1、js方法
function exporterExcel(){ var no=$("#serchNo").val(); var machineImei=$("#serchMachineImei").val(); var orderNo=$("#serchOrderNo").val(); var status=$("#serchStatus").val(); var dealResult=$("#serchDealResult").val(); var url="../saleAfterMaintainAction/exporterExcel.shtml?queryParam['no']="+no+"&queryParam['machineImei']="+machineImei+"&queryParam['orderNo']="+orderNo+"&queryParam['status']="+status+"&queryParam['dealResult']="+dealResult; window.open(url); }
2、Action
@RequestMapping(value = "/exporterExcel") @ResponseBody public ResultVO exportExcel(QueryVO queryVO, HttpServletRequest request, HttpServletResponse response) { saleAfterMaintainService.exportExcel(queryVO, request, response); return success("导出成功"); }
3、Service
public void exportExcel(QueryVO queryVO, HttpServletRequest req, HttpServletResponse res) { int totalCount = saleAfterMaintainDao.queryCount(queryVO);//数据库查询数据条数 int rows=10; long start = System.currentTimeMillis(); try { LinkedHashMap<String, String> headMap = new LinkedHashMap<String, String>(); headMap.put("no", "保修单号"); headMap.put("name", "姓名"); headMap.put("tel", "电话"); headMap.put("address", "地址"); headMap.put("reason", "保修原因"); headMap.put("machineImei", "设备IMEI"); headMap.put("orderNo", "对应订单号"); headMap.put("status", "状态"); headMap.put("dealResult", "处理结果"); headMap.put("createTime", "创建时间"); // 开始excel生成 SXSSFWorkbook wb = new SXSSFWorkbook(100); Sheet sh = wb.createSheet(); // 表格样式 CellStyle cellStyle = ExportExcel.getContentStyle(wb); // 设置表格宽度 setShColumnWidth(sh); // 循环数据 Row row = sh.createRow(0); int headNum = 0; for (String key : headMap.keySet()) { Cell cell = row.createCell(headNum); cell.setCellValue(headMap.get(key)); cell.setCellStyle(cellStyle); headNum++; } rows = 1000; int pageCount = totalCount % rows == 0 ? totalCount / rows : totalCount / rows + 1; int rowNum = 1; for (int i = 0; i < pageCount; i++) { List<Map<String, Object>> pageList = saleAfterMaintainDao.findPageReturn(queryVO, pageCount, rows);//数据库查询数据 for (Map<String, Object> map : pageList) { row = sh.createRow(rowNum); int index = 0; for (String key : headMap.keySet()) { Cell cell = row.createCell(index); ExportExcel.setCellValue(cell, map, key); cell.setCellStyle(cellStyle); index++; } rowNum++; } } String prePath = req.getSession().getServletContext().getRealPath("/"); File file = new File(prePath); if (!file.exists()) file.mkdirs(); String filePath = prePath + "保修单.xlsx"; FileOutputStream out = new FileOutputStream(filePath); wb.write(out); out.close(); wb.dispose(); ExportExcel.openExcel(req, res, "保修单.xlsx"); LogUtil.writeLog("导出excel的时间:" + (System.currentTimeMillis() - start) / 1000 + "秒"); } catch (Exception e) { LogUtil.writeErrorLog(e.getMessage(), e); } } /** * 设置表格的宽度 * * @param sh */ private void setShColumnWidth(Sheet sh) { sh.setColumnWidth(0, 6000); sh.setColumnWidth(1, 4000); sh.setColumnWidth(2, 4000); sh.setColumnWidth(3, 4000); sh.setColumnWidth(4, 5000); sh.setColumnWidth(5, 5000); sh.setColumnWidth(6, 3000); sh.setColumnWidth(7, 2000); sh.setColumnWidth(8, 5000); sh.setColumnWidth(9, 2000); sh.setColumnWidth(10, 4000); sh.setColumnWidth(11, 6000); sh.setColumnWidth(12, 5000); sh.setColumnWidth(13, 5000); sh.setColumnWidth(14, 5000); sh.setColumnWidth(15, 5000); sh.setColumnWidth(16, 4000); sh.setColumnWidth(17, 5000); }
4、ExportExcel 类
package com.xg.util; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.net.URLEncoder; import java.text.SimpleDateFormat; import java.util.Date; import java.util.LinkedHashMap; import java.util.List; import java.util.Map; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.CellStyle; import org.apache.poi.ss.usermodel.Font; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.ss.usermodel.Sheet; import org.apache.poi.xssf.streaming.SXSSFWorkbook; import com.pay.util.LogUtil; import com.xg.dao.BaseDao; import com.xg.framework.vo.query.QueryVO; public class ExportExcel { /** * 弹出打开框 * * @param request * @param response * @param downName */ public static void openExcel(HttpServletRequest request, HttpServletResponse response, String downName) { BufferedInputStream bis = null; BufferedOutputStream bos = null; String filepath = null; try { filepath = request.getSession().getServletContext().getRealPath("/") + downName; bis = new BufferedInputStream(new FileInputStream(new