人工智能和人工神经网络,提到这些可能有很多人都觉得很高深,很高级。但其实也有简单的,比如BP神经网络,就目前的人工神经网络发展看,除了深度学习算法的人工神经网络以外,应用最广泛的就是BP神经网络,BP神经网络能够快速发现并学习具备线性回归特征的问题。相信也有很多人想把它用在彩票分析上,处于爱好和玩的原因,我就来做一个实现。
BP神经网络的关键参数一般有3个,输入节点个数,隐藏节点个数,输出节点个数。双色球,自然输入输出都是7了。基本想法是,根据前一期的号码,推算下一期的号码。这样训练样本也很丰富,历史的中奖号码按照出奖顺序,依次传入作为训练样本即可。比较容易操作。
接下来,就是组织数据格式,要符合BP网络的算法要求了,BP神经网络训练时,只能接受小数作为输入,也就是输入数据的单向必须小于1.这好办,只需要将双色球的中奖号码除以100,形成一个2位小数,输出结果也是2位小数。甚至不需要乘100的操作,想必也能直接看懂结果
说动手就动手,先搞个BP神经网络的实现。如下:
- package ghost.writer.logic;
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileOutputStream;
- import java.io.IOException;
- import java.io.ObjectInputStream;
- import java.io.ObjectOutputStream;
- public class BPFactory {
- /**
- * BP神经网络元
- */
- private static BP bp;
- /**
- * 初始化一个全新的bp神经网络
- * @param inputSize
- * @param hiddenSize
- * @param outputSize
- */
- public static void initialization(int inputSize,int hiddenSize,int outputSize) {
- bp=new BP(inputSize, hiddenSize, outputSize);
- }
- /**
- * 从文件数据中读取bp神经网络
- * @param file
- * @throws IOException
- * @throws ClassNotFoundException
- */
- public static void initialization(File file) throws IOException, ClassNotFoundException {
- FileInputStream fi = new FileInputStream(file);
- ObjectInputStream si = new ObjectInputStream(fi);
- bp = (BP) si.readObject();
- si.close();
- }
- /**
- * 将目前的神经网络储存在指定文件
- * @param file
- * @throws IOException
- */
- public static void save(File file) throws IOException {
- FileOutputStream fo = new FileOutputStream(file);
- ObjectOutputStream so = new ObjectOutputStream(fo);
- so.writeObject(bp);
- so.close();
- }
- /**
- * 训练BP神经网络
- * @param trainData
- * @param target
- */
- public static void train(double[] trainData, double[] target) {
- bp.train(trainData, target);
- }
- /**
- * 要求bp神经网络返回预测值
- * @param inData
- * @return
- */
- public static double[] test(double[] inData) {
- return bp.test(inData);
- }
- }
聪明的同学可能已经发现了,上面只是个工厂类,我们继续:
- package ghost.writer.logic;
- import java.io.Serializable;
- import java.util.Random;
- /**
- * BPNN.
- *
- * @author RenaQiu
- *
- */
- public class BP implements Serializable {
- /**
- *
- */
- private static final long serialVersionUID = 1L;
- /**
- * input vector.
- */
- private final double[] input;
- /**
- * hidden layer.
- */
- private final double[] hidden;
- /**
- * output layer.
- */
- private final double[] output;
- /**
- * target.
- */
- private final double[] target;
- /**
- * delta vector of the hidden layer .
- */
- private final double[] hidDelta;
- /**
- * output layer of the output layer.
- */
- private final double[] optDelta;
- /**
- * learning rate.
- */
- private final double eta;
- /**
- * momentum.
- */
- private final double momentum;
- /**
- * weight matrix from input layer to hidden layer.
- */
- private final double[][] iptHidWeights;
- /**
- * weight matrix from hidden layer to output layer.
- */
- private final double[][] hidOptWeights;
- /**
- * previous weight update.
- */
- private final double[][] iptHidPrevUptWeights;
- /**
- * previous weight update.
- */
- private final double[][] hidOptPrevUptWeights;
- public double optErrSum = 0d;
- public double hidErrSum = 0d;
- private final Random random;
- /**
- * Constructor.
- * <p>
- * <strong>Note:</strong> The capacity of each layer will be the parameter
- * plus 1. The additional unit is used for smoothness.
- * </p>
- *
- * @param inputSize
- * @param hiddenSize
- * @param outputSize
- * @param eta
- * @param momentum
- * @param epoch
- */
- public BP(int inputSize, int hiddenSize, int outputSize, double eta,
- double momentum) {
- input = new double[inputSize + 1];
- hidden = new double[hiddenSize + 1];
- output = new double[outputSize + 1];
- target = new double[outputSize + 1];
- hidDelta = new double[hiddenSize + 1];
- optDelta = new double[outputSize + 1];
- iptHidWeights = new double[inputSize + 1][hiddenSize + 1];
- hidOptWeights = new double[hiddenSize + 1][outputSize + 1];
- random = new Random(20140106);
- randomizeWeights(iptHidWeights);
- randomizeWeights(hidOptWeights);
- iptHidPrevUptWeights = new double[inputSize + 1][hiddenSize + 1];
- hidOptPrevUptWeights = new double[hiddenSize + 1][outputSize + 1];
- this.eta = eta;
- this.momentum = momentum;
- }
- private void randomizeWeights(double[][] matrix) {
- for (int i = 0, len = matrix.length; i != len; i++)
- for (int j = 0, len2 = matrix[i].length; j != len2; j++) {
- double real = random.nextDouble();
- matrix[i][j] = random.nextDouble() > 0.5 ? real : -real;
- }
- }
- /**
- * Constructor with default eta = 0.25 and momentum = 0.3.
- *
- * @param inputSize
- * @param hiddenSize
- * @param outputSize
- * @param epoch
- */
- public BP(int inputSize, int hiddenSize, int outputSize) {
- this(inputSize, hiddenSize, outputSize, 0.998, 0.001);
- }
- /**
- * Entry method. The train data should be a one-dim vector.
- *
- * @param trainData
- * @param target
- */
- public void train(double[] trainData, double[] target) {
- loadInput(trainData);
- loadTarget(target);
- forward();
- calculateDelta();
- adjustWeight();
- }
- /**
- * Test the BPNN.
- *
- * @param inData
- * @return
- */
- public double[] test(double[] inData) {
- if (inData.length != input.length - 1) {
- throw new IllegalArgumentException("Size Do Not Match.");
- }
- System.arraycopy(inData, 0, input, 1, inData.length);
- forward();
- return getNetworkOutput();
- }
- /**
- * Return the output layer.
- *
- * @return
- */
- private double[] getNetworkOutput() {
- int len = output.length;
- double[] temp = new double[len - 1];
- for (int i = 1; i != len; i++)
- temp[i - 1] = output[i];
- return temp;
- }
- /**
- * Load the target data.
- *
- * @param arg
- */
- private void loadTarget(double[] arg) {
- if (arg.length != target.length - 1) {
- throw new IllegalArgumentException("Size Do Not Match.");
- }
- System.arraycopy(arg, 0, target, 1, arg.length);
- }
- /**
- * Load the training data.
- *
- * @param inData
- */
- private void loadInput(double[] inData) {
- if (inData.length != input.length - 1) {
- throw new IllegalArgumentException("Size Do Not Match.");
- }
- System.arraycopy(inData, 0, input, 1, inData.length);
- }
- /**
- * Forward.
