引言
除了静态内存和栈内存外,每个程序还有一个内存池,这部分内存被称为自由空间或者堆。程序用堆来存储动态分配的对象即那些在程序运行时分配的对象,当动态对象不再使用时,我们的代码必须显式的销毁它们。
在C++中,动态内存的管理是用一对运算符完成的:new和delete,new:在动态内存中为对象分配一块空间并返回一个指向该对象的指针,delete:指向一个动态独享的指针,销毁对象,并释放与之关联的内存。
动态内存管理经常会出现两种问题:一种是忘记释放内存,会造成内存泄漏;一种是尚有指针引用内存的情况下就释放了它,就会产生引用非法内存的指针。
为了更加容易(更加安全)的使用动态内存,引入了智能指针的概念。智能指针的行为类似常规指针,重要的区别是它负责自动释放所指向的对象。标准库提供的两种智能指针的区别在于管理底层指针的方法不同,shared_ptr允许多个指针指向同一个对象,unique_ptr则“独占”所指向的对象。标准库还定义了一种名为weak_ptr的伴随类,它是一种弱引用,指向shared_ptr所管理的对象,这三种智能指针都定义在memory头文件中。
什么是智能指针
C++的智能指针其实就是对普通指针的封装(即封装成一个类),通过重载 * 和 ->两个运算符,使得智能指针表现的就像普通指针一样。
智能指针的作用
C++程序设计中使用堆内存是非常频繁的操作,堆内存的申请和释放都由程序员自己管理。程序员自己管理堆内存可以提高程序的效率,但是整体来说堆内存的管理是麻烦的,C++11中引入了智能指针的概念,方便管理堆内存。使用普通指针,容易造成堆内存泄露(忘记释放),二次释放,程序发生异常时内存泄露等问题等,使用智能指针能更好的管理堆内存。
智能指针实现原理
智能指针(smart pointer)的通用实现技术是使用引用计数(reference count)。智能指针类将一个计数器与类指向的对象相关联,引用计数跟踪该类有多少个对象的指针指向同一对象。每次创建类的新对象时,初始化指针就将引用计数置为1;当对象作为另一对象的副本而创建时,拷贝构造函数拷贝指针并增加与之相应的引用计数;对一个对象进行赋值时,赋值操作符减少左操作数所指对象的引用计数(如果引用计数为减至0,则删除对象),并增加右操作数所指对象的引用计数;调用析构函数时,析构函数减少引用计数(如果引用计数减至0,则删除基础对象)。
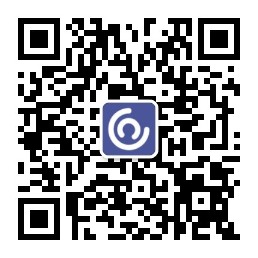
使用C++标准库中的智能指针
智能指针在C++11版本之后提供,包含在头文件<memory>中,废弃了auto_ptr,从Boost标准库中引入了shared_ptr、unique_ptr、weak_ptr三种指针。
STL一共给我们提供了四种智能指针:auto_ptr、unique_ptr、shared_ptr和weak_ptr,auto_ptr是C++98提供的解决方案,C+11已将其摒弃,并提出了unique_ptr作为auto_ptr替代方案。虽然auto_ptr已被摒弃,但在实际项目中仍可使用,但建议使用较新的unique_ptr,因为unique_ptr比auto_ptr更加安全,后文会详细叙述。shared_ptr和weak_ptr则是C+11从准标准库Boost中引入的两种智能指针。此外,Boost库还提出了boost::scoped_ptr、boost::scoped_array、boost::intrusive_ptr 等智能指针,虽然尚未得到C++标准采纳,但是实际开发工作中可以使用。
- shared_ptr
利用引用计数->每有一个指针指向相同的一片内存时,引用计数+1,每当一个指针取消指向一片内存时,引用计数-1,减为0时释放内存。
- week_ptr
弱指针 ->辅助shared_ptr解决循环引用的问题
weak_ptr是为了配合shared_ptr而引入的一种智能指针,因为它不具有普通指针的行为,没有重载operator*和->,它的最大作用在于协助shared_ptr工作,像旁观者那样观测资源的使用情况。weak_ptr可以从一个shared_ptr或者另一个weak_ptr对象构造,获得资源的观测权。但weak_ptr没有共享资源,它的构造不会引起指针引用计数的增加。使用weak_ptr的成员函数use_count()可以观测资源的引用计数,另一个成员函数expired()的功能等价于use_count()==0,但更快,表示被观测的资源 (也就是shared_ptr的管理的资源)已经不复存在。weak_ptr可以使用一个非常重要的成员函数lock()从被观测的shared_ptr 获得一个可用的shared_ptr对象, 从而操作资源。但当expired()==true的时候,lock()函数将返回一个存储空指针的shared_ptr。
- unique_ptr
“唯一”拥有其所指对象,同一时刻只能有一个unique_ptr指向给定对象(禁止拷贝、赋值),可以释放所有权,转移所有权。
智能指针的实现(继承引用计数基类,实现线程安全)
#include<iostream>
#include<mutex>
using namespace std;
/* 实现一个线程安全的智能指针 */
/* 引用计数基类 */
class Sp_counter
{
private :
size_t *_count;
std::mutex mt;
