","November","December"};
cout << months[month] << "/" << day << " " << year << endl;
}
Date::~Date()
{
//dtor
}
Employee.h
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include "Date.h"
#include <string>
using namespace std;
class Employee
{
public:
Employee(string ,string ,string ,Date &);
void setBirth(int,int,int);
void setYears(int); //设置继续续聘年数
void setWages(double);
void keepHire();
void display()const;
const Date &getBirth()const;
virtual ~Employee();
private:
string num;
string name;
string ID;
Date birth;
Date hire;
int years;
double wages;
};
#endif // EMPLOYEE_H
Employee.cpp
#include "Employee.h"
#include "Date.h"
#include <iostream>
using namespace std;
//构造函数工号,姓名,身份证号,受聘日期
Employee::Employee(string tnum,string tname,string tID,Date &h)
:num(tnum),name(tname),ID(tID),hire(h)
{
string y = tID.substr(6,4);
string m = tID.substr(10,2);
string d = tID.substr(12,2);
int year = stoi(y);
int month = stoi(m);
int day = stoi(d);
birth.setDate(year,month,day);
wages = 8000.0;
years = 3;
}
void Employee::setBirth(int y,int m,int d)
{
birth.setDate(y,m,d);
}
const Date &Employee::getBirth()const //要返回的类型为const 很重要
{
return birth;
}
void Employee::setYears(int y)
{
years += y;
}
void Employee::setWages(double w)
{
wages = w;
}
void Employee::keepHire()
{
if(hire.getYear() - birth.getYear() + years < 60)
{
cout << name << " Keep hire one year!" << endl;
years++;
}
else
cout << " Sorry " + name + "age is larger than 60,cannot to hire!" << endl;
}
void Employee::display()const
{
cout << "gonghao : " << num << endl;
cout << "name : " << name << endl;
cout << "ID : " << ID << endl;
cout << "born day : ";
birth.printDate();
cout << "Employee day :";
hire.printDate();
Date expire(hire.getYear() + years,hire.getMonth(),hire.getDay());
cout << "expire day : ";
expire.printDate();
cout << "employ year : " << years << endl;
cout << "wages : " << wages << endl << endl;
}
Employee::~Employee()
{
//dtor
}
main.cpp
#include <iostream>
#include "Employee.h"
#include "Date.h"
#include <string>
using namespace std;
int main()
{
Date h1(2019,01,01);
Employee e1("000001","Jone snow","411526199001101234",h1);
//e1.getBirth().printDate();
e1.display();
e1.keepHire();
e1.setWages(12000);
e1.display();
e1.setYears(9);
e1.display();
Employee e2("000002","Sam tan","41152619603011234",h1);
e2.keepHire();
e2.display();
}