文章目录
- Creating and Using a Class
- Working with Classes and Instances
- Inheritance
- The `__init__()` Method for a Child Class
- Defining Attributes and Methods for the Child Class
- Overriding Methods from the Parent Class
- Instances as Attributes
- Importing Classes
- Importing a Single Class
- Storing Multiple Classes in a Module
- Importing Multiple Classes from a Module
- Importing an Entire Module
- Importing All Classes from a Module
- Importing a Module into a Module
- Styling Classes
Creating and Using a Class
Creating the Dog Class
class Dog():
"""A simple attempt to model a dog"""
def __init__(self, name, age):
"""Initialize name and age attributes"""
self.name = name
self.age = age
def sit(self):
"""Simulate a dog sitting in response to a command"""
print(self.name.title() + " is now sitting")
def roll_over(self):
"""Simulate rolling over in response to a command."""
print(self.name.title() + " rolled over!")
__init__()
This Method is a special method Python runs automatically whenever we create a new instance based on Dog
class.
This method has two leading underscores and two trailing underscores,
a convention that helps prevent Python’s default method names
from conflicting with your method names.
- underscores: 下划线
Making an Instance from a Class
# class Dog():
# --snip--
my_dog = Dog('willie', 6)
print("My dog's name is " + my_dog.name.title() + ".")
print("My dog is " + str(my_dog.age) + " years old.")
-
Accessing Attributes:
my_dog.name
-
Calling Methods:
my_dog.sit()
my_dog.roll_over()
-
Accessing Attributes:访问属性
-
Calling Methods:调用方法
Working with Classes and Instances
The Car Class
class Car():
"""A simple attempt to represent a car"""
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def get_descriptive_name(self):
"""Return a neatly formatted descriptive name"""
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
my_new_car = Car('audi', 'a4', 2016)
print(my_new_car.get_descriptive_name())
- descriptive:描述性的
- neatly:整洁地
Setting a Default Value for an Attribute
class Car():
"""A simple attempt to represent a car"""
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_descriptive_name(self):
# --snip
def get_odometer(self):
"""Print a statement showing the car's mileage"""
print("This car has " + str(self.odometer_reading) + " miles on it")
my_new_car = Car('audi', 'a4', 2016)
print(my_new_car.get_descriptive_name())
my_new_car.get_odometer()
Modifying Attribute Values
Modifying an Attribute’s Value Directly
class Car():
# --snip--
my_new_car = Car('audi', 'a4', 2016)
print(my_new_car.get_descriptive_name())
Modifying an Attribute’s Value Through a Method
class Car():
# --snip--
def update_odometer(self, mileage):
"""Set the odometer reading to the given value."""
self.odometer_reading = mileage
my_new_car = Car('audi', 'a4', 2016)
print(my_new_car.get_descriptive_name())
Inheritance
The __init__()
Method for a Child Class
class Car():
"""A simple attempt to represent a car."""
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_descriptive_name(self):
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
class ElectricCar(Car):
"""Represent aspects of a car, specific to electric vehicles"""
def __init__(self, make, model, year):
"""Initialize attributes of the parent class."""
super().__init__(make, model, year)
my_tesla = ElectricCar('tesla', 'model_s', 2016)
print(my_tesla.get_descriptive_name())
Defining Attributes and Methods for the Child Class
class Car():
# --snip--
class ElectricCar(Car):
"""Represent aspects of a car, specific to electric vehicles"""
def __init__(self, make, model, year):
"""
Initialize attributes of the parent class.
Then initialize attributes specific to the electric car.
"""
super().__init__(make, model, year)
self.battery_size = 70
def describe_battery(self):
"""Print a statement describing the battery size."""
print("This car has a " + str(self.battery_size ) + "-kWh battery")
my_tesla = ElectricCar('tesla', 'model_s', 2016)
print(my_tesla.get_descriptive_name())
Overriding Methods from the Parent Class
define a method
in the child class with the same name as the method you want to override
in the parent class.
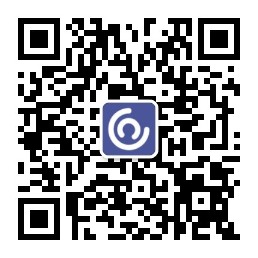
# The class Car had a method called fill_gas_tank()
def ElectricCar(Car):
--snip--
def fill_gas_tank():
"""Electric cars don't have gas tanks."""
print("This car doesn't need a gas tank!")