public :
Sp_counter()
{
cout<<"父类构造,分配counter内存"<<endl;
_count = new size_t(0);
}
virtual ~Sp_counter()
{
if(_count && !(*_count) ){
cout<<"父类析构"<<endl;
cout<<"[释放counter内存]"<<endl;
delete _count;
_count = NULL;
}
}
Sp_counter &operator=(Sp_counter &spc)
{
cout<<"父类重载="<<endl;
cout<<"[释放counter内存]"<<endl;
delete _count;
this->_count = spc._count;
return *this;
}
Sp_counter &GetCounter()
{
return *this;
}
size_t Get_Reference()
{
return *_count;
}
virtual void Increase()
{
mt.lock();
(*_count)++;
//cout<<"_count++:"<<*_count<<endl;
mt.unlock();
}
virtual void Decrease()
{
mt.lock();
(*_count)--;
//cout<<"_count--:"<<*_count<<endl;
mt.unlock();
}
};
template<typename T>
class smart_pointer : public Sp_counter
{
private :
T *_ptr;
public :
smart_pointer(T *ptr = NULL);
~smart_pointer();
smart_pointer(smart_pointer<T> &);
smart_pointer<T> &operator=(smart_pointer<T> &);
T &operator*();
T *operator->(void);
size_t use_count();
};
/* 子类参构造函数&带参数构造函数 */
template<typename T>
inline smart_pointer<T>::smart_pointer(T *ptr)
{
if(ptr){
cout<<"子类默认构造"<<endl;
_ptr = ptr;
this->Increase();
}
}
/* 子类析构函数 */
template<typename T>
smart_pointer<T>::~smart_pointer()
{
/* 指针非空才析构 */
if(this->_ptr){
cout<<"子类析构,计数减1"<<endl;
if(this->Get_Reference())
this->Decrease();
if(!(this->Get_Reference()) ){
cout<<"(((子类析构,主内存被释放)))"<<endl;
delete _ptr;
_ptr = NULL;
}
}
}
/* 得到引用计数值 */
template<typename T>
inline size_t smart_pointer<T>::use_count()
{
return this->Get_Reference();
}
/* 拷贝构造 */
template<typename T>
inline smart_pointer<T>::smart_pointer(smart_pointer<T> &sp)
{
cout<<"子类拷贝构造"<<endl;
/* 防止自己对自己的拷贝 */
if(this != &sp){
this->_ptr = sp._ptr;
this->GetCounter() = sp.GetCounter();
this->Increase();
}
}
/* 赋值构造 */
template<typename T>
inline smart_pointer<T> &smart_pointer<T>::operator=(smart_pointer<T> &sp)
{
/* 防止自己对自己的赋值以及指向相同内存单元的赋值 */
if(this != &sp){
cout<<"赋值构造"<<endl;
/* 如果不是构造一个新智能指针并且两个只能指针不是指向同一内存单元 */
/* =左边引用计数减1,=右边引用计数加1 */
if(this->_ptr && this->_ptr != sp._ptr){
this->Decrease();
/* 引用计数为0时 */
if(!this->Get_Reference()){
cout<<"引用计数为0,主动调用析构"<<endl;
this->~smart_pointer();
//this->~Sp_counter();
cout<<"调用完毕"<<endl;
}
}
this->_ptr = sp._ptr;
this->GetCounter() = sp.GetCounter();
this->Increase();
}
return *this;
}
/* 重载解引用*运算符 */
template<typename T>
inline T &smart_pointer<T>::operator*()
