The French author Georges Perec (1936–1982) once wrote a book, La disparition, without the letter 'e'. He was a member of the Oulipo group. A quote from the book:
Tout avait Pair normal, mais tout s’affirmait faux. Tout avait Fair normal, d’abord, puis surgissait l’inhumain, l’affolant. Il aurait voulu savoir où s’articulait l’association qui l’unissait au roman : stir son tapis, assaillant à tout instant son imagination, l’intuition d’un tabou, la vision d’un mal obscur, d’un quoi vacant, d’un non-dit : la vision, l’avision d’un oubli commandant tout, où s’abolissait la raison : tout avait l’air normal mais…
Perec would probably have scored high (or rather, low) in the following contest. People are asked to write a perhaps even meaningful text on some subject with as few occurrences of a given “word” as possible. Our task is to provide the jury with a program that counts these occurrences, in order to obtain a ranking of the competitors. These competitors often write very long texts with nonsense meaning; a sequence of 500,000 consecutive 'T's is not unusual. And they never use spaces.
So we want to quickly find out how often a word, i.e., a given string, occurs in a text. More formally: given the alphabet {'A', 'B', 'C', …, 'Z'} and two finite strings over that alphabet, a word W and a text T, count the number of occurrences of W in T. All the consecutive characters of W must exactly match consecutive characters of T. Occurrences may overlap.
Input
The first line of the input file contains a single number: the number of test cases to follow. Each test case has the following format:
- One line with the word W, a string over {'A', 'B', 'C', …, 'Z'}, with 1 ≤ |W| ≤ 10,000 (here |W| denotes the length of the string W).
- One line with the text T, a string over {'A', 'B', 'C', …, 'Z'}, with |W| ≤ |T| ≤ 1,000,000.
Output
For every test case in the input file, the output should contain a single number, on a single line: the number of occurrences of the word W in the text T.
Sample Input
3
BAPC
BAPC
AZA
AZAZAZA
VERDI
AVERDXIVYERDIAN
Sample Output
1
3
0
题意:输入两串字符串,比较下面字符串包含几个 上面的字符串 并输出;
#include<iostream>
#include<cstring>
#include<cstdio>
#include<algorithm>
#include<queue>
#include<cmath>
#include<stack>
using namespace std;
unsigned long long Hash[10010];
int t;
int base = 237;
char str1[1000010], str2[1000010];
int main()
{
scanf("%d", &t);
while(t--)
{
scanf("%s%s", str1, str2);
int sum = 0;
int len1 = strlen(str1);
int len2 = strlen(str2);
if(len1>len2)
{
printf("%d",sum);
continue;
}
unsigned long long sh1 = 0;
unsigned long long sh2 = 0;
unsigned long long hb = 1;
for(int i=0; i<len1; i++)
{
sh1 = sh1*base+str1[i]-'A';
}
for(int i=0; i<len1; i++)
{
sh2 = sh2*base+str2[i]-'A';
}
for(int i=0; i<len1; i++)
{
hb*=base;
}
for(int i=0; i+len1<=len2; i++)
{
if(sh1==sh2)
sum++;
sh2 = sh2*base-(str2[i]-'A')*hb + (str2[i+len1]-'A');
}
printf("%d\n", sum);
}
return 0;
}
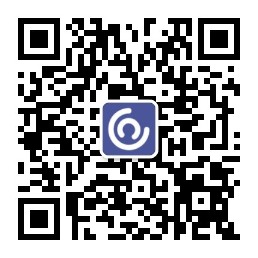
#include<iostream>
#include<cstring>
#include<cstdio>
#include<algorithm>
#include<queue>
#include<cmath>
#include<stack>
using namespace std;
char mapp1[10005], mapp2[1000005];
int next[10010] = {-5};//注意此代码将next全部初始化为-5;
//得到子串的next数组,数组next[i] = j 用来记录 在子串的第i个字符之前(不包含第i个字符)有最大长度为j的相同前缀后缀,
//例如:ABCDABCT 的next[7] = 3;
void getnext(int len2)
{
int k = -1;
int j = 0;
next[j] = k;
while(j<len2)
{
if((k==-1)||(mapp1[j]==mapp1[k]))//匹配成功的条件
{
k++;
j++;
next[j] = k;//如果匹配成功,则都往后移;
}
//如果匹配不成功,看j串是第几个不成功的,第n个不成功,则j回溯为next[n-1],找next[n-1],
//即为的k下一次的值,然后下一次从k的值处开始匹配。
else
{
k = next[k];
}
}
}
//KMP算法,用来计算子串中包含多少个父串;
int KMP(int len2, int len1)//len2是父串的长度,len1是子串的长度;
{
int i, j;
int ans = 0;
i = 0;
j = 0;
while(i<len1)
{
if((j==-1)||(mapp2[i]==mapp1[j]))
{
++i;
++j;
}
else
{
j = next[j];//将在第j个字符之前的最大相同前缀后缀赋给j;
}
if(j==len2)//如果j等于父串的长度,则说明其与父串相同,ans++;并且
{
ans++;
j = next[j-1];
i--;
}
}
return ans;
}
int main()
{
int T;
scanf("%d", &T);
while(T--)
{
int index = 0;
scanf("%s%s", mapp1, mapp2);
int len1 = strlen(mapp2);
int len2 = strlen(mapp1);
int pos = 0;
getnext(len2);
index = KMP(len2, len1);
printf("%d\n",index);
}
return 0;
}