File(filepath))); bos = new BufferedOutputStream(response.getOutputStream()); response.setHeader("Content-disposition", "attachment;filename=" + URLEncoder.encode(downName, "utf-8")); int bytesRead = 0; byte[] buffer = new byte[8192]; while ((bytesRead = bis.read(buffer, 0, 8192)) != -1) { bos.write(buffer, 0, bytesRead); } bos.flush(); } catch (FileNotFoundException e) { LogUtil.writeErrorLog(e.getMessage(), e); } catch (IOException e) { LogUtil.writeErrorLog(e.getMessage(), e); } finally { if (null != bis) try { bis.close(); } catch (IOException e) { LogUtil.writeErrorLog(e.getMessage(), e); } if (null != bos) try { bos.close(); } catch (IOException e) { LogUtil.writeErrorLog(e.getMessage(), e); } new File(filepath).delete(); } } public static CellStyle getContentStyle(SXSSFWorkbook wb) { CellStyle cellStyle = wb.createCellStyle(); cellStyle.setBorderBottom(CellStyle.BORDER_THIN); // 下边框 cellStyle.setBorderLeft(CellStyle.BORDER_THIN);// 左边框 cellStyle.setBorderTop(CellStyle.BORDER_THIN);// 上边框 cellStyle.setBorderRight(CellStyle.BORDER_THIN);// 右边框 cellStyle.setAlignment(CellStyle.ALIGN_CENTER); // 水平居中 cellStyle.setAlignment(CellStyle.VERTICAL_CENTER); // 垂直居中 Font font = wb.createFont(); font.setFontName("宋体");// 设置字体 font.setFontHeightInPoints((short) 10);// 设置字体大小 cellStyle.setFont(font); return cellStyle; } /** * 表格赋值 * * @param cell * @param map * @param key */ public static void setCellValue(Cell cell, Map<String, Object> map, String key) { Object value = map.get(key); if (value == null) { cell.setCellValue(""); } else if (value instanceof Double) { cell.setCellValue(((Double) value).doubleValue()); } else if (value instanceof Date) { Date date = (Date) value; SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); cell.setCellValue(sdf.format(date)); } else { String reg = "^\\d+$"; String keyValue = value.toString(); boolean isNum = keyValue.matches(reg); if (isNum) { cell.setCellValue(Double.valueOf(keyValue)); } else { cell.setCellValue(value.toString()); } } } /** * excel导出 * * @param excelWith * * @param machineComboOrderDao * @param queryVO * @param req * @param res * @param headMap * @param excelName * @throws FileNotFoundException * @throws IOException */ @SuppressWarnings({ "rawtypes", "unchecked" }) public static void doExportData(BaseDao baseDao, int[] excelWith, QueryVO queryVO, HttpServletRequest req, HttpServletResponse res, LinkedHashMap<String, String> headMap, String excelName) throws FileNotFoundException, IOException { // 总条数 int totalCount = baseDao.queryCount(queryVO); int rows = 10; long start = System.currentTimeMillis(); // 开始excel生成 SXSSFWorkbook wb = new SXSSFWorkbook(100); Sheet sh = wb.createSheet(); // 表格样式 CellStyle cellStyle = getContentStyle(wb); // 设置表格宽度 setShColumnWidth(sh, excelWith); // 循环数据 Row row = sh.createRow(0); int headNum = 0; for (String key : headMap.keySet()) { Cell cell = row.createCell(headNum); cell.setCellValue(headMap.get(key)); cell.setCellStyle(cellStyle); headNum++; } rows = 1000; int pageCount = totalCount % rows == 0 ? totalCount / rows : totalCount / rows + 1; int rowNum = 1; for (int i = 0; i < pageCount; i++) { List<Map<String, Object>> pageList = baseDao.findPageReturn(queryVO, pageCount, rows); for (Map<String, Object> map : pageList) { row = sh.createRow(rowNum); int index = 0; for (String key : headMap.keySet()) { Cell cell = row.createCell(index); ExportExcel.setCellValue(cell, map, key); cell.setCellStyle(cellStyle); index++; } rowNum++; } } String prePath = req.getSession().getServletContext().getRealPath("/"); File file = new File(prePath); if (!file.exists()) file.mkdirs(); String filePath = prePath + excelName; FileOutputStream out = new FileOutputStream(filePath); wb.write(out); out.close(); wb.dispose(); openExcel(req, res, excelName); LogUtil.writeLog("导出excel的时间:" + (System.currentTimeMillis() - start) / 1000 + "秒");//记入日志 } /** * 设置表格宽度 * * @param sh * @param excelWith */ private static void setShColumnWidth(Sheet sh, int[] excelWith) { for (int i = 0; i < excelWith.length; i++) { sh.setColumnWidth(i, excelWith[i]); } } }
5、jar包
poi-examples-3.13-20150929.jar
poi-excelant-3.13-20150929.jar
poi-ooxml-3.13-20150929.jar
poi-ooxml-schemas-3.13-20150929.jar
poi-scratchpad-3.13-20150929.jar
扫描二维码关注公众号,回复:
555796 查看本文章
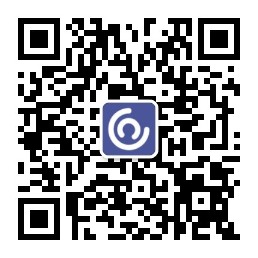