{
return *(this->_ptr);
}
template<typename T>
inline T *smart_pointer<T>::operator->(void)
{
return this->_ptr;
}
int main()
{
int *a = new int(10);
int *b = new int(20);
cout<<"-------------默认构造测试----------------->"<<endl;
cout<<"构造sp"<<endl;
smart_pointer<int> sp(a);
cout<<"sp.use_count:"<<sp.use_count()<<endl;
cout<<"------------------------------------------>"<<endl<<endl;
{
cout<<"-------------拷贝构造测试----------------->"<<endl;
cout<<"构造sp1 :sp1(sp)"<<endl;
smart_pointer<int> sp1(sp);
cout<<"构造sp2 :sp2(sp)"<<endl;
smart_pointer<int> sp2(sp1);
cout<<"sp1和sp2引用计数为3才是正确的"<<endl;
cout<<"sp1.use_count:"<<sp1.use_count()<<endl;
cout<<"sp2.use_count:"<<sp2.use_count()<<endl;
cout<<"------------------------------------------>"<<endl<<endl;
cout<<"调用析构释放sp1,sp2"<<endl;
}
cout<<"-------------析构函数测试----------------->"<<endl;
cout<<"此处sp.use_count应该为1才是正确的"<<endl;
cout<<"sp.use_count:"<<sp.use_count()<<endl;
cout<<"------------------------------------------>"<<endl<<endl;
cout<<"-------------赋值构造测试----------------->"<<endl;
cout<<"构造sp3 :sp3(b)"<<endl;
smart_pointer<int> sp3(b);
cout<<"sp3.use_count:"<<sp3.use_count()<<endl;
cout<<"sp3 = sp"<<endl;
sp3 = sp;
cout<<"sp3先被释放,然后sp3引用计数为2才正确,sp的引用计数为2才正确"<<endl;
cout<<"sp3.use_count:"<<sp3.use_count()<<endl;
cout<<"sp.use_count :"<<sp.use_count()<<endl;
cout<<"------------------------------------------>"<<endl<<endl;
cout<<"-------------解引用测试----------------->"<<endl;
cout<<"*sp3:"<<*sp3<<endl;
cout<<"*sp3 = 100"<<endl;
*sp3 = 100;
cout<<"*sp3:"<<*sp3<<endl;
cout<<"------------------------------------------>"<<endl;
// cout<<"sp3.use_count:"<<sp3.use_count()<<endl;
//cout<<"sp.use_count:"<<sp.use_count()<<endl;
cout<<"===================end main===================="<<endl;
return 0;
}
上面的实现是通过继承引用计数基类实现的智能指针,把引用计数写到智能指针类里,也可实现。
下面是一个基于引用计数的智能指针的实现,需要实现构造,析构,拷贝构造,=操作符重载,重载*-和>操作符。代码如下,
template <typename T>
class SmartPointer {
public:
//构造函数
SmartPointer(T* p=0): _ptr(p), _reference_count(new size_t){
if(p)
*_reference_count = 1;
else
*_reference_count = 0;
}
//拷贝构造函数
SmartPointer(const SmartPointer& src) {
if(this!=&src) {
_ptr = src._ptr;
_reference_count = src._reference_count;
(*_reference_count)++;
}
}
//重载赋值操作符
SmartPointer& operator=(const SmartPointer& src) {
if(_ptr==src._ptr) {
return *this;
}
releaseCount();
