CS560/460 Spring 2019
Programming Assignment 1
(Due February 12, 2019 by 11:59pm)
1.Geometric Primitives Drawing
Description: The line drawing function is the basic function provided by many graphics tools, such as the MS Word, power point, etc. A number of classic algorithms have been introduced, e.g., mid-point algorithm, Bresenham algorithm, etc. In this assignment you are required to design a program to realize the interactive freeform line drawing using the basic algorithms. In the same time, you are required to implement the line drawing function using OpenGL as well. Therefore, both results can be compared and verified each other. This assignment is designed to let you get familiar with the basic OpenGL programming, and enhance the concept of the classic can line conversion algorithms for drawing geometric primitives.
Your implementation: It is possible to implement a version of the line drawing algorithm for all octants with only one iteration loop. In this assignment, you will write a program to draw letters “WAKE” and an XYZ coordinate system, for example:
(Line Stipple with different width)
Definition: Left button click (LButtonDown): select control points.
Mouse moving without clicking any button (MouseMove): draw a line from a previously selected control point to the current cursor position. Since the mouse is freely moved, the line drawing is dynamically refreshed until the left button is clicked again.
Right button click (RButtonDown): end of control point selection.
More specifically, your program will do three things:
1)It will draw the letters “WAKE” automatically on the left-hand side of the window using your own implementation of the specified line drawing algorithm.
2)It will draw the letters “WAKE” automatically on the right-hand side of the window using OpenGL’s line drawing functions (GL_LINES).
3)It will allow the user to draw a series of connected line segments using the mouse anywhere in the window. In other words, the user will click to create control points, and the program will draw the lines between the first point and the second, the second and third, the third and the fourth, etc.:
a.Left button click (LButtonDown): add a new control point
b.Mouse moving without clicking any buttons (MouseMove): draw a line from the previously added control point to the current cursor position. The line drawing should be refreshed dynamically as the mouse moves until the left button is clicked again.
c.Right button click (RButtonDown): add last control point. Your program should draw all the line segments created up to this point until the user clicks the left button again. If the user clicks the left button again after clicking the right button, the last line segment list should be cleared and a new line segment list started.
NOTE:
(a)You can use the MS Visual Studio MFC and OpenGL to do the implementation.
(b)If you use GLUT, you can use GLUT’s double-buffering (to avoid flickering) and glutIdleFunc() to draw the two versions of WAKE and the user-drawn line segments on each render loop. You can also use the mouse callback functions to update the data in the control point list for the user-drawn line segments.
(c)Note that the line can be defined by different styles (e.g., solid line and stipple line with different width and/or color).
(1)For CS560: (a) Using mid-point based Gupta-Sproull algorithm to display the anti-aliased lines, drawing letters “WAKE” and an XYZ coordinate system. The same letters are drawn simultaneously using OpenGL with a certain distance translation. (b) Design an algorithm to draw a circle with specified radius centered at current cursor position. Extra points: (a) design an algorithm to draw an ellipse with specified radius centered at current cursor position. (b) Draw anti-aliased characters “UP”; (c) design an algorithm to draw a parabola and combine the circles to create the following figure.
(2)For CS460: (1) Using Bresenham algorithm to draw the letters “WAKE” and an XYZ coordinate system. The same letters are drawn simultaneously using OpenGL with a certain distance translation. (2) Using the mid-point algorithm to implement above line drawing.
Extra points: (a) design an algorithm to draw a circle and an ellipse with specified radius centered at current cursor position. (b) Draw anti-aliased characters “UP”; (c) design an algorithm to draw a parabola and combine the circles to create the above “happy face” figure.
2.Submission:
代做CS560/460作业
The submission of your assignment includes two parts: code and report.
Code package: your program package (including source code, and executable), similar to the sample program package provided in the course website.
Write-up (report): In your hand-in you should document the way in which you solved the problem. This documentation should describe your solution so that the reader understands the problem that you are solving and then understands the code that you hand in. It should include
(1)problem statement
(2)algorithm design (with explanation of the major code if necessary)
(3)instruction on how to run your program.
(4)sample images from screen shots.
Your program package and the assignment report must be compressed in a single ZIP file, and submit it to the digital drop-box of the blackboard on/before the due date. The TA of this course will run your program and examine your report.
Your file must be named as follows:
YourLastName_CS560_HW1.zip
or
YourLastName_CS460_HW1.zip
3. Mark distribution
For CS560: your assignment will be marked as follows
Demo and Code (90%):
Mid-point based anti-aliasing line drawing (30%)
Circle drawing (30%)
Line drawing using OpenGL (15%)
Interaction (mouse clicking) and various line styles (15%)
Write-up:
Clearly explain your code design, the solution to the problem
you have solved, and sample images (10%)
Extra points: (a) 8% (b) 8% (c) 10%
For CS460: your assignment will be marked as follows
Demo and Code (90%):
Bresenham line drawing algorithm (30%)
Mid-point line drawing algorithm (30%)
Line drawing using OpenGL (15%)
Interaction (mouse clicking) and various line styles (15%)
Write-up:
Clearly explain your code design, the solution to the problem
you have solved, and the sample images (10%)
Extra points: (a) 8% (b) 8% (c) 10%
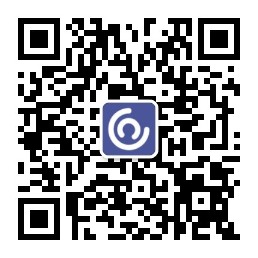
In order to get a high mark on the assignment you are required to show a good write-up and a good demo. It requires a good design and implementation. Good luck!
Note:
1. The window area is partitioned into two parts.
(1)Right side of the window: draw lines with OpenGL API.
(2)Left side of the window: automatically draw same lines with MFC or OpenGL but by your own algorithm, so as to contrast with (1)
2. The point picked by mouse is with respect to the upper-left corner;
OpenGL takes the lower-left corner as the origin. Therefore, the coordinate of “(y of OpenGL)” is equal to the “Height – (y of mouse-picked)”.
3. OpenGL:
glVertex2f(); glColor3f(); glLineWidth(); glLineStipple();
因为专业,所以值得信赖。如有需要,请加QQ:99515681 或邮箱:[email protected]
微信:codinghelp