- *
- * @param layer0
- * @param layer1
- * @param weight
- */
- private void forward(double[] layer0, double[] layer1, double[][] weight) {
- // threshold unit.
- layer0[0] = 1.0;
- for (int j = 1, len = layer1.length; j != len; ++j) {
- double sum = 0;
- for (int i = 0, len2 = layer0.length; i != len2; ++i)
- sum += weight[i][j] * layer0[i];
- layer1[j] = sigmoid(sum);
- // layer1[j] = tansig(sum);
- }
- }
- /**
- * Forward.
- */
- private void forward() {
- forward(input, hidden, iptHidWeights);
- forward(hidden, output, hidOptWeights);
- }
- /**
- * Calculate output error.
- */
- private void outputErr() {
- double errSum = 0;
- for (int idx = 1, len = optDelta.length; idx != len; ++idx) {
- double o = output[idx];
- optDelta[idx] = o * (1d - o) * (target[idx] - o);
- errSum += Math.abs(optDelta[idx]);
- }
- optErrSum = errSum;
- }
- /**
- * Calculate hidden errors.
- */
- private void hiddenErr() {
- double errSum = 0;
- for (int j = 1, len = hidDelta.length; j != len; ++j) {
- double o = hidden[j];
- double sum = 0;
- for (int k = 1, len2 = optDelta.length; k != len2; ++k)
- sum += hidOptWeights[j][k] * optDelta[k];
- hidDelta[j] = o * (1d - o) * sum;
- errSum += Math.abs(hidDelta[j]);
- }
- hidErrSum = errSum;
- }
- /**
- * Calculate errors of all layers.
- */
- private void calculateDelta() {
- outputErr();
- hiddenErr();
- }
- /**
- * Adjust the weight matrix.
- *
- * @param delta
- * @param layer
- * @param weight
- * @param prevWeight
- */
- private void adjustWeight(double[] delta, double[] layer,
- double[][] weight, double[][] prevWeight) {
- layer[0] = 1;
- for (int i = 1, len = delta.length; i != len; ++i) {
- for (int j = 0, len2 = layer.length; j != len2; ++j) {
- double newVal = momentum * prevWeight[j][i] + eta * delta[i]
- * layer[j];
- weight[j][i] += newVal;
- prevWeight[j][i] = newVal;
- }
- }
- }
- /**
- * Adjust all weight matrices.
- */
- private void adjustWeight() {
- adjustWeight(optDelta, hidden, hidOptWeights, hidOptPrevUptWeights);
- adjustWeight(hidDelta, input, iptHidWeights, iptHidPrevUptWeights);
- }
- /**
- * Sigmoid.
- *
- * @param val
- * @return
- */
- private double sigmoid(double val) {
- return 1d / (1d + Math.exp(-val));
- }
- private double tansig(double val) {
- return 2d / (1d + Math.exp(-2 * val)) - 1;
- }
- }
上面就是BP神经网络的核心实现类了,看上去很简单吧。注意哦,它可是有线性学习能力的!工具有了,还是先把训练数据准备好吧,读数据库什么的太隐晦,先用个数组做简单点,即使要改以后也很容易。毕竟是做实验嘛,如果成功了,中了个二等奖,精度不够,要更进一步训练,我想也就不用俺多说了~~~
- package ghost.writer.data;
- public class Data {
- public static double[][] trainData_89feibo = {
- { 0.10, 0.13, 0.17, 0.28, 0.30, 0.32, 0.04 },
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 },
- { 0.06, 0.10, 0.11, 0.28, 0.30, 0.33, 0.12 },
- { 0.01, 0.04, 0.19, 0.22, 0.24, 0.25, 0.15 },
- { 0.15, 0.18, 0.23, 0.27, 0.32, 0.33, 0.04 },
- { 0.03, 0.04, 0.07, 0.17, 0.21, 0.27, 0.14 },
- { 0.08, 0.10, 0.12, 0.14, 0.18, 0.28, 0.14 },
- { 0.05, 0.14, 0.16, 0.21, 0.29, 0.30, 0.12 },
- { 0.08, 0.09, 0.19, 0.20, 0.25, 0.32, 0.16 },
- { 0.05, 0.07, 0.08, 0.20, 0.31, 0.33, 0.11 },
- { 0.09, 0.10, 0.13, 0.14, 0.21, 0.32, 0.02 },
- { 0.01, 0.08, 0.11, 0.19, 0.21, 0.24, 0.08 },
- { 0.05, 0.09, 0.13, 0.15, 0.17, 0.21, 0.13 },
- { 0.04, 0.09, 0.19, 0.22, 0.25, 0.29, 0.15 } };
- public static double[][] target_89feibo = {
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 },
- { 0.06, 0.10, 0.11, 0.28, 0.30, 0.33, 0.12 },
- { 0.01, 0.04, 0.19, 0.22, 0.24, 0.25, 0.15 },
- { 0.15, 0.18, 0.23, 0.27, 0.32, 0.33, 0.04 },
- { 0.03, 0.04, 0.07, 0.17, 0.21, 0.27, 0.14 },
- { 0.08, 0.10, 0.12, 0.14, 0.18, 0.28, 0.14 },
- { 0.05, 0.14, 0.16, 0.21, 0.29, 0.30, 0.12 },
- { 0.08, 0.09, 0.19, 0.20, 0.25, 0.32, 0.16 },
- { 0.05, 0.07, 0.08, 0.20, 0.31, 0.33, 0.11 },
- { 0.09, 0.10, 0.13, 0.14, 0.21, 0.32, 0.02 },
- { 0.01, 0.08, 0.11, 0.19, 0.21, 0.24, 0.08 },
- { 0.05, 0.09, 0.13, 0.15, 0.17, 0.21, 0.13 },
- { 0.04, 0.09, 0.19, 0.22, 0.25, 0.29, 0.15 },
- { 0.02, 0.11, 0.19, 0.30, 0.32, 0.33, 0.09 }};
- // 输入1期,输出1期,输入参数7个,减少输入的期数,长期无趋势短期也许会有
- public static double[][] trainData_5num = {
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 } };
- // 输入1期,输出1期,输出参数7个
- public static double[][] target_5num = {
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 },
- { 0.06, 0.10, 0.11, 0.28, 0.30, 0.33, 0.12 } };
- // 输入1期,输出1期,输入参数7个
- public static double[][] trainData = {
- { 0.05, 0.14, 0.24, 0.25, 0.26, 0.32, 0.01 },
- { 0.10, 0.12, 0.18, 0.22, 0.28, 0.29, 0.07 },
- { 0.04, 0.05, 0.11, 0.21, 0.27, 0.28, 0.10 },