_ptr = src._ptr;
_reference_count = src._reference_count;
(*_reference_count)++;
return *this;
}
//重载操作符
T& operator*() {
if(ptr) {
return *_ptr;
}
//throw exception
}
//重载操作符
T* operator->() {
if(ptr) {
return _ptr;
}
//throw exception
}
//析构函数
~SmartPointer() {
if (--(*_reference_count) == 0) {
delete _ptr;
delete _reference_count;
}
}
private:
T *_ptr;
size_t *_reference_count;
void releaseCount() {
if(_ptr) {
(*_reference_count)--;
if((*_reference_count)==0) {
delete _ptr;
delete _reference_count;
}
}
}
};
int main()
{
SmartPointer<char> cp1(new char('a'));
SmartPointer<char> cp2(cp1);
SmartPointer<char> cp3;
cp3 = cp2;
cp3 = cp1;
cp3 = cp3;
SmartPointer<char> cp4(new char('b'));
cp3 = cp4;
}
总结
- 你知道智能指针吗?智能指针的原理。
- 常用的智能指针
- 智能指针的实现(见上)
1.答案:智能指针是一个类,这个类的构造函数中传入一个普通指针,析构函数中释放传入的指针。智能指针的类都是栈上的对象,所以当函数(或程序)结束时会自动被释放,
2.最常用的智能指针:
1)std::auto_ptr,有很多问题。 不支持复制(拷贝构造函数)和赋值(operator =),但复制或赋值的时候不会提示出错。因为不能被复制,所以不能被放入容器中。
2) C++11引入的unique_ptr, 也不支持复制和赋值,但比auto_ptr好,直接赋值会编译出错。实在想赋值的话,需要使用:std::move。
例如:
std::unique_ptr<int> p1(new int(5));
std::unique_ptr<int> p2 = p1; // 编译会出错
std::unique_ptr<int> p3 = std::move(p1); // 转移所有权, 现在那块内存归p3所有, p1成为无效的指针.
3) C++11或boost的shared_ptr,基于引用计数的智能指针。可随意赋值,直到内存的引用计数为0的时候这个内存会被释放。但这个模型使用时可能会出现循环引用问题,可以使用weak_ptr辅助解决。
4)C++11或boost的weak_ptr,弱引用。 引用计数有一个问题就是互相引用形成环,这样两个指针指向的内存都无法释放。需要手动打破循环引用或使用weak_ptr。顾名思义,weak_ptr是一个弱引用,只引用,不计数。如果一块内存被shared_ptr和weak_ptr同时引用,当所有shared_ptr析构了之后,不管还有没有weak_ptr引用该内存,内存也会被释放。所以weak_ptr不保证它指向的内存一定是有效的,在使用之前需要检查weak_ptr是否为空指针。
5)Boost中的scoped_ptr,scoped和weak_ptr的区别就是,给出了拷贝和赋值操作的声明并没有给出具体实现,并且将这两个操作定义成私有的,这样就保证scoped_ptr不能使用拷贝来构造新的对象也不能执行赋值操作,更加安全,但有了”++”“–”以及“*”“->”这些操作,比weak_ptr能实现更多功能。
前向声明时的交叉循环引用问题
考虑一个简单的对象建模——家长与子女:a Parent has a Child, a Child knowshis/her Parent。在Java 里边很好写,不用担心内存泄漏,也不用担心空悬指针,只要正确初始化myChild 和myParent,那么Java 程序员就不用担心出现访问错误。一个handle 是否有效,只需要判断其是否non null。
public class Parent
{
private Child myChild;
}
public class Child
{
private Parent myParent;
}
在C++ 里边就要为资源管理费一番脑筋。如果使用原始指针作为成员,Child和Parent由谁释放?那么如何保证指针的有效性?如何防止出现空悬指针?这些问题是C++面向对象编程麻烦的问题,现在可以借助smart pointer把对象语义(pointer)转变为值(value)语义,shared_ptr轻松解决生命周期的问题,不必担心空悬指针。但是这个模型存在循环引用的问题,注意其中一个指针应该为weak_ptr。
//原始指针的做法,容易出错
#include <iostream>
#include <memory>
class Child;
class Parent;
class Parent {
private:
Child* myChild;
