#include <unistd.h>
int chdir(const char *path);
int fchdir(int fd);
作用:改变调用这一函数的进程(即程序执行)的当前工作目录,注意不是shell的当前工作目录。
返回值:0成功 -1失败
#include <unistd.h>
char *getcwd(char *buff, size_t size);
作用:获取当前进程的当前工作目录。在返回值或者buff中。
返回值:成功则返回进程的当前工作目录;失败,返回NULL 且可通过perror和errno查看详细错误情况。
[root@localhost dir_op]# gcc -pipe -Wall -pedantic -ggdb3 chdir.c -o chdir
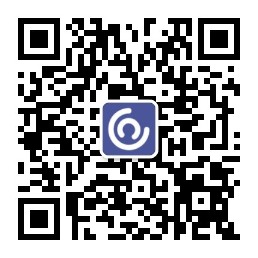
//代码
#include <stdio.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char* argv[])
{
if(argc < 2)
{
printf("a.out dir\n");
exit(1);
}
int ret = chdir(argv[1]); //改变当前进程的工作目录
if(ret == -1)
{
perror("chdir");
exit(1);
}
int fd = open("chdir.txt", O_CREAT | O_RDWR, 0777); //在改变的目录中新创建一个文件
if(fd == -1)
{
perror("open");
exit(1);
}
close(fd);
char buf[128];
getcwd(buf, sizeof(buf)); //获取进程的当前工作目录
printf("current dir: %s\n", buf); //printf是行缓冲,因此注意最后加上换行符,且换行符也会进行输出,而0、\0和NULL不会输出(都视为字符串结束符,无效)。
return 0;
}
[root@localhost dir_op]# ./chdir ../
current dir: /mnt/hgfs/share/work/01_DEMO
[root@localhost dir_op]# cd ../
[root@localhost 01_DEMO]# ls //可见改变了工作目录
chdir.txt demo dir_op dup english.txt file_op stat.c
#include <sys/stat.h>
#include <sys/types.h>
int mkdir(const char *pathname, mode_t mode);
作用:创建目录。注意创建的目录必须具有执行权限,否则无法进入目录。
第一个形参为目录,第二个为给予的权限。
返回值:0 成功 -1 失败
//代码
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
int main(int argc, char* argv[])
{
if(argc < 3)
{
printf("a.out newDir mode\n");
exit(1);
}
int mode = strtol(argv[2], NULL, 8); //命令行参数为字符串,必须将其转化为8进制整型数,如0777
int ret = mkdir(argv[1], mode); //创建一个目录
if(ret == -1)
{
perror("mkdir");
exit(1);
}
return 0;
}
#include <unistd.h>
int rmdir(const char *pathname);
作用:只能删除空目录
返回值:0成功 -1失败