- { 0.05, 0.07, 0.12, 0.16, 0.28, 0.32, 0.04 },
- { 0.06, 0.08, 0.14, 0.15, 0.24, 0.25, 0.06 },
- { 0.01, 0.16, 0.18, 0.22, 0.28, 0.30, 0.12 },
- { 0.22, 0.23, 0.26, 0.27, 0.28, 0.33, 0.09 },
- { 0.06, 0.10, 0.16, 0.20, 0.27, 0.32, 0.08 },
- { 0.01, 0.13, 0.14, 0.25, 0.31, 0.32, 0.12 },
- { 0.09, 0.10, 0.13, 0.17, 0.22, 0.30, 0.13 },
- { 0.02, 0.09, 0.15, 0.22, 0.26, 0.32, 0.01 },
- { 0.03, 0.08, 0.17, 0.21, 0.25, 0.32, 0.15 },
- { 0.01, 0.04, 0.09, 0.13, 0.16, 0.23, 0.02 },
- { 0.01, 0.09, 0.11, 0.17, 0.32, 0.33, 0.12 },
- { 0.03, 0.12, 0.17, 0.24, 0.27, 0.29, 0.09 },
- { 0.06, 0.14, 0.17, 0.22, 0.28, 0.29, 0.02 },
- { 0.05, 0.06, 0.13, 0.19, 0.22, 0.28, 0.09 },
- { 0.02, 0.04, 0.05, 0.17, 0.19, 0.20, 0.08 },
- { 0.05, 0.06, 0.07, 0.11, 0.13, 0.18, 0.15 },
- { 0.02, 0.05, 0.06, 0.12, 0.14, 0.28, 0.05 },
- { 0.04, 0.06, 0.12, 0.30, 0.31, 0.32, 0.09 },
- { 0.02, 0.08, 0.13, 0.28, 0.29, 0.30, 0.05 },
- { 0.01, 0.02, 0.05, 0.16, 0.20, 0.26, 0.06 },
- { 0.01, 0.07, 0.08, 0.12, 0.16, 0.21, 0.01 },
- { 0.01, 0.06, 0.17, 0.19, 0.26, 0.31, 0.11 },
- { 0.02, 0.04, 0.07, 0.09, 0.15, 0.20, 0.07 },
- { 0.03, 0.06, 0.15, 0.18, 0.30, 0.32, 0.05 },
- { 0.04, 0.05, 0.13, 0.23, 0.27, 0.30, 0.09 },
- { 0.16, 0.17, 0.18, 0.24, 0.25, 0.30, 0.08 },
- { 0.04, 0.11, 0.14, 0.15, 0.22, 0.31, 0.11 },
- { 0.01, 0.02, 0.04, 0.12, 0.21, 0.24, 0.12 },
- { 0.07, 0.08, 0.14, 0.25, 0.26, 0.28, 0.13 },
- { 0.06, 0.07, 0.10, 0.19, 0.23, 0.29, 0.12 },
- { 0.07, 0.14, 0.18, 0.25, 0.26, 0.29, 0.06 },
- { 0.03, 0.13, 0.14, 0.15, 0.21, 0.33, 0.03 },
- { 0.04, 0.21, 0.25, 0.29, 0.30, 0.33, 0.03 },
- { 0.05, 0.06, 0.13, 0.17, 0.19, 0.28, 0.01 },
- { 0.06, 0.15, 0.20, 0.22, 0.26, 0.33, 0.09 },
- { 0.01, 0.14, 0.15, 0.17, 0.26, 0.30, 0.02 },
- { 0.04, 0.05, 0.09, 0.27, 0.29, 0.31, 0.13 },
- { 0.02, 0.15, 0.18, 0.27, 0.28, 0.32, 0.14 },
- { 0.09, 0.10, 0.12, 0.14, 0.15, 0.19, 0.11 },
- { 0.01, 0.02, 0.14, 0.15, 0.24, 0.29, 0.06 },
- { 0.02, 0.04, 0.10, 0.12, 0.17, 0.30, 0.10 },
- { 0.02, 0.10, 0.12, 0.17, 0.23, 0.24, 0.05 },
- { 0.01, 0.08, 0.12, 0.13, 0.15, 0.33, 0.03 },
- { 0.03, 0.06, 0.14, 0.15, 0.17, 0.25, 0.16 },
- { 0.03, 0.05, 0.11, 0.18, 0.26, 0.28, 0.06 },
- { 0.06, 0.07, 0.08, 0.14, 0.23, 0.31, 0.12 },
- { 0.03, 0.16, 0.19, 0.20, 0.24, 0.26, 0.06 },
- { 0.01, 0.08, 0.11, 0.17, 0.27, 0.30, 0.12 },
- { 0.10, 0.13, 0.17, 0.28, 0.30, 0.32, 0.04 },
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 } };
- // 输入1期,输出1期,输出参数7个
- public static double[][] target = {
- { 0.10, 0.12, 0.18, 0.22, 0.28, 0.29, 0.07 },
- { 0.04, 0.05, 0.11, 0.21, 0.27, 0.28, 0.10 },
- { 0.05, 0.07, 0.12, 0.16, 0.28, 0.32, 0.04 },
- { 0.06, 0.08, 0.14, 0.15, 0.24, 0.25, 0.06 },
- { 0.01, 0.16, 0.18, 0.22, 0.28, 0.30, 0.12 },
- { 0.22, 0.23, 0.26, 0.27, 0.28, 0.33, 0.09 },
- { 0.06, 0.10, 0.16, 0.20, 0.27, 0.32, 0.08 },
- { 0.01, 0.13, 0.14, 0.25, 0.31, 0.32, 0.12 },
- { 0.09, 0.10, 0.13, 0.17, 0.22, 0.30, 0.13 },
- { 0.02, 0.09, 0.15, 0.22, 0.26, 0.32, 0.01 },
- { 0.03, 0.08, 0.17, 0.21, 0.25, 0.32, 0.15 },
- { 0.01, 0.04, 0.09, 0.13, 0.16, 0.23, 0.02 },
- { 0.01, 0.09, 0.11, 0.17, 0.32, 0.33, 0.12 },
- { 0.03, 0.12, 0.17, 0.24, 0.27, 0.29, 0.09 },
- { 0.06, 0.14, 0.17, 0.22, 0.28, 0.29, 0.02 },
- { 0.05, 0.06, 0.13, 0.19, 0.22, 0.28, 0.09 },
- { 0.02, 0.04, 0.05, 0.17, 0.19, 0.20, 0.08 },
- { 0.05, 0.06, 0.07, 0.11, 0.13, 0.18, 0.15 },
- { 0.02, 0.05, 0.06, 0.12, 0.14, 0.28, 0.05 },
- { 0.04, 0.06, 0.12, 0.30, 0.31, 0.32, 0.09 },
- { 0.02, 0.08, 0.13, 0.28, 0.29, 0.30, 0.05 },
- { 0.01, 0.02, 0.05, 0.16, 0.20, 0.26, 0.06 },
- { 0.01, 0.07, 0.08, 0.12, 0.16, 0.21, 0.01 },
- { 0.01, 0.06, 0.17, 0.19, 0.26, 0.31, 0.11 },
- { 0.02, 0.04, 0.07, 0.09, 0.15, 0.20, 0.07 },
- { 0.03, 0.06, 0.15, 0.18, 0.30, 0.32, 0.05 },
- { 0.04, 0.05, 0.13, 0.23, 0.27, 0.30, 0.09 },
- { 0.16, 0.17, 0.18, 0.24, 0.25, 0.30, 0.08 },
- { 0.04, 0.11, 0.14, 0.15, 0.22, 0.31, 0.11 },
- { 0.01, 0.02, 0.04, 0.12, 0.21, 0.24, 0.12 },
- { 0.07, 0.08, 0.14, 0.25, 0.26, 0.28, 0.13 },
- { 0.06, 0.07, 0.10, 0.19, 0.23, 0.29, 0.12 },
- { 0.07, 0.14, 0.18, 0.25, 0.26, 0.29, 0.06 },
- { 0.03, 0.13, 0.14, 0.15, 0.21, 0.33, 0.03 },
- { 0.04, 0.21, 0.25, 0.29, 0.30, 0.33, 0.03 },
- { 0.05, 0.06, 0.13, 0.17, 0.19, 0.28, 0.01 },
- { 0.06, 0.15, 0.20, 0.22, 0.26, 0.33, 0.09 },
- { 0.01, 0.14, 0.15, 0.17, 0.26, 0.30, 0.02 },
- { 0.04, 0.05, 0.09, 0.27, 0.29, 0.31, 0.13 },
- { 0.02, 0.15, 0.18, 0.27, 0.28, 0.32, 0.14 },
- { 0.09, 0.10, 0.12, 0.14, 0.15, 0.19, 0.11 },
