Exercise 10.1: Least squares
Generate matrix with . Also generate some vector .
Now find .
Print the norm of the residual.
编写代码如下,调用lstsq函数求最小二乘解,再调用norm求范数即可(默认为2-范数)。
import scipy as sp
import scipy.linalg as lg
import numpy as np
def least_square():
A = np.random.random((10, 20)) * 10
b1 = np.random.random(10) * 5
x = lg.lstsq(A, b1, cond=-1)[0]
print(x)
residual = A.dot(x) - b1
print(lg.misc.norm(residual))
least_square()
Exercise 10.2: Optimization
Find the maximum of the function
编写代码如下,使用optimize的minimize_scalar函数求 的最小值,再取负即可。
import scipy.optimize as op
import math
def get_max():
fun = lambda x: math.sin(x - 2) ** 2 * math.exp(- x * x) * -1
ans = op.minimize_scalar(fun, bounds=[-5, 5])
print(ans.x, -ans.fun) #取负
get_max()
运行结果如下,同图像一致。
D:\python\python.exe D:/pycharm/projects/Matplot/scipy_exercise.py
0.21624132858697098 0.9116854118471548
Process finished with exit code 0
Exercise 10.3: Pairwise distances
Let be a matrix with rows and columns. How can you compute the pairwise distances between every two rows?
As an example application, consider cities, and we are given their coordinates in two columns. Now we want a nice table that tells us for each two cities, how far they are apart.
Again, make sure you make use of Scipy’s functionality instead of writing your own routine.
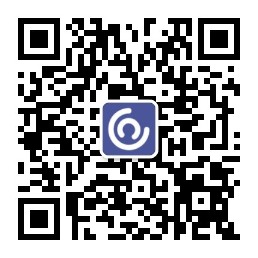
编写代码如下:
import scipy.spatial.distance as ds
import numpy as np
def pairwise_distance():
m, n =5, 2 # five cities represented by a 2-dim vector
x = np.random.randint(0, 10, (m, n))
dis_list = []
table = {}
for i in range(m):
for j in range(i + 1, m):
dis_list.append(str(i) + ' to ' + str(j))
print(x)
dis = ds.pdist(x, 'euclidean')
for ci, di in zip(dis_list, dis):
table[ci] = di
print(table)
pairwise_distance()
执行结果如下:
D:\python\python.exe D:/pycharm/projects/Matplot/scipy_exercise.py
[[6 7]
[2 1]
[0 2]
[6 5]
[1 4]]
{'0 to 1': 7.211102550927978, '0 to 2': 7.810249675906654, '0 to 3': 2.0, '0 to 4': 5.830951894845301, '1 to 2': 2.23606797749979, '1 to 3': 5.656854249492381, '1 to 4': 3.1622776601683795, '2 to 3': 6.708203932499369, '2 to 4': 2.23606797749979, '3 to 4': 5.0990195135927845}
Process finished with exit code 0