参考文章:《深度学习笔记5:池化层的实现》
池化层的理解:
池化层是卷积神经网络中常用的操作,属于前馈神经网络的一部分。主要功能:
1. 降低参数规模,防止过拟合 2. 提高模型鲁棒性(当图像有小的平移时,maxpooling结果不变)主要方法
3. Max-pooling:最大池化 4. Mean-pooling:平均池化目标矩阵的尺寸
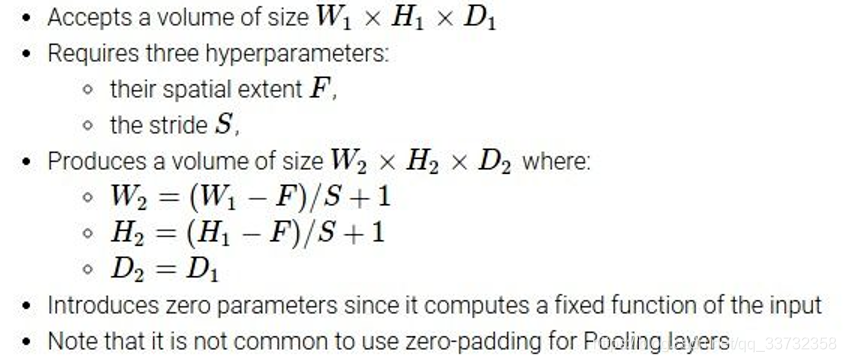C++代码实现
#include "pch.h"
#include <iostream>
#include <map>
#include <math.h>
class MaxPolling {
public:
// 最大池化函数
template <typename _Tp>
_Tp* poll(_Tp* matrix, int matrix_w, int matrix_h, int kernel_size, int stride, bool show) {
// 池化结果的size
int result_w = (matrix_w - kernel_size ) / stride + 1, result_h = (matrix_h - kernel_size ) / stride + 1;
// 申请内存
_Tp* result = (_Tp*)malloc(sizeof(_Tp)*result_w*result_h);
int x = 0, y = 0;
for (int i = 0; i < result_h; i++) {
for (int j = 0; j < result_w; j++) {
result[y*result_w + x] = getMax(matrix, matrix_w, matrix_h, kernel_size, j*stride, i*stride);
x++;
}
y++; x = 0;
}
if (show) {
showMatrix(result, result_w, result_h);
}
return result;
}
template <typename _Tp>
void showMatrix(_Tp matrix, int matrix_w, int matrix_h) {
for (int i = 0; i < matrix_h; i++) {
for (int j = 0; j < matrix_w; j++) {
std::cout << matrix[i*matrix_w + j] << " ";
}
std::cout << std::endl;
}
}
// 取kernel中最大值
template <typename _Tp>
_Tp getMax(_Tp* matrix, int matrix_w, int matrix_h, int kernel_size, int x, int y) {
int max_value = matrix[y*matrix_w + x];
for (int i = 0; i < kernel_size; i++) {
for (int j = 0; j < kernel_size; j++) {
if (max_value < matrix[matrix_w*(y + i) + x + j]) {
max_value = matrix[matrix_w*(y + i) + x + j];
}
}
}
return max_value;
}
void testMaxPolling() {
int matrix[36] = { 1,3,1,3,5,1,4,7,5,7,9,12,1,4,6,2,5,8,6,3,9,2,1,5,8,9,2,4,6,8,4,12,54,8,0,23 };
poll(matrix, 6, 6, 2, 2, true);
}
};