- { 0.01, 0.02, 0.14, 0.15, 0.24, 0.29, 0.06 },
- { 0.02, 0.04, 0.10, 0.12, 0.17, 0.30, 0.10 },
- { 0.02, 0.10, 0.12, 0.17, 0.23, 0.24, 0.05 },
- { 0.01, 0.08, 0.12, 0.13, 0.15, 0.33, 0.03 },
- { 0.03, 0.06, 0.14, 0.15, 0.17, 0.25, 0.16 },
- { 0.03, 0.05, 0.11, 0.18, 0.26, 0.28, 0.06 },
- { 0.06, 0.07, 0.08, 0.14, 0.23, 0.31, 0.12 },
- { 0.03, 0.16, 0.19, 0.20, 0.24, 0.26, 0.06 },
- { 0.01, 0.08, 0.11, 0.17, 0.27, 0.30, 0.12 },
- { 0.10, 0.13, 0.17, 0.28, 0.30, 0.32, 0.04 },
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 } };
- // 连续2期作为一组输入,输入是14个变量
- public static double[][] trainData_2 = {
- { 0.05, 0.14, 0.24, 0.25, 0.26, 0.32, 0.01, 0.10, 0.12, 0.18, 0.22,
- 0.28, 0.29, 0.07 },
- { 0.10, 0.12, 0.18, 0.22, 0.28, 0.29, 0.07, 0.04, 0.05, 0.11, 0.21,
- 0.27, 0.28, 0.10 },
- { 0.04, 0.05, 0.11, 0.21, 0.27, 0.28, 0.10, 0.05, 0.07, 0.12, 0.16,
- 0.28, 0.32, 0.04 },
- { 0.05, 0.07, 0.12, 0.16, 0.28, 0.32, 0.04, 0.06, 0.08, 0.14, 0.15,
- 0.24, 0.25, 0.06 },
- { 0.06, 0.08, 0.14, 0.15, 0.24, 0.25, 0.06, 0.01, 0.16, 0.18, 0.22,
- 0.28, 0.30, 0.12 },
- { 0.01, 0.16, 0.18, 0.22, 0.28, 0.30, 0.12, 0.22, 0.23, 0.26, 0.27,
- 0.28, 0.33, 0.09 },
- { 0.22, 0.23, 0.26, 0.27, 0.28, 0.33, 0.09, 0.06, 0.10, 0.16, 0.20,
- 0.27, 0.32, 0.08 },
- { 0.06, 0.10, 0.16, 0.20, 0.27, 0.32, 0.08, 0.01, 0.13, 0.14, 0.25,
- 0.31, 0.32, 0.12 },
- { 0.01, 0.13, 0.14, 0.25, 0.31, 0.32, 0.12, 0.09, 0.10, 0.13, 0.17,
- 0.22, 0.30, 0.13 },
- { 0.09, 0.10, 0.13, 0.17, 0.22, 0.30, 0.13, 0.02, 0.09, 0.15, 0.22,
- 0.26, 0.32, 0.01 },
- { 0.02, 0.09, 0.15, 0.22, 0.26, 0.32, 0.01, 0.03, 0.08, 0.17, 0.21,
- 0.25, 0.32, 0.15 },
- { 0.03, 0.08, 0.17, 0.21, 0.25, 0.32, 0.15, 0.01, 0.04, 0.09, 0.13,
- 0.16, 0.23, 0.02 },
- { 0.01, 0.04, 0.09, 0.13, 0.16, 0.23, 0.02, 0.01, 0.09, 0.11, 0.17,
- 0.32, 0.33, 0.12 },
- { 0.01, 0.09, 0.11, 0.17, 0.32, 0.33, 0.12, 0.03, 0.12, 0.17, 0.24,
- 0.27, 0.29, 0.09 },
- { 0.03, 0.12, 0.17, 0.24, 0.27, 0.29, 0.09, 0.06, 0.14, 0.17, 0.22,
- 0.28, 0.29, 0.02 },
- { 0.06, 0.14, 0.17, 0.22, 0.28, 0.29, 0.02, 0.05, 0.06, 0.13, 0.19,
- 0.22, 0.28, 0.09 },
- { 0.05, 0.06, 0.13, 0.19, 0.22, 0.28, 0.09, 0.02, 0.04, 0.05, 0.17,
- 0.19, 0.20, 0.08 },
- { 0.02, 0.04, 0.05, 0.17, 0.19, 0.20, 0.08, 0.05, 0.06, 0.07, 0.11,
- 0.13, 0.18, 0.15 },
- { 0.05, 0.06, 0.07, 0.11, 0.13, 0.18, 0.15, 0.02, 0.05, 0.06, 0.12,
- 0.14, 0.28, 0.05 },
- { 0.02, 0.05, 0.06, 0.12, 0.14, 0.28, 0.05, 0.04, 0.06, 0.12, 0.30,
- 0.31, 0.32, 0.09 },
- { 0.04, 0.06, 0.12, 0.30, 0.31, 0.32, 0.09, 0.02, 0.08, 0.13, 0.28,
- 0.29, 0.30, 0.05 },
- { 0.02, 0.08, 0.13, 0.28, 0.29, 0.30, 0.05, 0.01, 0.02, 0.05, 0.16,
- 0.20, 0.26, 0.06 },
- { 0.01, 0.02, 0.05, 0.16, 0.20, 0.26, 0.06, 0.01, 0.07, 0.08, 0.12,
- 0.16, 0.21, 0.01 },
- { 0.01, 0.07, 0.08, 0.12, 0.16, 0.21, 0.01, 0.01, 0.06, 0.17, 0.19,
- 0.26, 0.31, 0.11 },
- { 0.01, 0.06, 0.17, 0.19, 0.26, 0.31, 0.11, 0.02, 0.04, 0.07, 0.09,
- 0.15, 0.20, 0.07 },
- { 0.02, 0.04, 0.07, 0.09, 0.15, 0.20, 0.07, 0.03, 0.06, 0.15, 0.18,
- 0.30, 0.32, 0.05 },
- { 0.03, 0.06, 0.15, 0.18, 0.30, 0.32, 0.05, 0.04, 0.05, 0.13, 0.23,
- 0.27, 0.30, 0.09 },
- { 0.04, 0.05, 0.13, 0.23, 0.27, 0.30, 0.09, 0.16, 0.17, 0.18, 0.24,
- 0.25, 0.30, 0.08 },
- { 0.16, 0.17, 0.18, 0.24, 0.25, 0.30, 0.08, 0.04, 0.11, 0.14, 0.15,
- 0.22, 0.31, 0.11 },
- { 0.04, 0.11, 0.14, 0.15, 0.22, 0.31, 0.11, 0.01, 0.02, 0.04, 0.12,
- 0.21, 0.24, 0.12 },
- { 0.01, 0.02, 0.04, 0.12, 0.21, 0.24, 0.12, 0.07, 0.08, 0.14, 0.25,
- 0.26, 0.28, 0.13 },
- { 0.07, 0.08, 0.14, 0.25, 0.26, 0.28, 0.13, 0.06, 0.07, 0.10, 0.19,
- 0.23, 0.29, 0.12 },
- { 0.06, 0.07, 0.10, 0.19, 0.23, 0.29, 0.12, 0.07, 0.14, 0.18, 0.25,
- 0.26, 0.29, 0.06 },
- { 0.07, 0.14, 0.18, 0.25, 0.26, 0.29, 0.06, 0.03, 0.13, 0.14, 0.15,
- 0.21, 0.33, 0.03 },
- { 0.03, 0.13, 0.14, 0.15, 0.21, 0.33, 0.03, 0.04, 0.21, 0.25, 0.29,
- 0.30, 0.33, 0.03 },
- { 0.04, 0.21, 0.25, 0.29, 0.30, 0.33, 0.03, 0.05, 0.06, 0.13, 0.17,
- 0.19, 0.28, 0.01 },
- { 0.05, 0.06, 0.13, 0.17, 0.19, 0.28, 0.01, 0.06, 0.15, 0.20, 0.22,
- 0.26, 0.33, 0.09 },
- { 0.06, 0.15, 0.20, 0.22, 0.26, 0.33, 0.09, 0.01, 0.14, 0.15, 0.17,
- 0.26, 0.30, 0.02 },
- { 0.01, 0.14, 0.15, 0.17, 0.26, 0.30, 0.02, 0.04, 0.05, 0.09, 0.27,
- 0.29, 0.31, 0.13 },
- { 0.04, 0.05, 0.09, 0.27, 0.29, 0.31, 0.13, 0.02, 0.15, 0.18, 0.27,
- 0.28, 0.32, 0.14 },
- { 0.02, 0.15, 0.18, 0.27, 0.28, 0.32, 0.14, 0.09, 0.10, 0.12, 0.14,
- 0.15, 0.19, 0.11 },
- { 0.09, 0.10, 0.12, 0.14, 0.15, 0.19, 0.11, 0.01, 0.02, 0.14, 0.15,
- 0.24, 0.29, 0.06 },