public:
void setChild(Child* ch) {
this->myChild = ch;
}
void doSomething() {
if (this->myChild) {
}
}
~Parent() {
delete myChild;
}
};
class Child {
private:
Parent* myParent;
public:
void setPartent(Parent* p) {
this->myParent = p;
}
void doSomething() {
if (this->myParent) {
}
}
~Child() {
delete myParent;
}
};
int main() {
{
Parent* p = new Parent;
Child* c = new Child;
p->setChild(c);
c->setPartent(p);
delete c; //only delete one
}
return 0;
}
//循环引用内存泄漏问题
#include <iostream>
#include <memory>
class Child;
class Parent;
class Parent {
private:
std::shared_ptr<Child> ChildPtr;
public:
void setChild(std::shared_ptr<Child> child) {
this->ChildPtr = child;
}
void doSomething() {
if (this->ChildPtr.use_count()) {
}
}
~Parent() {
}
};
class Child {
private:
std::shared_ptr<Parent> ParentPtr;
public:
void setPartent(std::shared_ptr<Parent> parent) {
this->ParentPtr = parent;
}
void doSomething() {
if (this->ParentPtr.use_count()) {
}
}
~Child() {
}
};
int main() {
std::weak_ptr<Parent> wpp;
std::weak_ptr<Child> wpc;
{
std::shared_ptr<Parent> p(new Parent);
std::shared_ptr<Child> c(new Child);
p->setChild(c);
c->setPartent(p);
wpp = p;
wpc = c;
std::cout << p.use_count() << std::endl; // 2
std::cout << c.use_count() << std::endl; // 2
}
std::cout << wpp.use_count() << std::endl; // 1
std::cout << wpc.use_count() << std::endl; // 1
return 0;
}
//正确的做法
#include <iostream>
#include <memory>
class Child;
class Parent;
class Parent {
private:
//std::shared_ptr<Child> ChildPtr;
std::weak_ptr<Child> ChildPtr;
public:
void setChild(std::shared_ptr<Child> child) {
this->ChildPtr = child;
}
void doSomething() {
//new shared_ptr
if (this->ChildPtr.lock()) {
}
}
~Parent() {
}
};
class Child {
private:
std::shared_ptr<Parent> ParentPtr;
public:
void setPartent(std::shared_ptr<Parent> parent) {
this->ParentPtr = parent;
}
void doSomething() {
if (this->ParentPtr.use_count()) {
}
}
~Child() {
}
};
int main() {
std::weak_ptr<Parent> wpp;
std::weak_ptr<Child> wpc;
{
std::shared_ptr<Parent> p(new Parent);
std::shared_ptr<Child> c(new Child);
p->setChild(c);
c->setPartent(p);
wpp = p;
wpc = c;
std::cout << p.use_count() << std::endl; // 2
std::cout << c.use_count() << std::endl; // 1
}
std::cout << wpp.use_count() << std::endl; // 0
std::cout << wpc.use_count() << std::endl; // 0
return 0;
}