Instances as Attributes
class Car():
--snip--
class Battery():
"""A simple attempt to model a battery for an electric car."""
def __init__(self, battery_size=70):
"""Initialize the battery's attributes."""
self.battery_size = battery_size
def describe_battery(self):
"""Print a statement describing the battery size."""
print("This car has a " + str(self.battery_size) + "-KWh battery.")
def ElectricCar(Car):
"""Represent aspects of a car, specific to electric vehicles."""
def __init__(self, make, model, year):
"""
Initialize attributes of the parent class.
Then initialize attributes specific to the electric car.
"""
super().__init__(make, model, year)
self.battery = Battery()
my_tesla = ElectricCar('tesla', 'model s', 2016)
print(my_tesla.get_descriptive_name())
my_tesla.battery.describe_battery()
Importing Classes
Importing a Single Class
class Car():
"""A simple attempt to represent a car."""
def __init__(self, make, model, year):
"""Initialize attributes to describe a car."""
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_descriptive_name(self):
"""Return a neatly formatted descriptive name."""
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
"""Print a statement showing the car's mileage."""
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
"""
Set the odometer reading to the given value.
Reject the change if it attempts to roll the odometer back.
"""
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
"""Add the given amount to the odometer reading."""
self.odometer_reading += miles
my_car.py
from car import Car
my_new_car = Car('audi', 'a4', 2016)
print(my_new_car.get_descriptive_name())
my_new_car.odometer_reading = 23
my_new_car.read_odometer()
Storing Multiple Classes in a Module
class Car():
# --snip--
class Battery():
"""A simple attempt to model a battery for an electric car."""
def __init__(self, battery_size=60):
"""Initialize the batteery's attributes."""
self.battery_size = battery_size
def describe_battery(self):
"""Print a statement describing the battery size."""
print("This car has a " + str(self.battery_size) + "-kWh battery.")
def get_range(self):
"""Print a statement about the range this battery provides."""
if self.battery_size == 70:
range = 240
elif self.battery_size == 85:
range = 270
message = "This car can go approximately " + str(range)
message += " miles on a full charge."
print(message)
class ElectricCar(Car):
"""Models aspects of a car, specific to electric vehicles."""
def __init__(self, make, model, year):
"""
Initialize attributes of the parent class.
Then initialize attributes specific to an electric car.
"""
super().__init__(make, model, year)
self.battery = Battery()
my_electric_car.py
from car import ElectricCar
my_tesla = ElectricCar('tesla', 'model's', 2016)
print(my_tesla.get_descriptive_name())
my_tesla.battery.describe_battery()
my_tesla.battery.get_range()
Importing Multiple Classes from a Module
my_car.py
from car import Car, ElectricCar
my_beetle = Car('volkswagen', 'beetle', 2016)
print(my_beetle.get_descriptive_name())
my_tesla = ElectricCar('tesla', 'roadster', 2016)
print(my_tesla.get_descriptive_name())
Importing an Entire Module
my_car.py
import car
my_beetle = car.Car('volkswagen', 'beetle', 2016)
print(my_beetle.get_descriptive_name())
my_tesla = car.ElectricCar('tesla', 'roadster', 2016)
print(my_tesla.get_descriptive_name())
Importing All Classes from a Module
from module_name import *
Importing a Module into a Module
electric_car.py
"""A set of classes that can be used to represent electric cars."""
from car import Car
class Battery():
# --snip
class ElectricCar(Car):
# --snip
"""A class that can be used to represent a car."""
class Car():
# --snip--
my_cars.py
from car import Car
from electric_car import ElectricCar
my_beetle = Car('volkswagen', 'beetle', 2016)
print(my_beetle.get_descriptive_name())
my_tesla = ElectricCar('tesla', 'roadster', 2016)
print(my_tesla.get_descriptive_name())
Styling Classes
A few styling issues related to classes are worth clarifying, especially as your programs become more complicated.
Class names should be written in CamelCaps. To do this, capitalize the first letter of each word in the name, and don’t use underscores. Instance and module names should be written in lowercase with underscores between words.
Every class should have a docstring immediately following the class definition. The docstring should be a brief description of what the class does, and you should follow the same formatting conventions you used for writing
docstrings in functions. Each module should also have a docstring describing what the classes in a module can be used for.
You can use blank lines to organize code, but don’t use them excessively. Within a class you can use one blank line between methods, and within a module you can use two blank lines to separate classes.
If you need to import a module from the standard library and a module that you wrote, place the import statement for the standard library module first. Then add a blank line and the import statement for the module you wrote. In programs with multiple import statements, this convention makes it easier to see where the different modules used in the program come from.
capitalize :首字母大写
lowercase:小写
docstring:出现在模块、函数、类、方法里第一个语句的,就是docstring。会自动变成属性__doc__。
For example:
def foo():
""" This is function foo"""