- { 0.01, 0.02, 0.14, 0.15, 0.24, 0.29, 0.06, 0.02, 0.04, 0.10, 0.12,
- 0.17, 0.30, 0.10 },
- { 0.02, 0.04, 0.10, 0.12, 0.17, 0.30, 0.10, 0.02, 0.10, 0.12, 0.17,
- 0.23, 0.24, 0.05 },
- { 0.02, 0.10, 0.12, 0.17, 0.23, 0.24, 0.05, 0.01, 0.08, 0.12, 0.13,
- 0.15, 0.33, 0.03 },
- { 0.01, 0.08, 0.12, 0.13, 0.15, 0.33, 0.03, 0.03, 0.06, 0.14, 0.15,
- 0.17, 0.25, 0.16 },
- { 0.03, 0.06, 0.14, 0.15, 0.17, 0.25, 0.16, 0.03, 0.05, 0.11, 0.18,
- 0.26, 0.28, 0.06 },
- { 0.03, 0.05, 0.11, 0.18, 0.26, 0.28, 0.06, 0.06, 0.07, 0.08, 0.14,
- 0.23, 0.31, 0.12 },
- { 0.06, 0.07, 0.08, 0.14, 0.23, 0.31, 0.12, 0.03, 0.16, 0.19, 0.20,
- 0.24, 0.26, 0.06 },
- { 0.03, 0.16, 0.19, 0.20, 0.24, 0.26, 0.06, 0.01, 0.08, 0.11, 0.17,
- 0.27, 0.30, 0.12 },
- { 0.01, 0.08, 0.11, 0.17, 0.27, 0.30, 0.12, 0.10, 0.13, 0.17, 0.28,
- 0.30, 0.32, 0.04 },
- { 0.10, 0.13, 0.17, 0.28, 0.30, 0.32, 0.04, 0.10, 0.13, 0.14, 0.16,
- 0.21, 0.32, 0.14 },
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14, 0.03, 0.07, 0.13, 0.18,
- 0.22, 0.25, 0.03 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03, 0.08, 0.12, 0.15, 0.19,
- 0.28, 0.29, 0.02 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02, 0.06, 0.07, 0.14, 0.21,
- 0.22, 0.24, 0.13 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13, 0.03, 0.12, 0.13, 0.22,
- 0.30, 0.33, 0.14 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14, 0.03, 0.04, 0.08, 0.14,
- 0.21, 0.28, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14, 0.08, 0.18, 0.19, 0.22,
- 0.27, 0.32, 0.06 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06, 0.03, 0.12, 0.25, 0.26,
- 0.28, 0.29, 0.16 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16, 0.13, 0.16, 0.19, 0.23,
- 0.26, 0.28, 0.05 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05, 0.08, 0.11, 0.17, 0.21,
- 0.23, 0.24, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05, 0.03, 0.10, 0.18, 0.24,
- 0.27, 0.29, 0.09 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09, 0.05, 0.07, 0.10, 0.13,
- 0.19, 0.20, 0.15 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15, 0.05, 0.06, 0.07, 0.12,
- 0.13, 0.18, 0.12 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12, 0.01, 0.06, 0.07, 0.19,
- 0.22, 0.27, 0.02 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02, 0.10, 0.15, 0.18, 0.20,
- 0.23, 0.31, 0.12 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12, 0.01, 0.09, 0.13, 0.22,
- 0.25, 0.32, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12, 0.07, 0.18, 0.19, 0.23,
- 0.29, 0.30, 0.02 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02, 0.01, 0.03, 0.16, 0.17,
- 0.20, 0.32, 0.07 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07, 0.01, 0.04, 0.09, 0.15,
- 0.22, 0.30, 0.06 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06, 0.02, 0.07, 0.13, 0.20,
- 0.25, 0.27, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06, 0.07, 0.16, 0.17, 0.18,
- 0.30, 0.33, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06, 0.02, 0.03, 0.09, 0.10,
- 0.28, 0.30, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06, 0.05, 0.12, 0.21, 0.23,
- 0.26, 0.28, 0.09 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09, 0.02, 0.08, 0.11, 0.14,
- 0.19, 0.33, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09, 0.02, 0.09, 0.13, 0.17,
- 0.20, 0.28, 0.11 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11, 0.03, 0.06, 0.08, 0.14,
- 0.19, 0.32, 0.03 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03, 0.04, 0.06, 0.09, 0.25,
- 0.30, 0.33, 0.14 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14, 0.14, 0.23, 0.24, 0.26,
- 0.29, 0.30, 0.03 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03, 0.09, 0.14, 0.23, 0.24,
- 0.26, 0.29, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03, 0.03, 0.05, 0.17, 0.18,
- 0.26, 0.27, 0.15 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15, 0.07, 0.13, 0.17, 0.19,
- 0.22, 0.26, 0.13 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13, 0.10, 0.11, 0.12, 0.23,
- 0.28, 0.32, 0.16 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16, 0.01, 0.04, 0.10, 0.13,
- 0.21, 0.31, 0.13 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13, 0.04, 0.13, 0.14, 0.20,
- 0.22, 0.30, 0.06 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06, 0.05, 0.06, 0.12, 0.14,
- 0.19, 0.23, 0.09 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09, 0.05, 0.07, 0.09, 0.11,
- 0.20, 0.21, 0.03 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03, 0.02, 0.08, 0.12, 0.14,
- 0.16, 0.32, 0.16 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16, 0.02, 0.04, 0.11, 0.13,
- 0.16, 0.26, 0.11 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11, 0.02, 0.13, 0.19, 0.23,
- 0.24, 0.28, 0.05 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05, 0.09, 0.15, 0.20, 0.21,
- 0.22, 0.24, 0.14 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14, 0.04, 0.08, 0.12, 0.19,
- 0.21, 0.25, 0.13 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13, 0.02, 0.05, 0.11, 0.23,
- 0.24, 0.29, 0.08 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08, 0.04, 0.14, 0.24, 0.25,
- 0.28, 0.31, 0.10 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10, 0.07, 0.11, 0.15, 0.21,
- 0.26, 0.31, 0.06 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06, 0.01, 0.02, 0.08, 0.26,
- 0.29, 0.31, 0.14 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14, 0.02, 0.04, 0.14, 0.18,
- 0.20, 0.22, 0.07 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07, 0.01, 0.06, 0.15, 0.19,
- 0.28, 0.29, 0.10 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10, 0.01, 0.02, 0.22, 0.28,
- 0.29, 0.30, 0.15 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15, 0.05, 0.14, 0.17, 0.22,
- 0.23, 0.25, 0.07 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07, 0.07, 0.15, 0.18, 0.19,
- 0.20, 0.26, 0.14 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14, 0.05, 0.11, 0.20, 0.21,
- 0.26, 0.31, 0.03 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03, 0.04, 0.08, 0.11, 0.14,
- 0.16, 0.20, 0.11 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11, 0.05, 0.07, 0.09, 0.23,
- 0.27, 0.32, 0.01 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01, 0.02, 0.04, 0.05, 0.06,
- 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03, 0.02, 0.04, 0.09, 0.13,
- 0.18, 0.20, 0.07 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07, 0.01, 0.02, 0.04, 0.15,
- 0.17, 0.28, 0.11 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11, 0.01, 0.11, 0.23, 0.27,
- 0.31, 0.32, 0.09 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09, 0.09, 0.11, 0.23, 0.30,
- 0.31, 0.32, 0.06 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06, 0.07, 0.09, 0.11, 0.17,
- 0.28, 0.31, 0.11 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11, 0.16, 0.21, 0.22, 0.28,
- 0.31, 0.32, 0.05 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05, 0.09, 0.23, 0.24, 0.27,
- 0.29, 0.32, 0.08 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08, 0.15, 0.17, 0.18, 0.21,
- 0.29, 0.32, 0.13 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13, 0.01, 0.02, 0.03, 0.06,
- 0.08, 0.33, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13, 0.01, 0.06, 0.12, 0.13,
- 0.22, 0.31, 0.07 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07, 0.04, 0.07, 0.11, 0.17,
- 0.24, 0.33, 0.09 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09, 0.04, 0.06, 0.17, 0.21,
- 0.23, 0.33, 0.07 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07, 0.03, 0.12, 0.16, 0.17,
- 0.18, 0.27, 0.08 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08, 0.12, 0.15, 0.21, 0.26,
- 0.32, 0.33, 0.07 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07, 0.04, 0.17, 0.19, 0.23,
- 0.24, 0.27, 0.10 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10, 0.04, 0.15, 0.16, 0.24,
- 0.27, 0.28, 0.03 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03, 0.07, 0.08, 0.11, 0.13,
- 0.21, 0.27, 0.08 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08, 0.01, 0.05, 0.12, 0.13,
- 0.21, 0.22, 0.10 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10, 0.03, 0.04, 0.05, 0.25,
- 0.30, 0.31, 0.04 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04, 0.11, 0.12, 0.14, 0.20,
- 0.22, 0.29, 0.14 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14, 0.12, 0.18, 0.21, 0.22,
- 0.27, 0.32, 0.11 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11, 0.05, 0.07, 0.12, 0.19,
- 0.27, 0.31, 0.02 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02, 0.06, 0.10, 0.13, 0.16,
- 0.23, 0.24, 0.15 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15, 0.08, 0.20, 0.25, 0.30,
- 0.32, 0.33, 0.01 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01, 0.02, 0.15, 0.16, 0.17,
- 0.19, 0.30, 0.08 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08, 0.06, 0.11, 0.12, 0.14,
- 0.17, 0.22, 0.01 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01, 0.09, 0.18, 0.25, 0.26,
- 0.30, 0.32, 0.11 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11, 0.01, 0.15, 0.16, 0.25,
- 0.26, 0.29, 0.10 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10, 0.03, 0.09, 0.10, 0.19,
- 0.28, 0.33, 0.09 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09, 0.04, 0.06, 0.14, 0.16,
- 0.18, 0.29, 0.05 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05, 0.08, 0.11, 0.13, 0.18,
- 0.28, 0.33, 0.10 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10, 0.07, 0.11, 0.14, 0.19,
- 0.24, 0.29, 0.05 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05, 0.03, 0.09, 0.15, 0.20,
- 0.27, 0.29, 0.01 } };
- // 连续2期作为一组输入的解,输出还是7个
- public static double[][] target_2 = {
- { 0.04, 0.05, 0.11, 0.21, 0.27, 0.28, 0.10 },
- { 0.05, 0.07, 0.12, 0.16, 0.28, 0.32, 0.04 },
- { 0.06, 0.08, 0.14, 0.15, 0.24, 0.25, 0.06 },
- { 0.01, 0.16, 0.18, 0.22, 0.28, 0.30, 0.12 },
- { 0.22, 0.23, 0.26, 0.27, 0.28, 0.33, 0.09 },
- { 0.06, 0.10, 0.16, 0.20, 0.27, 0.32, 0.08 },
- { 0.01, 0.13, 0.14, 0.25, 0.31, 0.32, 0.12 },
- { 0.09, 0.10, 0.13, 0.17, 0.22, 0.30, 0.13 },
- { 0.02, 0.09, 0.15, 0.22, 0.26, 0.32, 0.01 },
- { 0.03, 0.08, 0.17, 0.21, 0.25, 0.32, 0.15 },
- { 0.01, 0.04, 0.09, 0.13, 0.16, 0.23, 0.02 },
- { 0.01, 0.09, 0.11, 0.17, 0.32, 0.33, 0.12 },
- { 0.03, 0.12, 0.17, 0.24, 0.27, 0.29, 0.09 },
- { 0.06, 0.14, 0.17, 0.22, 0.28, 0.29, 0.02 },
- { 0.05, 0.06, 0.13, 0.19, 0.22, 0.28, 0.09 },
- { 0.02, 0.04, 0.05, 0.17, 0.19, 0.20, 0.08 },
- { 0.05, 0.06, 0.07, 0.11, 0.13, 0.18, 0.15 },
- { 0.02, 0.05, 0.06, 0.12, 0.14, 0.28, 0.05 },
- { 0.04, 0.06, 0.12, 0.30, 0.31, 0.32, 0.09 },
- { 0.02, 0.08, 0.13, 0.28, 0.29, 0.30, 0.05 },
- { 0.01, 0.02, 0.05, 0.16, 0.20, 0.26, 0.06 },
- { 0.01, 0.07, 0.08, 0.12, 0.16, 0.21, 0.01 },
- { 0.01, 0.06, 0.17, 0.19, 0.26, 0.31, 0.11 },
- { 0.02, 0.04, 0.07, 0.09, 0.15, 0.20, 0.07 },
- { 0.03, 0.06, 0.15, 0.18, 0.30, 0.32, 0.05 },
- { 0.04, 0.05, 0.13, 0.23, 0.27, 0.30, 0.09 },
- { 0.16, 0.17, 0.18, 0.24, 0.25, 0.30, 0.08 },
- { 0.04, 0.11, 0.14, 0.15, 0.22, 0.31, 0.11 },
- { 0.01, 0.02, 0.04, 0.12, 0.21, 0.24, 0.12 },
- { 0.07, 0.08, 0.14, 0.25, 0.26, 0.28, 0.13 },
- { 0.06, 0.07, 0.10, 0.19, 0.23, 0.29, 0.12 },
- { 0.07, 0.14, 0.18, 0.25, 0.26, 0.29, 0.06 },
- { 0.03, 0.13, 0.14, 0.15, 0.21, 0.33, 0.03 },
- { 0.04, 0.21, 0.25, 0.29, 0.30, 0.33, 0.03 },
- { 0.05, 0.06, 0.13, 0.17, 0.19, 0.28, 0.01 },
- { 0.06, 0.15, 0.20, 0.22, 0.26, 0.33, 0.09 },
- { 0.01, 0.14, 0.15, 0.17, 0.26, 0.30, 0.02 },
- { 0.04, 0.05, 0.09, 0.27, 0.29, 0.31, 0.13 },
- { 0.02, 0.15, 0.18, 0.27, 0.28, 0.32, 0.14 },
- { 0.09, 0.10, 0.12, 0.14, 0.15, 0.19, 0.11 },
- { 0.01, 0.02, 0.14, 0.15, 0.24, 0.29, 0.06 },
- { 0.02, 0.04, 0.10, 0.12, 0.17, 0.30, 0.10 },
- { 0.02, 0.10, 0.12, 0.17, 0.23, 0.24, 0.05 },
- { 0.01, 0.08, 0.12, 0.13, 0.15, 0.33, 0.03 },
- { 0.03, 0.06, 0.14, 0.15, 0.17, 0.25, 0.16 },
- { 0.03, 0.05, 0.11, 0.18, 0.26, 0.28, 0.06 },
- { 0.06, 0.07, 0.08, 0.14, 0.23, 0.31, 0.12 },
- { 0.03, 0.16, 0.19, 0.20, 0.24, 0.26, 0.06 },
- { 0.01, 0.08, 0.11, 0.17, 0.27, 0.30, 0.12 },
- { 0.10, 0.13, 0.17, 0.28, 0.30, 0.32, 0.04 },
- { 0.10, 0.13, 0.14, 0.16, 0.21, 0.32, 0.14 },
- { 0.03, 0.07, 0.13, 0.18, 0.22, 0.25, 0.03 },
- { 0.08, 0.12, 0.15, 0.19, 0.28, 0.29, 0.02 },
- { 0.06, 0.07, 0.14, 0.21, 0.22, 0.24, 0.13 },
- { 0.03, 0.12, 0.13, 0.22, 0.30, 0.33, 0.14 },
- { 0.03, 0.04, 0.08, 0.14, 0.21, 0.28, 0.14 },
- { 0.08, 0.18, 0.19, 0.22, 0.27, 0.32, 0.06 },
- { 0.03, 0.12, 0.25, 0.26, 0.28, 0.29, 0.16 },
- { 0.13, 0.16, 0.19, 0.23, 0.26, 0.28, 0.05 },
- { 0.08, 0.11, 0.17, 0.21, 0.23, 0.24, 0.05 },
- { 0.03, 0.10, 0.18, 0.24, 0.27, 0.29, 0.09 },
- { 0.05, 0.07, 0.10, 0.13, 0.19, 0.20, 0.15 },
- { 0.05, 0.06, 0.07, 0.12, 0.13, 0.18, 0.12 },
- { 0.01, 0.06, 0.07, 0.19, 0.22, 0.27, 0.02 },
- { 0.10, 0.15, 0.18, 0.20, 0.23, 0.31, 0.12 },
- { 0.01, 0.09, 0.13, 0.22, 0.25, 0.32, 0.12 },
- { 0.07, 0.18, 0.19, 0.23, 0.29, 0.30, 0.02 },
- { 0.01, 0.03, 0.16, 0.17, 0.20, 0.32, 0.07 },
- { 0.01, 0.04, 0.09, 0.15, 0.22, 0.30, 0.06 },
- { 0.02, 0.07, 0.13, 0.20, 0.25, 0.27, 0.06 },
- { 0.07, 0.16, 0.17, 0.18, 0.30, 0.33, 0.06 },
- { 0.02, 0.03, 0.09, 0.10, 0.28, 0.30, 0.06 },
- { 0.05, 0.12, 0.21, 0.23, 0.26, 0.28, 0.09 },
- { 0.02, 0.08, 0.11, 0.14, 0.19, 0.33, 0.09 },
- { 0.02, 0.09, 0.13, 0.17, 0.20, 0.28, 0.11 },
- { 0.03, 0.06, 0.08, 0.14, 0.19, 0.32, 0.03 },
- { 0.04, 0.06, 0.09, 0.25, 0.30, 0.33, 0.14 },
- { 0.14, 0.23, 0.24, 0.26, 0.29, 0.30, 0.03 },
- { 0.09, 0.14, 0.23, 0.24, 0.26, 0.29, 0.03 },
- { 0.03, 0.05, 0.17, 0.18, 0.26, 0.27, 0.15 },
- { 0.07, 0.13, 0.17, 0.19, 0.22, 0.26, 0.13 },
- { 0.10, 0.11, 0.12, 0.23, 0.28, 0.32, 0.16 },
- { 0.01, 0.04, 0.10, 0.13, 0.21, 0.31, 0.13 },
- { 0.04, 0.13, 0.14, 0.20, 0.22, 0.30, 0.06 },
- { 0.05, 0.06, 0.12, 0.14, 0.19, 0.23, 0.09 },
- { 0.05, 0.07, 0.09, 0.11, 0.20, 0.21, 0.03 },
- { 0.02, 0.08, 0.12, 0.14, 0.16, 0.32, 0.16 },
- { 0.02, 0.04, 0.11, 0.13, 0.16, 0.26, 0.11 },
- { 0.02, 0.13, 0.19, 0.23, 0.24, 0.28, 0.05 },
- { 0.09, 0.15, 0.20, 0.21, 0.22, 0.24, 0.14 },
- { 0.04, 0.08, 0.12, 0.19, 0.21, 0.25, 0.13 },
- { 0.02, 0.05, 0.11, 0.23, 0.24, 0.29, 0.08 },
- { 0.04, 0.14, 0.24, 0.25, 0.28, 0.31, 0.10 },
- { 0.07, 0.11, 0.15, 0.21, 0.26, 0.31, 0.06 },
- { 0.01, 0.02, 0.08, 0.26, 0.29, 0.31, 0.14 },
- { 0.02, 0.04, 0.14, 0.18, 0.20, 0.22, 0.07 },
- { 0.01, 0.06, 0.15, 0.19, 0.28, 0.29, 0.10 },
- { 0.01, 0.02, 0.22, 0.28, 0.29, 0.30, 0.15 },
- { 0.05, 0.14, 0.17, 0.22, 0.23, 0.25, 0.07 },
- { 0.07, 0.15, 0.18, 0.19, 0.20, 0.26, 0.14 },
- { 0.05, 0.11, 0.20, 0.21, 0.26, 0.31, 0.03 },
- { 0.04, 0.08, 0.11, 0.14, 0.16, 0.20, 0.11 },
- { 0.05, 0.07, 0.09, 0.23, 0.27, 0.32, 0.01 },
- { 0.02, 0.04, 0.05, 0.06, 0.08, 0.16, 0.03 },
- { 0.02, 0.04, 0.09, 0.13, 0.18, 0.20, 0.07 },
- { 0.01, 0.02, 0.04, 0.15, 0.17, 0.28, 0.11 },
- { 0.01, 0.11, 0.23, 0.27, 0.31, 0.32, 0.09 },
- { 0.09, 0.11, 0.23, 0.30, 0.31, 0.32, 0.06 },
- { 0.07, 0.09, 0.11, 0.17, 0.28, 0.31, 0.11 },
- { 0.16, 0.21, 0.22, 0.28, 0.31, 0.32, 0.05 },
- { 0.09, 0.23, 0.24, 0.27, 0.29, 0.32, 0.08 },
- { 0.15, 0.17, 0.18, 0.21, 0.29, 0.32, 0.13 },
- { 0.01, 0.02, 0.03, 0.06, 0.08, 0.33, 0.13 },
- { 0.01, 0.06, 0.12, 0.13, 0.22, 0.31, 0.07 },
- { 0.04, 0.07, 0.11, 0.17, 0.24, 0.33, 0.09 },
- { 0.04, 0.06, 0.17, 0.21, 0.23, 0.33, 0.07 },
- { 0.03, 0.12, 0.16, 0.17, 0.18, 0.27, 0.08 },
- { 0.12, 0.15, 0.21, 0.26, 0.32, 0.33, 0.07 },
- { 0.04, 0.17, 0.19, 0.23, 0.24, 0.27, 0.10 },
- { 0.04, 0.15, 0.16, 0.24, 0.27, 0.28, 0.03 },
- { 0.07, 0.08, 0.11, 0.13, 0.21, 0.27, 0.08 },
- { 0.01, 0.05, 0.12, 0.13, 0.21, 0.22, 0.10 },
- { 0.03, 0.04, 0.05, 0.25, 0.30, 0.31, 0.04 },
- { 0.11, 0.12, 0.14, 0.20, 0.22, 0.29, 0.14 },
- { 0.12, 0.18, 0.21, 0.22, 0.27, 0.32, 0.11 },
- { 0.05, 0.07, 0.12, 0.19, 0.27, 0.31, 0.02 },
- { 0.06, 0.10, 0.13, 0.16, 0.23, 0.24, 0.15 },
- { 0.08, 0.20, 0.25, 0.30, 0.32, 0.33, 0.01 },
- { 0.02, 0.15, 0.16, 0.17, 0.19, 0.30, 0.08 },
- { 0.06, 0.11, 0.12, 0.14, 0.17, 0.22, 0.01 },
- { 0.09, 0.18, 0.25, 0.26, 0.30, 0.32, 0.11 },
- { 0.01, 0.15, 0.16, 0.25, 0.26, 0.29, 0.10 },
- { 0.03, 0.09, 0.10, 0.19, 0.28, 0.33, 0.09 },
- { 0.04, 0.06, 0.14, 0.16, 0.18, 0.29, 0.05 },
- { 0.08, 0.11, 0.13, 0.18, 0.28, 0.33, 0.10 },
- { 0.07, 0.11, 0.14, 0.19, 0.24, 0.29, 0.05 },
- { 0.03, 0.09, 0.15, 0.20, 0.27, 0.29, 0.01 },
- { 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04 } };
- }
试验数据准备完毕,开始写个主类调用一下!看看是不是就发了,哈哈:
- package ghost.writer.start;
- import ghost.writer.data.Data;
- import ghost.writer.logic.BPFactory;
- public class TestBP {
- /**
- * @param args
- */
- public static void main(String[] args) {
- // TODO Auto-generated method stub
- // 7,18,7
- BPFactory.initialization(7, 55, 7);
- double[][] trainData = Data.trainData_89feibo;
- double[][] target = Data.target_89feibo;
- for (int i = 0; i < 1156; i++) {
- for (int j = 0; j < trainData.length; j++) {
- BPFactory.train(trainData[j], target[j]);
- }
- System.out.println(i);
- }
- // 0.04, 0.21, 0.23, 0.31, 0.32, 0.33, 0.04
- // 0.03,0.09,0.15,0.20,0.27,0.29,0.01, 0.04, 0.21, 0.23, 0.31, 0.32,
- // 0.33, 0.04
- // 0.06, 0.10, 0.11, 0.28, 0.30, 0.33, 0.12
- double[] test = { 0.02, 0.11, 0.19, 0.30, 0.32, 0.33, 0.09 };
- double[] resault = BPFactory.test(test);
- System.out.print("{");
- for (double res : resault) {
- System.out.print(String.format("%.2f", res) + ",");
- }
- System.out.println("}");
- //
- // System.out.print("{");
- // int i = 1;
- // for (double res : resault) {
- // if (res > 0.5 && res < 1.5) {
- // System.out.print(i % 33 + ",");
- // }
- // i++;
- // }
- // System.out.println("}");
- }
- }
运行此类,效果明显啊!1156次样本训练之后,test里的号码,如果是训练数据中出现过的,其test结果直接就是其后一期中出号码!这就是要发财的节奏啊!
不过!各位也看到了,本博还在写博客,还在因为工作原因研究hadoop!本博对BP神经网络进行一番研究之后,发现隐层节点基本上将训练样本数据完全“记忆”了下来,因此在用训练样本数据做测试时,可以基本达到100%出现后一期号码。而当出现训练样本最后一条记录作为输入时,问题发生了。BP网络其实本身计算出了整个样本的各各输出的“平均”数,之后他将此数输出了出来。之所以发现这个规律,是连续2次跟进买号之后,发现每次输出的结果基本都一样。因为新产生的一注号码,毕竟对往届历史的N个号码影响很小。因此BP网络输出的看上去一直是一个均值。
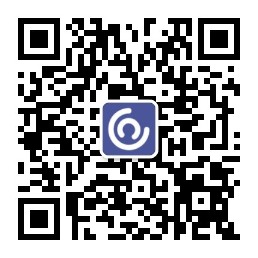
游戏做完了,BP网络也熟悉了。随机事件规律发现的问题,还需要进一步的去探索,而创造一个人工智能来帮助人们发现未知的规律,那才是真真有趣的!~~最后希望结交更多的人工智能实现方面的人,一起找乐子。用彩票数据来训练,纯属一种玩乐的心态,学习是一件有趣的事,不要太当真。